Python Basics: A Beginner's Guide
Getting Started with Python: Tutorial for Beginners
Python is a powerful and easy-to-learn programming language that can be used for a variety of tasks, from creating web applications to data analysis. This guide will introduce you to the core concepts of Python and help you get started on your programming journey.
- Python installation
Download the latest version of Python from the python.org official website.
Install Python to your computer. Remember to check the "Add Python to PATH" option during the installation process.
Enter the following command in the terminal to verify whether the installation is successful:
python --version
- Variables and data types
Python supports multiple data types. Here are the main types:
int: integer (e.g., 42, -10)
float: floating point number (e.g., 3.14, -0.5)
str: string (for example, 'Hello, World!')
bool: Boolean value (True or False)
Example:
Variable example
x = 10 # integer pi = 3.14 # floating point number name = "Alice" # string is_active = True # Boolean value
print(x, pi, name, is_active)
- Input and output
Use the input() function to get user input:
name = input("Please enter your name:") print("Hello,", name)
- Conditional statement
Python uses if, elif and else statements to perform different actions based on conditions:
age = int(input("Please enter your age:")) if age < 18: print("You are still a child.") elif age < 60: print("You are an adult.") else: print("You are a retiree.")
- Loop
The for loop is used to iterate over the elements in a sequence:
for i in range(5): print("Number:", i)
The while loop executes when the condition is true:
count = 0 while count < 5: print("Counter:", count) count = 1
- Function
Functions help organize code and improve code reusability:
def greet(name): return f"Hello, {name}!"
print(greet("Alice"))
- Lists and Dictionaries
A list is an ordered collection of data:
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
A dictionary is a collection of key-value pairs:
person = {"Name": "Alice", "Age": 25} print(person["name"])
- File Operation
Read and write files in Python:
Write to file
with open("example.txt", "w") as file: file.write("Hello, world!")
Read file
with open("example.txt", "r") as file: content = file.read() print(content)
- Python library
Python has a large number of libraries for various tasks. Here are some commonly used libraries:
math — used for mathematical operations.
random — used to generate random numbers.
pandas — for data analysis.
matplotlib — for data visualization.
Math library usage example:
import math print(math.sqrt(16)) # Output: 4.0
- Advice for beginners
Practice every day.
Troubleshoot on sites like Codewars or LeetCode.
Read documentation and books like Learn Python by Mark Lutz.
Participate in the developer community.
Join the Developer Telegram channel.
Congratulations! You now have the basics to start learning Python. Happy coding!
The above is the detailed content of Python Basics: A Beginner's Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










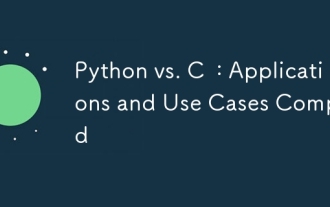
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
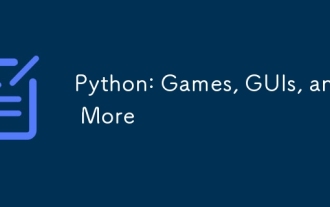
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
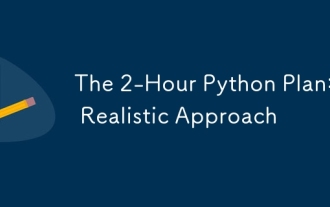
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
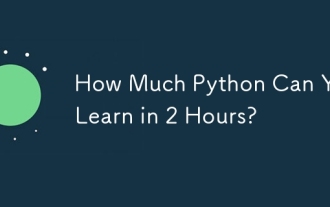
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
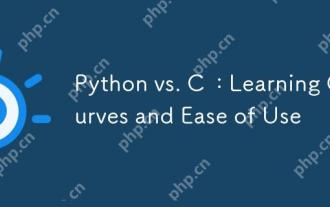
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
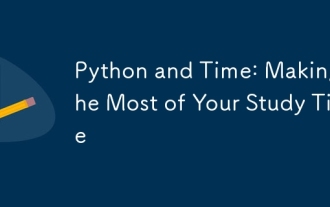
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
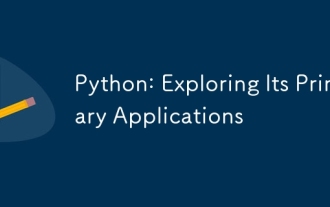
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
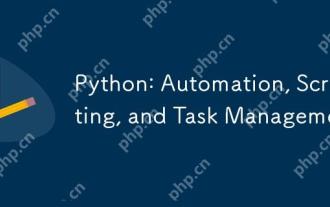
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
