


Golang - How a Chef and Waiter Teach the Single Responsibility Principle
Welcome to the first installment of my SOLID principles series focused on Golang! This series will dissect each SOLID design principle, guiding you toward creating more maintainable and scalable Go applications. We begin with the Single Responsibility Principle (SRP) – a cornerstone concept emphasizing cleaner code by ensuring each module handles a single task. ?
?️ Understanding the Single Responsibility Principle
The Single Responsibility Principle dictates:
A class, module, or function should have only one reason to change.
Essentially, each component should concentrate on a single responsibility. Multi-tasking code becomes difficult to maintain and expand without introducing errors. Adhering to SRP improves modularity, reusability, and testability.
?? A Real-World SRP Example: The Chef and the Waiter
Consider a busy restaurant. Two key roles ensure customer satisfaction:
- The Chef: Focuses solely on food preparation.
- The Waiter: Manages orders, serves food, and addresses customer needs.
Imagine one person performing both roles. The chef pausing cooking to take orders would:
- Slow service.
- Delay food preparation.
- Increase error potential.
This scenario is inefficient; one person juggling unrelated tasks leads to chaos.
Applying SRP in the Restaurant Setting
Following the Single Responsibility Principle:
- The Chef solely prepares food.
- The Waiter solely manages orders and customer service.
This separation allows each individual to excel in their specific role, resulting in a more efficient and positive dining experience. ?✨
? SRP in Software Development
Similar to the restaurant example, your code should structure classes and functions to handle only one responsibility. This enhances maintainability, accelerates changes, and minimizes errors.
SRP in Action with Golang
Let's examine how violating SRP can create brittle and unmanageable code.
❌ Example: Violating SRP
Consider a basic coffee shop order management system:
<code>package main import "fmt" // Order stores coffee order details. type Order struct { CustomerName string CoffeeType string Price float64 } // ProcessOrder handles multiple responsibilities. func (o *Order) ProcessOrder() { // Handles payment processing fmt.Printf("Processing payment of $%.2f for %s\n", o.Price, o.CustomerName) // Prints receipt fmt.Printf("Receipt:\nCustomer: %s\nCoffee: %s\nAmount: $%.2f\n", o.CustomerName, o.CoffeeType, o.Price) } func main() { order := Order{CustomerName: "John Doe", CoffeeType: "Cappuccino", Price: 4.50} order.ProcessOrder() }</code>
The Order
struct handles data storage, payment processing, and receipt printing – a clear SRP violation. Modifying any aspect impacts ProcessOrder
, hindering maintainability.
?️ Refactoring to Adhere to SRP
Let's separate responsibilities into distinct components:
<code>package main import "fmt" // Order stores coffee order details. type Order struct { CustomerName string CoffeeType string Price float64 } // ProcessOrder handles multiple responsibilities. func (o *Order) ProcessOrder() { // Handles payment processing fmt.Printf("Processing payment of $%.2f for %s\n", o.Price, o.CustomerName) // Prints receipt fmt.Printf("Receipt:\nCustomer: %s\nCoffee: %s\nAmount: $%.2f\n", o.CustomerName, o.CoffeeType, o.Price) } func main() { order := Order{CustomerName: "John Doe", CoffeeType: "Cappuccino", Price: 4.50} order.ProcessOrder() }</code>
? Advantages of SRP
-
Separation of Concerns:
Order
stores data;PaymentProcessor
handles payments;ReceiptPrinter
generates receipts. -
Enhanced Testability:
PaymentProcessor
andReceiptPrinter
can be tested independently. - Simplified Maintenance: Receipt formatting changes won't affect payment processing.
❓ When to Apply SRP
Identify violations such as:
- Functions or structs performing unrelated tasks.
- Modules mixing concerns (e.g., business logic and I/O).
✨ Conclusion
The Single Responsibility Principle simplifies code understanding, maintenance, and expansion. This is just the start! The next post in this series explores the "O" in SOLID: the Open/Closed Principle.
You can also explore my previous post on Dependency Injection, a crucial OOP technique.
Happy coding! ?
Follow me for updates on future posts:
- Github
- Twitter/X
The above is the detailed content of Golang - How a Chef and Waiter Teach the Single Responsibility Principle. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


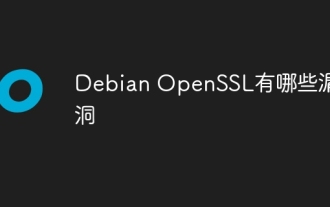
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
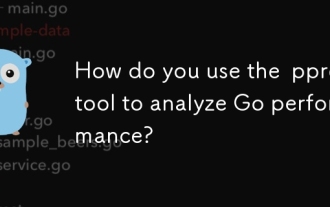
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
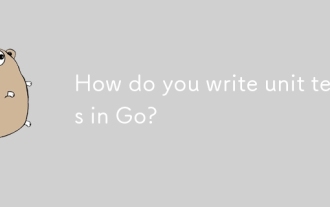
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
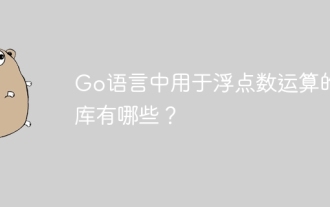
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
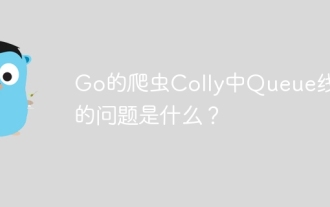
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
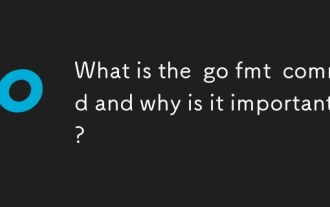
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
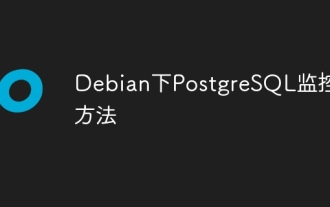
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
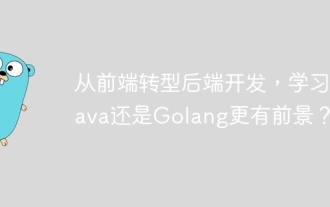
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
