


How to Efficiently Retrieve Non-Null Values from a MySQL Table Using SELECT?
Efficiently Selecting Non-Null Values in MySQL
MySQL database users often need to retrieve only non-null values. While PHP loops can achieve this, MySQL's SELECT
statement offers more efficient, streamlined methods.
Using IS NOT NULL
:
The simplest approach is employing the IS NOT NULL
condition within the WHERE
clause:
SELECT * FROM your_table WHERE YourColumn IS NOT NULL;
This query returns all rows where YourColumn
doesn't contain a NULL
value.
Negating the Null-Safe Equality Operator (MySQL Specific):
MySQL allows using the NOT
operator to negate the null-safe equality operator (<=>
). This operator returns UNKNOWN
if either operand is NULL
. Note that this is a MySQL-specific approach and isn't standard SQL:
SELECT * FROM your_table WHERE NOT (YourColumn <=> NULL);
Leveraging the CASE
Statement:
For tables with multiple columns, and you only require non-null values from specific columns, a CASE
statement provides a solution:
SELECT CASE idx WHEN 1 THEN val1 WHEN 2 THEN val2 END AS val FROM your_table JOIN (SELECT 1 AS idx UNION ALL SELECT 2) t HAVING val IS NOT NULL;
This query uses a JOIN
to create a sequence of indices corresponding to your columns. The CASE
statement selects values only if they are not null.
These techniques allow for direct retrieval of non-null data within MySQL, eliminating the need for external language processing like PHP.
The above is the detailed content of How to Efficiently Retrieve Non-Null Values from a MySQL Table Using SELECT?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


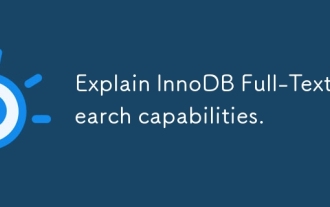
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
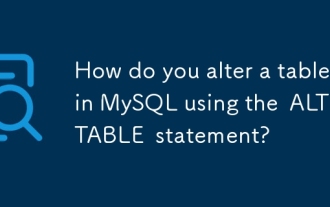
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
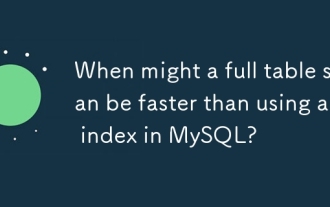
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
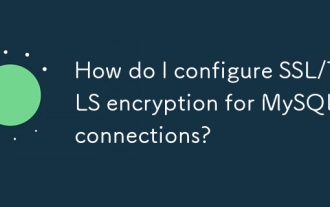
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
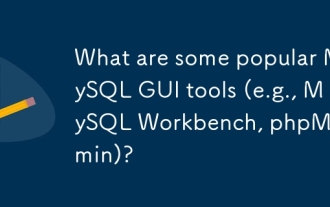
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
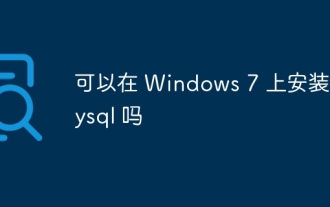
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
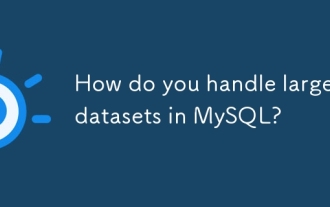
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
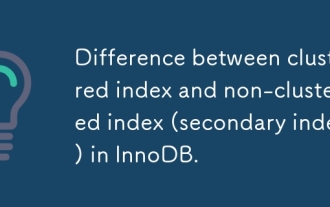
The difference between clustered index and non-clustered index is: 1. Clustered index stores data rows in the index structure, which is suitable for querying by primary key and range. 2. The non-clustered index stores index key values and pointers to data rows, and is suitable for non-primary key column queries.
