


How Can I Implement Best Practices for Exception Handling in My Software?
Robust Exception Handling: Best Practices for Software Development
Effective exception handling is paramount for building stable and user-friendly software. This guide outlines key best practices to optimize your approach.
Never Ignore Exceptions (Empty Catch Blocks)
Empty catch
blocks are unhelpful. Always either handle the exception appropriately or allow it to propagate for higher-level handling.
Leverage Custom Exceptions
For predictable exceptions, create custom exception classes. This provides more informative error messages and preserves crucial exception details, enabling precise handling and preventing cascading failures.
Implement Global Exception Handling
Use a top-level error handler (e.g., hooking into Application.ThreadException
) to capture all unhandled exceptions. The response should be context-dependent: logging, user-friendly error messages, or other appropriate actions for UI or service applications.
Targeted Try-Catch Blocks
Wrap potentially problematic code sections (e.g., external component interactions, file I/O, complex calculations) in try-catch
blocks to prevent unexpected crashes.
Comprehensive Exception Logging and Reporting
Never disregard exceptions. Implement logging to record details like the error message, stack trace, and timestamp. This facilitates thorough error analysis and future improvements.
Informative User Error Messages
Notify users of critical exceptions that impact their experience. Craft clear, helpful error messages that provide context and allow users to take corrective action or continue their workflow.
Meaningful Exception Re-throwing
Avoid simply re-throwing exceptions without added context. When catching an exception, either handle it directly or enrich it with extra information before propagating it further.
Illustrative Code Example
try { // Perform operation } catch (Exception ex) when (ex is FileNotFoundException || ex is IOException) { // Handle file I/O errors } catch (Exception ex) { // Handle all other exceptions LogException(ex); }
By following these best practices, you'll create more reliable and user-friendly applications, minimizing disruptions caused by exceptions.
The above is the detailed content of How Can I Implement Best Practices for Exception Handling in My Software?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


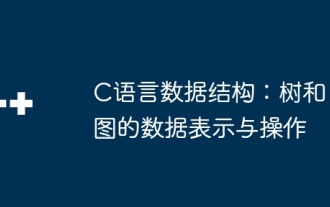
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
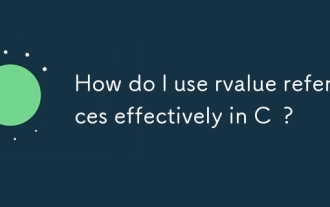
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
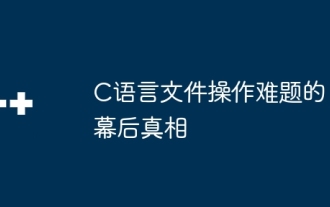
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
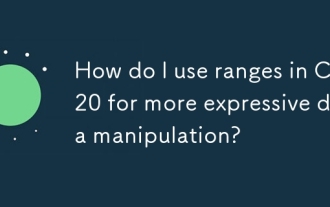
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
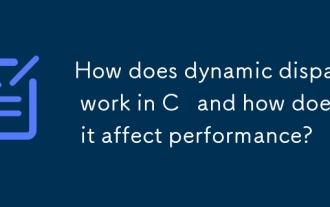
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
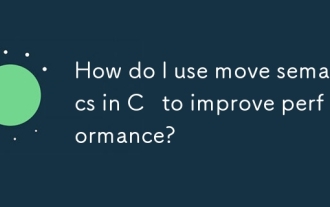
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
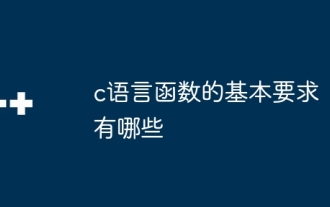
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
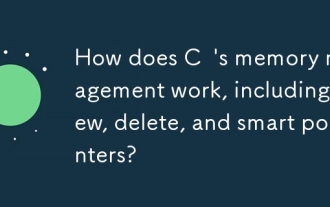
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
