


Scalable Python backend: Building a containerized FastAPI Application with uv, Docker, and pre-commit: a step-by-step guide
In today's containerized world, efficient backend application deployment is crucial. FastAPI, a popular Python framework, excels at creating fast, high-performance APIs. We'll use uv
, a package manager, to streamline dependency management.
uv
Assuming you've installed uv
and Docker, let's create our app: uv init simple-app
. This generates:
<code>simple-app/ ├── .python-version ├── README.md ├── hello.py └── pyproject.toml</code>
pyproject.toml
holds project metadata:
[project] name = "simple-app" version = "0.1.0" description = "Add your description here" readme = "README.md" requires-python = ">=3.11" dependencies = []
Add project dependencies to pyproject.toml
:
dependencies = [ "fastapi[standard]=0.114.2", "python-multipart=0.0.7", "email-validator=2.1.0", "pydantic>2.0", "SQLAlchemy>2.0", "alembic=1.12.1", ] [tool.uv] dev-dependencies = [ "pytest=7.4.3", "mypy=1.8.0", "ruff=0.2.2", "pre-commit=4.0.0", ]
The [tool.uv]
section defines development dependencies excluded during deployment. Run uv sync
to:
- Create
uv.lock
. - Create a virtual environment (
.venv
).uv
downloads a Python interpreter if needed. - Install dependencies.
FastAPI
Create the FastAPI application structure:
<code>recipe-app/ ├── app/ │ ├── main.py │ ├── __init__.py │ └── ... ├── .python-version ├── README.md └── pyproject.toml</code>
In app/main.py
:
from fastapi import FastAPI from pydantic import BaseModel app = FastAPI() class Hello(BaseModel): message: str @app.get("/", response_model=Hello) async def hello() -> Hello: return Hello(message="Hi, I am using FastAPI")
Run with: uv run fastapi dev app/main.py
. You'll see output similar to:
Access it at https://www.php.cn/link/c099034308f2a231c24281de338726c1.
Docker
Let's Dockerize. We'll develop within containers. Add a Dockerfile
:
FROM python:3.11-slim ENV PYTHONUNBUFFERED=1 COPY --from=ghcr.io/astral-sh/uv:0.5.11 /uv /uvx /bin/ ENV UV_COMPILE_BYTE=1 ENV UV_LINK_MODE=copy WORKDIR /app ENV PATH="/app/.venv/bin:$PATH" COPY ./pyproject.toml ./uv.lock ./.python-version /app/ RUN --mount=type=cache,target=/root/.cache/uv \ --mount=type=bind,source=uv.lock,target=uv.lock \ --mount=type=bind,source=pyproject.toml,target=pyproject.toml \ uv sync --frozen --no-install-project --no-dev COPY ./app /app/app RUN --mount=type=cache,target=/root/.cache/uv \ uv sync --frozen --no-dev CMD ["fastapi", "dev", "app/main.py", "--host", "0.0.0.0"]
For easier container management, use docker-compose.yaml
:
services: app: build: context: . dockerfile: Dockerfile working_dir: /app volumes: - ./app:/app/app ports: - "${APP_PORT:-8000}:8000" environment: - DATABASE_URL=${DATABASE_URL} depends_on: - postgres postgres: image: postgres:15 environment: POSTGRES_DB: ${POSTGRES_DB} POSTGRES_USER: ${POSTGRES_USER} POSTGRES_PASSWORD: ${POSTGRES_PASSWORD} volumes: - postgres_data:/var/lib/postgresql/data volumes: postgres_data: {}
Create a .env
file with environment variables. Run with: docker compose up --build
.
[tool.uv]
and Development Tools
The [tool.uv]
section in pyproject.toml
lists development tools:
- pytest: Testing framework (out of scope here).
- mypy: Static type checker. Run manually:
uv run mypy app
. - ruff: Fast linter (replaces multiple tools).
- pre-commit: Manages pre-commit hooks. Create
.pre-commit-config.yaml
:
repos: - repo: https://github.com/pre-commit/pre-commit-hooks rev: v4.4.0 hooks: - id: check-added-large-files - id: check-toml - id: check-yaml args: - --unsafe - id: end-of-file-fixer - id: trailing-whitespace - repo: https://github.com/astral-sh/ruff-pre-commit rev: v0.8.6 hooks: - id: ruff args: [--fix] - id: ruff-format
Add pyproject.toml
configurations for mypy
and ruff
(example provided in the original text). Install a VS Code Ruff extension for real-time linting. This setup ensures consistent code style, type checking, and pre-commit checks for a streamlined workflow.
The above is the detailed content of Scalable Python backend: Building a containerized FastAPI Application with uv, Docker, and pre-commit: a step-by-step guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










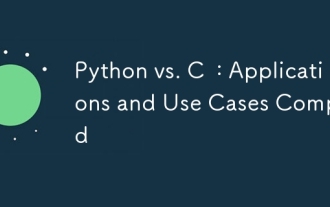
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
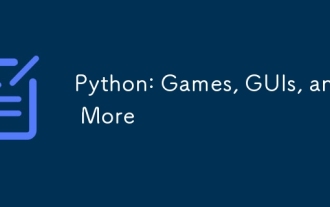
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
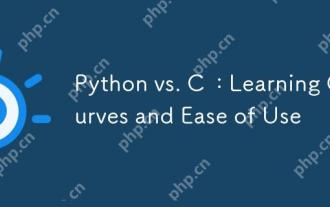
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
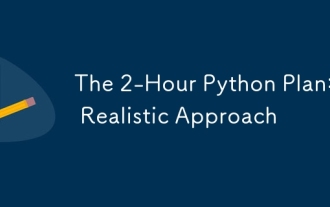
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
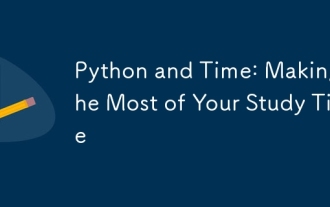
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
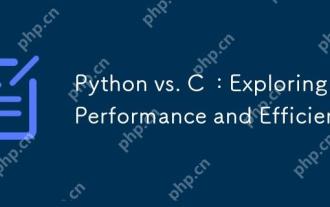
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
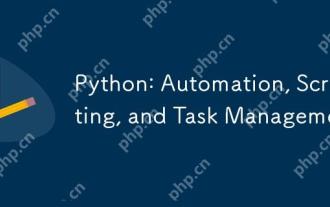
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
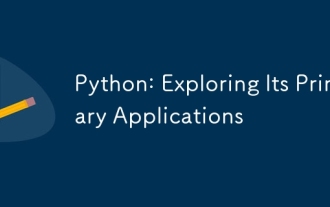
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
