


Laravel Eloquent ORM in Bangla Part-Inserting and Updating Models)
Laravel Eloquent ORM can be used to add new data and update existing data in the database. It works in a simple and natural manner. Let's discuss in detail.
Adding Data (Inserting Models)
1. Create new record using save()
method
The save()
method is used to create and save Eloquent models.
use App\Models\Post; // নতুন পোস্ট তৈরি $post = new Post(); $post->title = 'নতুন ব্লগ পোস্ট'; $post->content = 'এটি পোস্টের বিষয়বস্তু।'; $post->status = 'draft'; // ডেটা সংরক্ষণ $post->save();
save()
method creates a new record in the database.
2. create()
method using the
shorthand method
The create()
method inserts data into one line.
use App\Models\Post; Post::create([ 'title' => 'দ্রুত ব্লগ পোস্ট', 'content' => 'এটি পোস্টের বিষয়বস্তু।', 'status' => 'published', ]);
Remember: To use create()
you must define the fillable
or guarded
property in your model.
class Post extends Model { protected $fillable = ['title', 'content', 'status']; }
3. Adding multiple records (Mass Insert)
Multiple records can be inserted at once using theinsert()
method.
use App\Models\Post; Post::insert([ ['title' => 'পোস্ট ১', 'content' => 'বিষয়বস্তু ১', 'status' => 'published'], ['title' => 'পোস্ট ২', 'content' => 'বিষয়বস্তু ২', 'status' => 'draft'], ]);
Updating Data (Updating Models)
1. Updating specific records using the save()
method
A model's data can be updated by fetching it from the database.
use App\Models\Post; // রেকর্ড খুঁজে বের করা $post = Post::find(1); // ডেটা আপডেট করা $post->title = 'আপডেট করা ব্লগ পোস্ট'; $post->status = 'published'; // সংরক্ষণ $post->save();
2. update()
is updated using the
method
The update()
method is used to update multiple columns simultaneously.
use App\Models\Post; Post::where('id', 1)->update([ 'title' => 'আপডেট করা শিরোনাম', 'status' => 'published', ]);
3. Updating multiple records at once
Multiple records can be updated usingupdate()
.
use App\Models\Post; // নতুন পোস্ট তৈরি $post = new Post(); $post->title = 'নতুন ব্লগ পোস্ট'; $post->content = 'এটি পোস্টের বিষয়বস্তু।'; $post->status = 'draft'; // ডেটা সংরক্ষণ $post->save();
upsert()
method
upsert()
method is used to add new data or update existing data.
use App\Models\Post; Post::create([ 'title' => 'দ্রুত ব্লগ পোস্ট', 'content' => 'এটি পোস্টের বিষয়বস্তু।', 'status' => 'published', ]);
The above is the detailed content of Laravel Eloquent ORM in Bangla Part-Inserting and Updating Models). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










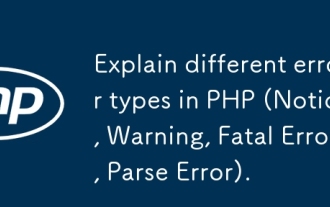
There are four main error types in PHP: 1.Notice: the slightest, will not interrupt the program, such as accessing undefined variables; 2. Warning: serious than Notice, will not terminate the program, such as containing no files; 3. FatalError: the most serious, will terminate the program, such as calling no function; 4. ParseError: syntax error, will prevent the program from being executed, such as forgetting to add the end tag.
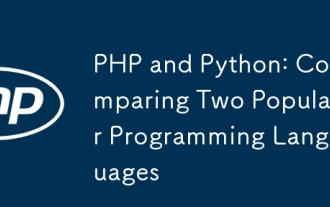
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
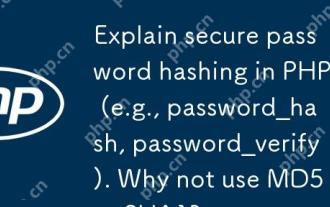
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
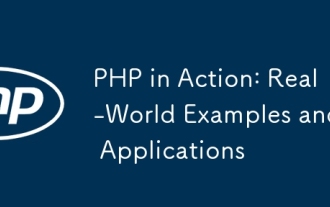
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
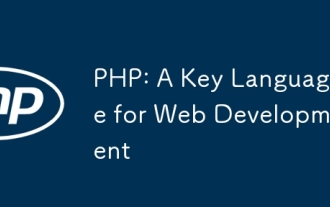
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
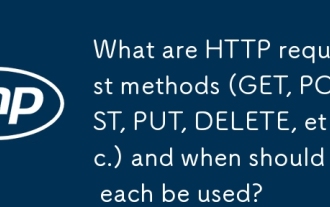
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
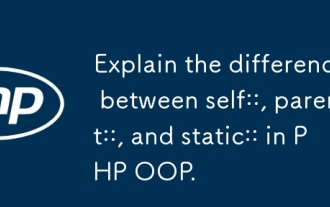
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
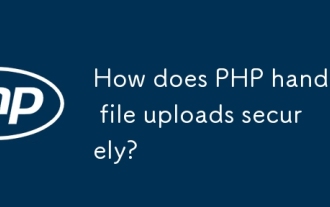
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
