How to Convert a Byte Array to a String in C#?
C# Byte Array to String Conversion: A Comprehensive Guide
This guide provides a detailed explanation of converting a byte array to a string in C#. This is a common task, particularly when dealing with data serialization or network communication.
You've already created a byte array using BinaryWriter
, containing two strings. To reconstruct these strings, you need to specify the encoding used during the initial writing process.
The simplest approach uses the system's default encoding:
var str = System.Text.Encoding.Default.GetString(result);
This method converts result
(your byte array) to a string using your system's default character encoding. However, for better reliability and cross-platform compatibility, explicitly specifying the encoding is highly recommended.
To use a specific encoding (e.g., UTF-8, UTF-16, ASCII), use this code:
var str = System.Text.Encoding.GetEncoding("YourEncoding").GetString(result);
Remember to replace "YourEncoding"
with the correct encoding name. Using UTF-8 is generally preferred for its broad support and ability to handle a wide range of characters. For example:
var str = System.Text.Encoding.UTF8.GetString(result);
Choosing the correct encoding is crucial. Using an incorrect encoding will lead to data corruption or garbled text. Ensure you use the same encoding for both writing the byte array and reading it back into a string.
The above is the detailed content of How to Convert a Byte Array to a String in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


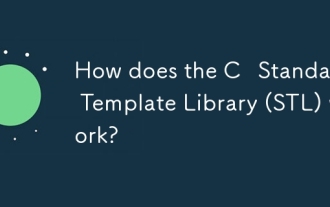
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
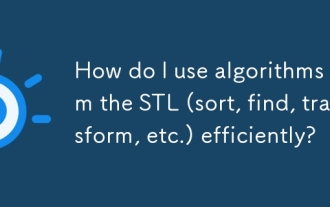
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
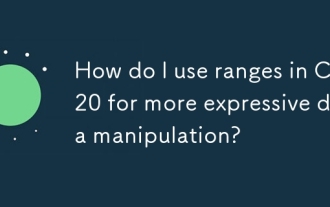
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
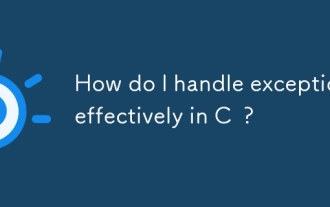
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
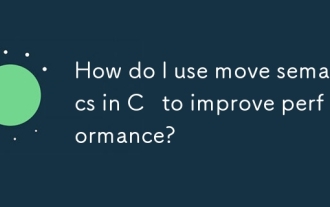
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
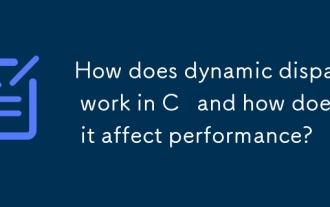
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
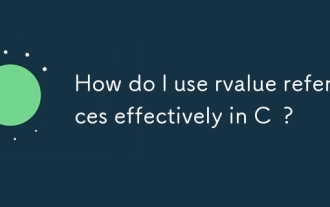
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
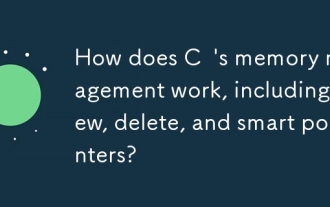
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
