Understanding Selection Sort Algorithm (with Examples in Java)
Selection Sort: A Step-by-Step Guide
Selection Sort is a straightforward sorting algorithm. It repeatedly finds the minimum element from the unsorted part of the list and places it at the beginning. This process continues until the entire list is sorted.
How Selection Sort Works
Let's illustrate with an example, sorting this array in ascending order:
Iteration 1:
The goal is to place the smallest element at the beginning. We start by assuming the first element is the minimum.
We compare the current minimum with each subsequent element, updating the minimum if a smaller element is found.
This continues until the actual minimum is identified.
Finally, we swap the minimum element with the first element.
The first element is now sorted. Subsequent iterations will only consider the unsorted portion.
Subsequent Iterations:
This process repeats for each remaining unsorted element.
The algorithm iterates n-1 times (where n is the array's length). After the fifth iteration (for a six-element array), the last element is implicitly sorted.
Selection Sort Implementation (Java):
import java.util.Arrays; public class SelectionSortTest { public static void main(String[] args) { int[] arr = {8, 2, 6, 4, 9, 1}; System.out.println("Unsorted array: " + Arrays.toString(arr)); selectionSort(arr); System.out.println("Sorted array: " + Arrays.toString(arr)); } public static void selectionSort(int[] arr) { int size = arr.length; // Iterate through the array size-1 times for (int i = 0; i < size - 1; i++) { int minIndex = i; // Find the minimum element in the unsorted part for (int j = i + 1; j < size; j++) { if (arr[j] < arr[minIndex]) { minIndex = j; } } // Swap the minimum element with the first unsorted element int temp = arr[minIndex]; arr[minIndex] = arr[i]; arr[i] = temp; } } }
Output:
Unsorted array: [8, 2, 6, 4, 9, 1] Sorted array: [1, 2, 4, 6, 8, 9]
Complexity Analysis:
- Time Complexity: O(n²) in all cases (best, average, worst). The nested loops always execute a fixed number of times regardless of the input order.
- Space Complexity: O(1). It's an in-place algorithm, requiring constant extra space.
Conclusion:
Selection Sort's O(n²) time complexity makes it inefficient for large datasets. It's best suited for small arrays or situations where simplicity is prioritized over performance.
The above is the detailed content of Understanding Selection Sort Algorithm (with Examples in Java). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










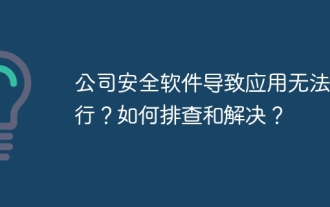
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
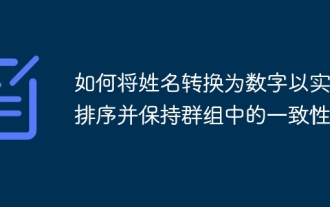
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
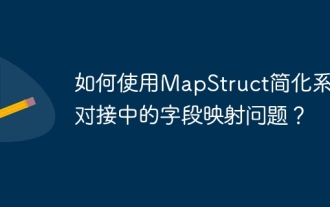
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
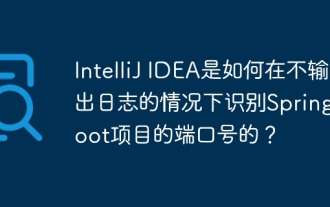
Start Spring using IntelliJIDEAUltimate version...
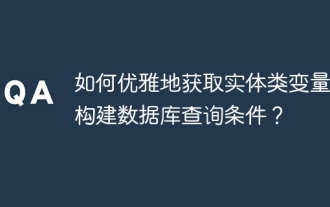
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
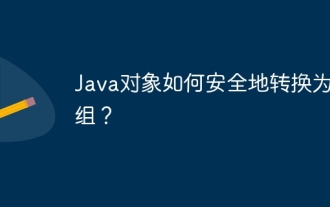
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
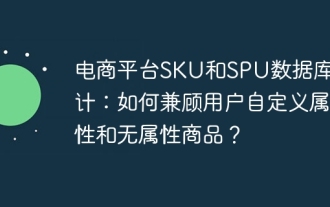
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
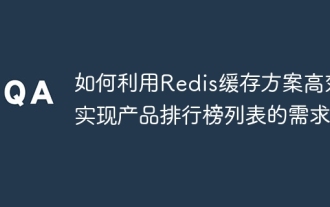
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
