How to set environment variables in Node.js
Modern software demands flexibility, scalability, and robust security. Environment variables play a vital role in achieving this balance. This guide explores various methods for setting and managing environment variables within Node.js applications, emphasizing best practices for security and maintainability. We'll cover everything from startup validation to preventing sensitive data exposure. Whether you're a seasoned developer or new to Node.js, this information is essential for building secure, adaptable applications.
What are Environment Variables?
Environment variables are key-value pairs stored outside your application's codebase, typically in configuration files or system settings. They hold sensitive data like API keys and database credentials, preventing hardcoding and improving security. This approach simplifies management across different environments (development, testing, production).
In Node.js, environment variables enable dynamic application configuration without code modification. The same codebase can interact with different databases or APIs depending on the environment, enhancing security, simplifying deployment, and boosting adaptability.
Unlike standard JavaScript variables, environment variables are not defined within the code. They're accessed via process.env
and exist independently, potentially influencing multiple applications on the system.
Accessing Environment Variables in Node.js
Node.js uses the process.env
object to access and manage environment variables. To retrieve a variable's value, use process.env.VARIABLE_NAME
. For example, process.env.API_KEY
retrieves the value associated with API_KEY
. While technically possible to set environment variables within code, this is generally discouraged; it negates the benefits of using environment variables in the first place.
Here's how API_KEY
might be used in an Express API:
const express = require('express'); const app = express(); // Access API key from environment variables const apiKey = process.env.API_KEY; if (!apiKey) { console.error('Error: API key is not defined.'); process.exit(1); } app.get('/', (req, res) => { res.send('API key successfully loaded.'); }); // Start the server const PORT = process.env.PORT || 3000; app.listen(PORT, () => { console.log(`Server running on port ${PORT}`); });
Setting Environment Variables in Node.js
Now, let's explore different methods for setting environment variables:
- Using
dotenv
: Thedotenv
package simplifies managing environment variables by separating them from your code. Define key-value pairs in a.env
file:
<code>PORT=3000 DB_USERNAME=dbuser</code>
Import and use it like this:
import * as dotenv from 'dotenv'; dotenv.config(); console.log(process.env.PORT); // Output: 3000 console.log(process.env.DB_USERNAME); // Output: dbuser
You can specify alternative .env
file paths using dotenv.config({ path: './path/to/another.env' })
. While useful for development, consider other methods for production.
- System-Level Setting: On Unix-like systems (Linux, macOS), add variables to your shell configuration file (e.g.,
~/.bashrc
,~/.zshrc
). This affects all processes in that shell session. For example:
const express = require('express'); const app = express(); // Access API key from environment variables const apiKey = process.env.API_KEY; if (!apiKey) { console.error('Error: API key is not defined.'); process.exit(1); } app.get('/', (req, res) => { res.send('API key successfully loaded.'); }); // Start the server const PORT = process.env.PORT || 3000; app.listen(PORT, () => { console.log(`Server running on port ${PORT}`); });
Restart your terminal or run source ~/.bashrc
to apply changes. For system-wide access (system processes), use /etc/environment
.
- Launch Script: Create a script that sets variables and then runs your Node.js application (e.g.,
launch.sh
):
<code>PORT=3000 DB_USERNAME=dbuser</code>
Make it executable (chmod x launch.sh
) and run it (./launch.sh
).
- PM2 (Process Manager 2): PM2 allows setting environment variables during application startup:
import * as dotenv from 'dotenv'; dotenv.config(); console.log(process.env.PORT); // Output: 3000 console.log(process.env.DB_USERNAME); // Output: dbuser
Or use an ecosystem.config.js
file for environment-specific configurations.
- Docker: In Docker, set variables in the
Dockerfile
usingENV
:
# ~/.bashrc export PORT=3000 export DB_USERNAME=myuser
Override defaults when running the container using -e PORT=5173
or within a docker-compose.yml
file.
Best Practices for Using Environment Variables
Follow these best practices for secure and maintainable applications:
-
Descriptive Names and Documentation: Use clear, descriptive names and document their purpose in your project's README.
-
Startup Validation: Validate environment variables at application startup to ensure they're set correctly. Handle missing variables gracefully (default values or error handling).
-
.env
File Exclusion: Exclude.env
files from version control (Git) using.gitignore
. -
Consider a KMS (Key Management System): For enhanced security, especially with highly sensitive data, use a KMS to encrypt and store your environment variables.
-
Default Values: Provide default values for non-critical environment variables to ensure application functionality even if variables are missing.
-
Never Expose in the Frontend: Never expose sensitive environment variables directly to the client-side code.
Clerk's Use of Environment Variables
Clerk SDKs utilize environment variables for configuration and application association within the Clerk dashboard. This enables secure backend requests and frontend validation using Express. For example:
#!/bin/bash export PORT=3000 export DB_USERNAME=myuser node app.js
Conclusion
Securely managing environment variables is paramount. By following these best practices, you'll significantly enhance the security and maintainability of your Node.js applications, ensuring they're ready for production deployment.
The above is the detailed content of How to set environment variables in Node.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


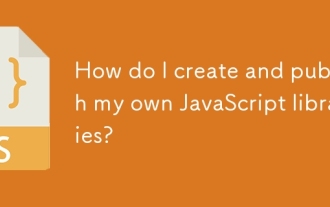
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
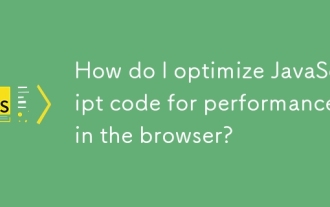
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
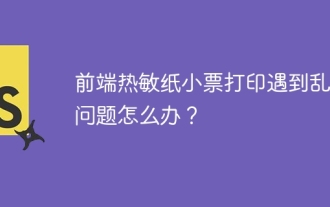
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
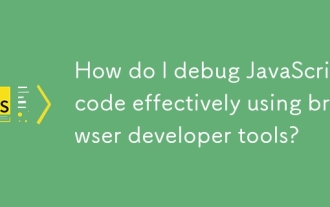
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
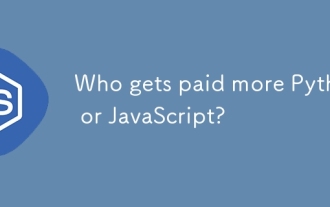
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
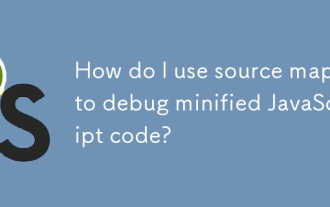
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
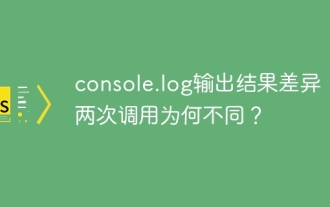
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
