Key derivation function with Python
Welcome to the next pikoTutorial!
In the previous article, we learned how to use Python for symmetric data encryption. The final example is about converting a user-supplied password directly into an encryption key. While it works, it's not a recommended practice. Today you will learn the recommended method, the key derivation function.
Key derivation function
Below you can find an extended example of how to use the PBKDF2HMAC key derivation function in Python:
# 导入Base64编码的实用程序 import base64 # 导入Fernet from cryptography.fernet import Fernet from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC from cryptography.hazmat.primitives import hashes # 导入getpass用于安全输入读取 from getpass import getpass # 读取纯文本密码 plain_text_password: str = getpass(prompt='密码:') # 将密码转换为字节 password_bytes = plain_text_password.encode('utf-8') # 演示的一些salt值,在实践中使用安全的随机值 salt = b'\x00' * 16 # 使用PBKDF2HMAC从密码派生安全密钥 kdf = PBKDF2HMAC( algorithm=hashes.SHA256(), length=32, salt=salt, iterations=100000 ) # 使用Base64编码派生的密钥 key = base64.urlsafe_b64encode(kdf.derive(password_bytes)) # 使用派生的密钥创建一个Fernet实例 fernet = Fernet(key) # 要加密的数据 data = b'一些秘密数据' # 加密数据 encrypted_data = fernet.encrypt(data) # 解密数据 decrypted_data = fernet.decrypt(encrypted_data) # 打印解密后的数据 print(f"解密文本:{decrypted_data.decode()}")
Not only are keys created this way more secure, but they no longer require plain text passwords to be exactly 32 bytes long.
Note for beginners: Remember that salt is necessary to decrypt data!
The above is the detailed content of Key derivation function with Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




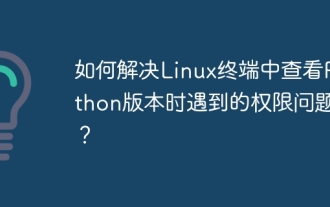
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
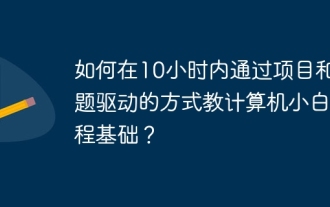
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
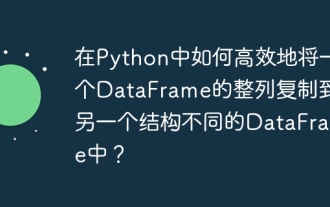
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
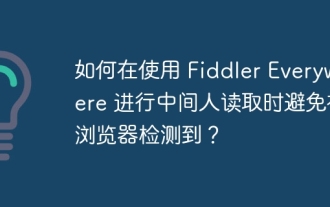
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
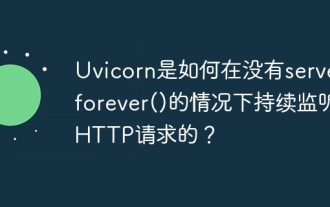
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
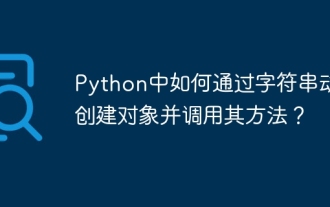
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
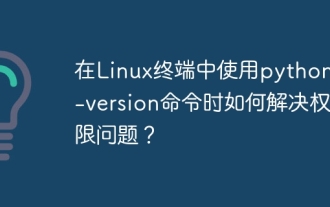
Using python in Linux terminal...
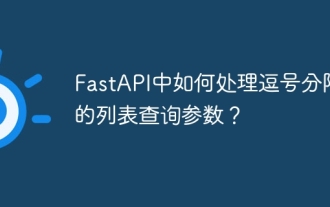
Fastapi ...
