Automating Google Meet Creation
Automating Google Meet Creation with Google Calendar API and Service Account
In this blog post, we will walk through the process of automatically creating a Google Meet link by creating a Google Calendar event using the Google Calendar API. We'll use a service account to authenticate, making it possible to create events on behalf of a user in your Google Workspace domain.
Prerequisites
Before we get started, make sure you have the following:
- A Google Cloud Project with the Google Calendar API enabled.
- A Service Account created and its JSON key file downloaded.
- Domain-Wide Delegation of Authority enabled for the Service Account.
- Access to your Google Admin Console to grant the necessary permissions.
- Basic knowledge of Node.js and API requests.
Steps to Create Google Meet Automatically
Step 1: Set Up Google Cloud Project
- Go to the Google Cloud Console.
- Create a new project or select an existing one.
- Enable the Google Calendar API:
- In the sidebar, search for Calendar API and enable it for your project.
- Create a Service Account:
- In the IAM & Admin section, create a new service account.
- Download the JSON key for the service account.
Step 2: Enable Domain-Wide Delegation
- Go to the Google Admin Console (admin.google.com).
- Navigate to Security → API Controls → Manage Domain-Wide Delegation.
- Add a new Client ID for the service account:
- Find the Client ID in the Google Cloud Console under your service account.
- Add the service account’s OAuth scopes, which are required for accessing Google Calendar:
- https://www.googleapis.com/auth/calendar
- Grant the service account permission to impersonate users in your domain.
Step 3: Install Required Packages
You need a few Node.js packages to interact with the Google API and handle JWT signing:
npm install google-auth-library jsonwebtoken node-fetch
Step 4: Generate JWT Token for Authentication
Next, we’ll write a Node.js script to generate a JWT (JSON Web Token) to authenticate the service account.
const fs = require('fs'); const jwt = require('jsonwebtoken'); // Path to your service account JSON file const SERVICE_ACCOUNT_KEY_FILE = '/path/to/your/service-account-key.json'; // Scopes required for the API const SCOPES = ['https://www.googleapis.com/auth/calendar']; // Full calendar access const AUDIENCE = 'https://oauth2.googleapis.com/token'; async function generateJWT() { try { // Read and parse the service account credentials const serviceAccount = JSON.parse(fs.readFileSync(SERVICE_ACCOUNT_KEY_FILE, 'utf8')); // JWT payload const jwtPayload = { iss: serviceAccount.client_email, // Issuer: service account email sub: 'user@example.com', // Subject: email of the user whose calendar to access aud: AUDIENCE, // Audience: Google token URL scope: SCOPES.join(' '), // Scopes: space-separated list of scopes iat: Math.floor(Date.now() / 1000), // Issued at: current time in seconds exp: Math.floor(Date.now() / 1000) + 3600 // Expiration: 1 hour from now }; // Sign the JWT using the service account's private key const signedJwt = jwt.sign(jwtPayload, serviceAccount.private_key, { algorithm: 'RS256' }); console.log('Generated JWT:', signedJwt); } catch (error) { console.error('Error generating JWT:', error); } } generateJWT();
Step 5: Exchange JWT for OAuth 2.0 Token
Now, use the JWT to obtain an OAuth 2.0 token from Google’s OAuth 2.0 token endpoint:
npm install google-auth-library jsonwebtoken node-fetch
Step 6: Create a Google Calendar Event with Google Meet Link
Using the access token, we can now create a Google Calendar event with a Google Meet link.
