


How to Prevent Multiple Instances of a .NET Application from Running Simultaneously?
Preventing Multiple Application Instances from Running Simultaneously in .NET: A Comprehensive Guide
In .NET development, you may encounter situations where you need to restrict multiple application instances from running at the same time. Whether it's for resource optimization or functionality reasons, it's crucial to understand how to achieve this.
Mutex lock: a reliable solution
One of the most common ways to control application instances is the Mutex class. A mutex lock (or "mutex") allows only one process instance to access a shared resource at any given time. By creating a mutex with a unique identifier (for example, a GUID), you ensure that only one application instance can run under that identifier.
Example implementation
The following C# code demonstrates how to use a mutex to prevent multiple instances:
[STAThread] static void Main() { using (Mutex mutex = new Mutex(false, "Global\" + appGuid)) { if (!mutex.WaitOne(0, false)) { MessageBox.Show("应用程序实例已在运行"); return; } Application.Run(new Form1()); } } private static string appGuid = "c0a76b5a-12ab-45c5-b9d9-d693faa6e7b9";
This code creates a mutex using a unique GUID as the identifier. If a previous instance of the application is running, the WaitOne method returns False and the user is alerted. Otherwise, a new instance will be started.
Notes
While Mutex provides a reliable solution, there are a few things to note:
- Shared resources: Ensure that all processes accessing shared resources (e.g. files, databases) use the same mutex identifier to prevent data corruption.
- Potential issues: If the mutex is never released (for example, due to an unhandled exception), this may prevent the application from running again.
- Performance overhead: Creating and managing mutex locks may incur some performance overhead, so use them with caution.
Alternative methods
In some cases, using Mutex may be too complex or impractical. Other methods to consider include:
- Named Pipes: Communication between instances via named pipes, allowing sharing of resources and coordination without blocking multiple instances.
- Remote Procedure Call (RPC): Similar to named pipes, RPC facilitates communication between distributed instances, reducing the need for multiple executions.
Conclusion
Preventing multiple application instances in .NET requires careful consideration of use cases and potential limitations. Mutex provides a reliable solution for enforcing exclusivity, but one must understand its caveats and explore alternatives as needed. By adhering to these guidelines, developers can ensure that their applications run as expected without conflicts or resource contention.
The above is the detailed content of How to Prevent Multiple Instances of a .NET Application from Running Simultaneously?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




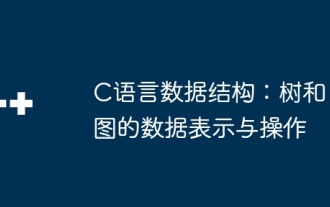
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
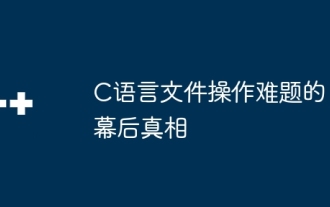
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
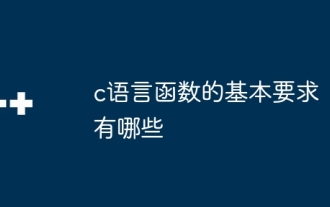
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
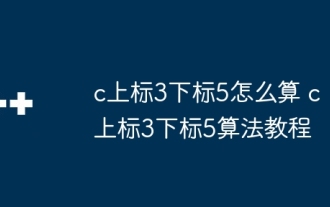
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
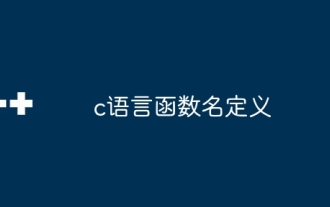
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
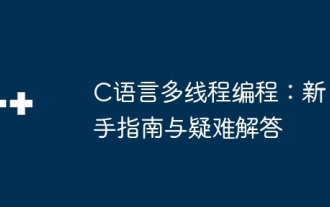
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
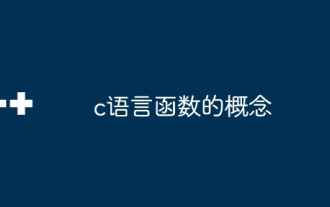
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
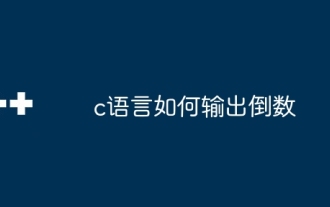
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
