Item Do not depend on the thread scheduler
1. The Role of the Thread Scheduler
The thread scheduler determines which threads can run and for how long. Scaling policies vary between operating systems. It is crucial not to depend on the scheduler's behavior to guarantee the correctness or performance of your program, as this compromises portability.
2. Strategies for Robust Programs: Thread Balancing
To create robust programs, keep a number of executable threads close to the number of available processors. This avoids overloading the scheduler and ensures consistent behavior. Although the total number of threads may be higher, waiting (non-runnable) threads do not significantly impact system load.
3. Techniques for Managing Threads: Avoiding Active Waiting and Scaling
Avoid hot waiting, where a thread constantly checks the state of a shared resource. This consumes processor resources unnecessarily. Decrease the number of executable threads by correctly sizing thread pools (as in the Executor Framework) and creating appropriately sized tasks – not so small that overhead prevails, not so large that parallelism is prevented.
4. Example of Bad Practice: Active Hope
The code below demonstrates active waiting:
public class SlowCountDownLatch { private int count; public SlowCountDownLatch(int count) { this.count = count; } public void await() { while (count > 0) { // Espera-ativa: desperdício de recursos do processador } } public void countDown() { if (count > 0) { count--; } } }
This code consumes excessive resources. The solution is to use CountDownLatch
, which uses efficient blocking mechanisms.
5. Avoiding Thread.yield()
The Thread.yield()
method is inconsistent between different JVM implementations and does not provide a robust or portable solution to concurrency problems. Instead of using Thread.yield()
, restructure the code to reduce the number of executable threads.
Incorrect example:
while (!condition) { Thread.yield(); // Incorreto: uso de Thread.yield() }
6. Thread Priority Adjustment
Adjusting thread priorities is also not very portable, varying between operating systems and JVMs. Its use must be restricted to specific scenarios where the improvement in quality of service justifies the loss of portability, and never as a solution to structural problems.
7. Conclusions
Do not depend on the scheduler to correct or optimize your program's performance. Avoid the use of Thread.yield()
and excessive priority adjustments. The best approach is to restructure your applications to maintain a balanced number of executable threads.
Example from the book:
The above is the detailed content of Item Do not depend on the thread scheduler. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
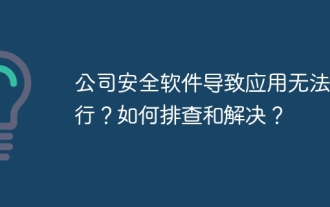
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
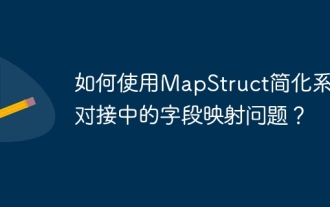
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
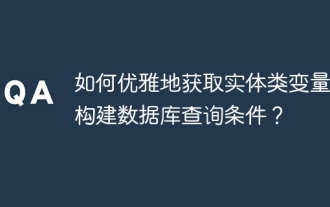
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
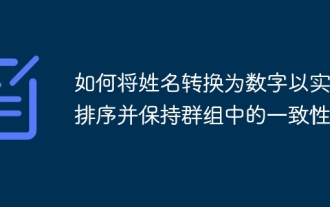
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
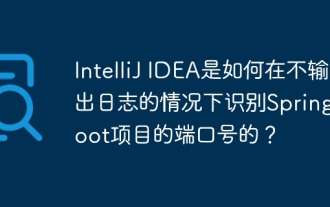
Start Spring using IntelliJIDEAUltimate version...
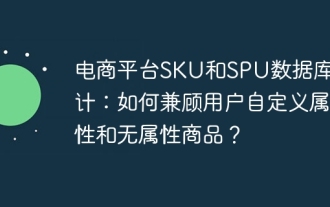
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
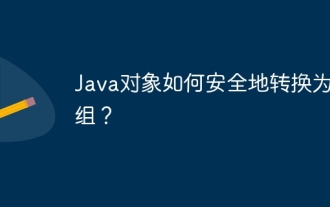
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
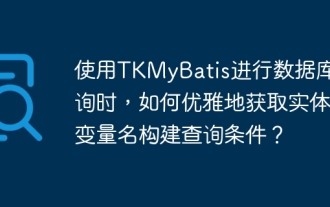
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