const fs = require('fs'); const jwt = require('jsonwebtoken'); // Path to your service account JSON file const SERVICE_ACCOUNT_KEY_FILE = '/path/to/your/service-account-key.json'; // Scopes required for the API const SCOPES = ['https://www.googleapis.com/auth/calendar']; // Full calendar access const AUDIENCE = 'https://oauth2.googleapis.com/token'; async function generateJWT() { try { // Read and parse the service account credentials const serviceAccount = JSON.parse(fs.readFileSync(SERVICE_ACCOUNT_KEY_FILE, 'utf8')); // JWT payload const jwtPayload = { iss: serviceAccount.client_email, // Issuer: service account email sub: 'user@example.com', // Subject: email of the user whose calendar to access aud: AUDIENCE, // Audience: Google token URL scope: SCOPES.join(' '), // Scopes: space-separated list of scopes iat: Math.floor(Date.now() / 1000), // Issued at: current time in seconds exp: Math.floor(Date.now() / 1000) + 3600 // Expiration: 1 hour from now }; // Sign the JWT using the service account's private key const signedJwt = jwt.sign(jwtPayload, serviceAccount.private_key, { algorithm: 'RS256' }); console.log('Generated JWT:', signedJwt); } catch (error) { console.error('Error generating JWT:', error); } } generateJWT();
Step 7: Run the Full Process
Combine all the parts and run the script to create the Google Meet event automatically.
const fetch = require('node-fetch'); async function getAccessToken(signedJwt) { const response = await fetch('https://oauth2.googleapis.com/token', { method: 'POST', headers: { 'Content-Type': 'application/x-www-form-urlencoded', }, body: new URLSearchParams({ 'grant_type': 'urn:ietf:params:oauth:grant-type:jwt-bearer', 'assertion': signedJwt }) }); const data = await response.json(); return data.access_token; }
Conclusion
With the above steps, you can create Google Calendar events with Google Meet links automatically, using a service account and Domain-Wide Delegation of Authority. This method is perfect for automating meetings in a Google Workspace domain.
By enabling Domain-Wide Delegation and configuring the service account to impersonate users, you can access and manage Google Calendar events programmatically, which is extremely useful for enterprise environments.
Happy coding! ✨
The above is the detailed content of Automating Google Meet Creation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










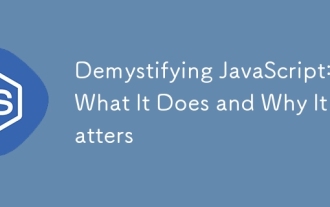
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
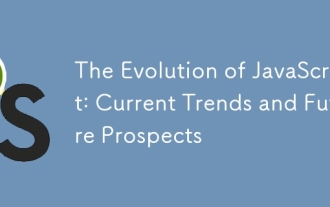
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
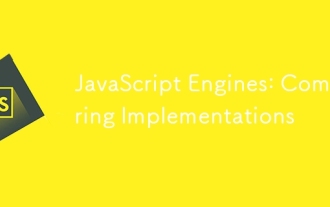
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
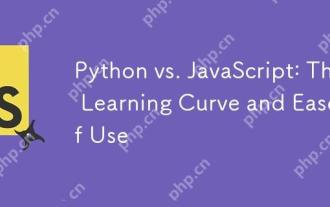
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
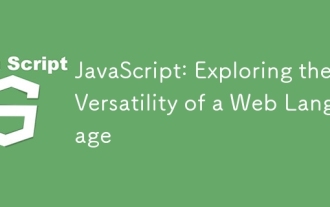
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
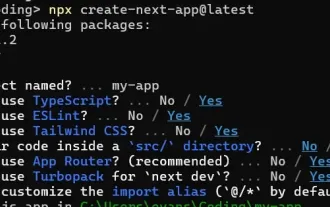
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
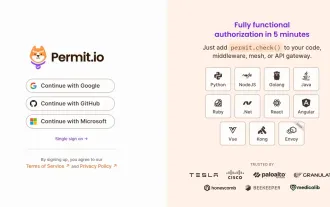
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
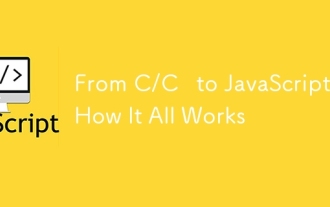
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
