Why this in JavaScript Differs from Other OOP Languages
JavaScript's this
keyword often causes confusion, especially for developers coming from languages like C#, Java, or Python where self
consistently refers to the current object instance. Unlike those languages, JavaScript's this
is dynamic, its value determined by the function's invocation context. This guide summarizes the various scenarios impacting this
's behavior.
1. Global Scope:
-
Non-Strict Mode:
this
points to the global object (window
in browsers,global
in Node.js).
console.log(this); // window or global
- Strict Mode:
this
isundefined
.
"use strict"; console.log(this); // undefined
2. Inside Functions:
- Regular Functions: In non-strict mode,
this
refers to the global object; in strict mode, it'sundefined
.
function myFunc() { console.log(this); } myFunc(); // window (non-strict), undefined (strict)
3. Object Methods:
- When a function is called as an object method,
this
refers to that object.
const myObj = { name: "JavaScript", greet() { console.log(this.name); // this refers to myObj } }; myObj.greet(); // Output: JavaScript
4. Arrow Functions:
- Arrow functions lack their own
this
. They inheritthis
from their lexical scope (surrounding context).
const myObj = { name: "JavaScript", arrowFunc: () => { console.log(this.name); // Inherits this from the global scope } }; myObj.arrowFunc(); // undefined (in browsers, this is window)
5. Constructors:
- Within a constructor function or class,
this
refers to the newly created instance.
class Person { constructor(name) { this.name = name; } greet() { console.log(`Hello, ${this.name}`); } } const person = new Person("Alice"); person.greet(); // Output: Hello, Alice
6. Explicit Binding (call
, apply
, bind
):
JavaScript functions are objects with methods (call
, apply
, bind
) for explicitly setting this
.
call
andapply
invoke the function with a specifiedthis
value.call
uses comma-separated arguments;apply
takes an array.
function greet(greeting) { console.log(`${greeting}, ${this.name}`); } const user = { name: "Alice" }; greet.call(user, "Hello"); // Output: Hello, Alice greet.apply(user, ["Hi"]); // Output: Hi, Alice
bind
returns a new function withthis
permanently bound.
const boundGreet = greet.bind(user); boundGreet("Hello"); // Output: Hello, Alice
7. Event Listeners:
- Regular Functions:
this
refers to the element triggering the event.
const btn = document.querySelector("button"); btn.addEventListener("click", function() { console.log(this); // The button element });
- Arrow Functions:
this
inherits from the surrounding scope, not the element.
btn.addEventListener("click", () => { console.log(this); // this depends on the arrow function's definition context });
8. setTimeout
/ setInterval
:
- Regular Functions:
this
defaults to the global object.
setTimeout(function() { console.log(this); // window in browsers }, 1000);
- Arrow Functions:
this
is inherited lexically.
setTimeout(() => { console.log(this); // Inherits this from surrounding context }, 1000);
9. Classes:
- Inside a class method,
this
refers to the class instance.
console.log(this); // window or global
10. Context Loss (Method Extraction):
Assigning a method to a variable or passing it as a callback can cause this
binding loss.
"use strict"; console.log(this); // undefined
Solutions: Use .bind(obj)
or an arrow function to maintain context.
11. new
Keyword:
Using new
with a function creates a new object, and this
refers to that object.
function myFunc() { console.log(this); } myFunc(); // window (non-strict), undefined (strict)
Summary Table:
Context |
|
||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Global (non-strict) | Global object (window/global) | ||||||||||||||||||||
Global (strict) | undefined |
||||||||||||||||||||
Object Method | The object owning the method | ||||||||||||||||||||
Arrow Function | Lexical scope (surrounding context) | ||||||||||||||||||||
Constructor/Class | The instance being created | ||||||||||||||||||||
call , apply , bind
|
Explicitly defined value | ||||||||||||||||||||
Event Listener | The element triggering the event | ||||||||||||||||||||
setTimeout /setInterval
|
Global object (regular function), lexical scope (arrow function) | ||||||||||||||||||||
Keyword |
The newly created object |
this
Understanding these scenarios is crucial for writing correct and predictable JavaScript code. Remember to utilize techniques like explicit binding when necessary to avoid unexpected behavior.The above is the detailed content of Why this in JavaScript Differs from Other OOP Languages. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
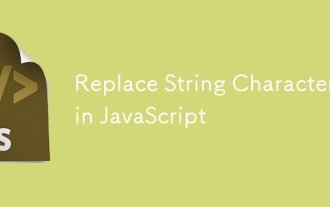
Replace String Characters in JavaScript
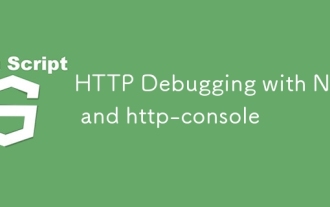
HTTP Debugging with Node and http-console
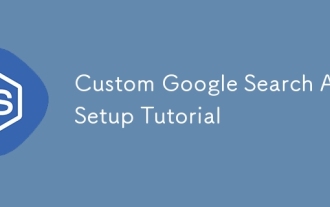
Custom Google Search API Setup Tutorial
