Working with AddrPort in net/netip: Complete Guide
Continuing our exploration of the net/netip
package, we now focus on AddrPort
, a structure elegantly combining IP addresses and port numbers. This pairing is fundamental in network programming, crucial for web servers, database connections, and virtually any network service.
Why Use AddrPort?
Prior to net/netip
, managing IP:port combinations often involved string manipulation, leading to parsing complexities and potential errors. AddrPort
provides a streamlined, type-safe alternative.
Getting Started with AddrPort
Let's begin with the basics:
package main import ( "fmt" "net/netip" ) func main() { // Create from a string ap1, err := netip.ParseAddrPort("192.168.1.1:8080") if err != nil { panic(err) } // Create from Addr and port addr := netip.MustParseAddr("192.168.1.1") ap2 := netip.AddrPortFrom(addr, 8080) fmt.Printf("From string: %v\nFrom components: %v\n", ap1, ap2) }
Key points regarding the port number:
- Must be within the range 0-65535.
- Stored as a
uint16
. - Leading zeros are permitted during parsing ("8080" and "08080" are equivalent).
Exploring AddrPort Methods
Let's examine the methods available for AddrPort
and their applications.
Accessing Address and Port Components
func examineAddrPort(ap netip.AddrPort) { // Retrieve the address component addr := ap.Addr() fmt.Printf("Address: %v\n", addr) // Retrieve the port number port := ap.Port() fmt.Printf("Port: %d\n", port) // Obtain the string representation ("<addr>:<port>") str := ap.String() fmt.Printf("String representation: %s\n", str) }
Handling IPv4 and IPv6 Addresses
AddrPort
seamlessly supports both IPv4 and IPv6:
func handleBothIPVersions() { // IPv4 with port ap4 := netip.MustParseAddrPort("192.168.1.1:80") // IPv6 with port ap6 := netip.MustParseAddrPort("[2001:db8::1]:80") // Note: Brackets are required for IPv6 addresses. "2001:db8::1:80" would fail. // IPv6 with zone and port apZone := netip.MustParseAddrPort("[fe80::1%eth0]:80") fmt.Printf("IPv4: %v\n", ap4) fmt.Printf("IPv6: %v\n", ap6) fmt.Printf("IPv6 with zone: %v\n", apZone) }
Real-World Applications of AddrPort
Let's explore practical scenarios where AddrPort
excels.
1. A Simple TCP Server
func runServer(ap netip.AddrPort) error { listener, err := net.Listen("tcp", ap.String()) if err != nil { return fmt.Errorf("failed to start server: %w", err) } defer listener.Close() fmt.Printf("Server listening on %v\n", ap) for { conn, err := listener.Accept() if err != nil { return fmt.Errorf("accept failed: %w", err) } go handleConnection(conn) } } func handleConnection(conn net.Conn) { defer conn.Close() // Handle the connection... }
2. A Service Registry
This example demonstrates a service registry managing services and their endpoints:
// ... (ServiceRegistry struct and methods as in the original example) ...
3. Load Balancer Configuration
Here's how AddrPort
can be used in a load balancer configuration:
// ... (LoadBalancer struct and methods as in the original example) ...
Common Patterns and Best Practices
- Input Validation: Always validate user-provided input:
func validateEndpoint(input string) error { _, err := netip.ParseAddrPort(input) if err != nil { return fmt.Errorf("invalid endpoint %q: %w", input, err) } return nil }
- Zero Value Handling: The zero value of
AddrPort
is invalid:
func isValidEndpoint(ap netip.AddrPort) bool { return ap.IsValid() }
- String Representation: When storing
AddrPort
as strings (e.g., in configuration files):
func saveConfig(endpoints []netip.AddrPort) map[string]string { config := make(map[string]string) for i, ep := range endpoints { key := fmt.Sprintf("endpoint_%d", i) config[key] = ep.String() } return config }
Integration with the Standard Library
AddrPort
integrates seamlessly with the standard library:
func dialService(endpoint netip.AddrPort) (net.Conn, error) { return net.Dial("tcp", endpoint.String()) } func listenAndServe(endpoint netip.AddrPort, handler http.Handler) error { return http.ListenAndServe(endpoint.String(), handler) }
Performance Considerations
- Prefer AddrPortFrom: When you already have a valid
Addr
, useAddrPortFrom
instead of string parsing for improved efficiency:
addr := netip.MustParseAddr("192.168.1.1") ap := netip.AddrPortFrom(addr, 8080) // More efficient than parsing "192.168.1.1:8080"
-
Minimize String Conversions: Keep addresses in
AddrPort
format as much as possible, converting to strings only when necessary.
What's Next?
Our next article will cover the Prefix
type, focusing on CIDR notation and subnet operations, completing our exploration of core net/netip
types. Until then, leverage the power and efficiency of AddrPort
in your network applications!
The above is the detailed content of Working with AddrPort in net/netip: Complete Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










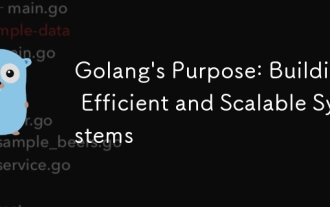
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
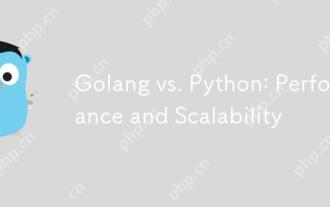
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
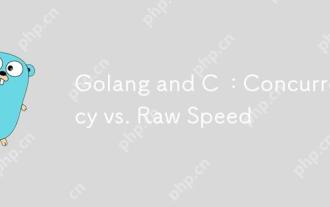
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
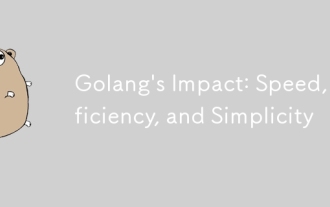
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
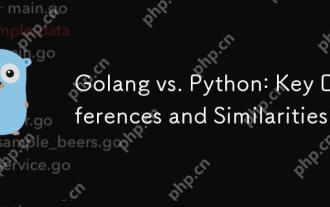
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
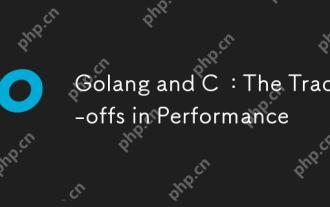
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
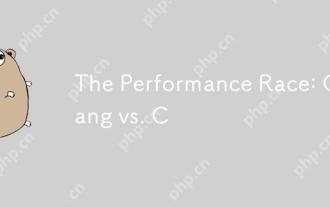
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
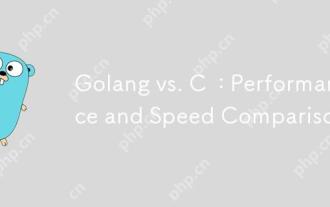
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
