


How to Efficiently Retrieve a Row's Position in a MySQL ORDER BY Clause?
Efficiently obtain row position in MySQL ORDER BY clause
Sorting of data in database tables provides a structured view of records. However, sometimes it is necessary to determine the position of a specific row in the sorted results. This article explores how to achieve this using the ORDER BY clause in MySQL and provides an optimized solution for retrieving a single row and its location.
Problem Statement:
Consider a MySQL table named "tbl" which contains two columns: "id" and "name". When sorted by the "name" column in ascending order, the table data looks like this:
<code>+-----------+--------------------+ | id | name | +-----------+--------------------+ | 5 | Alpha | | 7 | Beta | | 3 | Delta | | ... | ... | | 1 | Zed | +-----------+--------------------+</code>
Target:
The goal is to retrieve the "Beta" row from the sorted table and its position in the result set. The desired output should be:
<code>+-----------+-------------+--------------+ | id | position | name | +-----------+-------------+--------------+ | 7 | 2 | Beta | +-----------+-------------+--------------+</code>
Option 1: Optimized query
To retrieve the position of a specific row in the ORDER BY clause, we can use a subquery to assign row numbers incrementally based on the sort order. The following query effectively achieves this:
SELECT x.id, x.position, x.name FROM (SELECT t.id, t.name, @rownum := @rownum + 1 AS position FROM tbl t JOIN (SELECT @rownum := 0) r ORDER BY t.name) x WHERE x.name = 'Beta'
In this query, we create a subquery (enclosed in parentheses) that uses a user-defined variable (@rownum) to assign incrementing row numbers. Do a JOIN with a virtual table initialized with @rownum := 0, making sure the numbers start at 1. The WHERE clause filters the results to retrieve only rows with the name "Beta".
Option 2: Alternative query
The following alternative query can be formulated to obtain the location:
SELECT t.id, (SELECT COUNT(*) FROM tbl x WHERE x.name < t.name) + 1 AS position, t.name FROM tbl t WHERE t.name = 'Beta';
Unlike scenario one, this query calculates the position by counting the number of rows with the same name or less than the target name. However, it is important to note that this approach may result in duplication of rows with the same name, assigning them the same position value.
The above is the detailed content of How to Efficiently Retrieve a Row's Position in a MySQL ORDER BY Clause?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
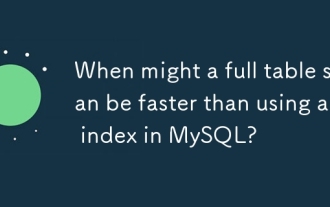
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
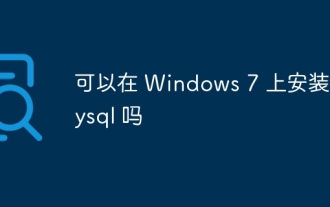
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
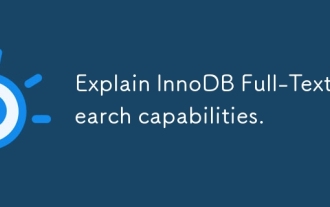
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
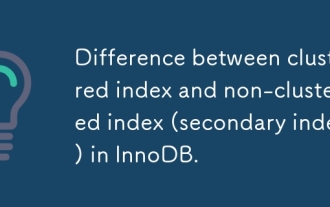
The difference between clustered index and non-clustered index is: 1. Clustered index stores data rows in the index structure, which is suitable for querying by primary key and range. 2. The non-clustered index stores index key values and pointers to data rows, and is suitable for non-primary key column queries.
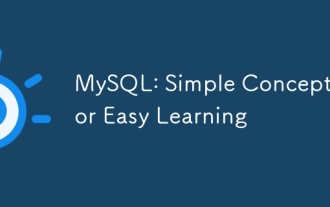
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
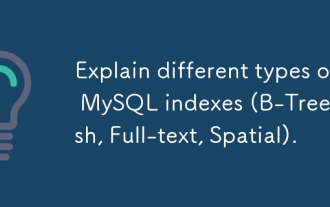
MySQL supports four index types: B-Tree, Hash, Full-text, and Spatial. 1.B-Tree index is suitable for equal value search, range query and sorting. 2. Hash index is suitable for equal value searches, but does not support range query and sorting. 3. Full-text index is used for full-text search and is suitable for processing large amounts of text data. 4. Spatial index is used for geospatial data query and is suitable for GIS applications.
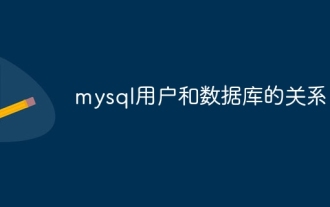
In MySQL database, the relationship between the user and the database is defined by permissions and tables. The user has a username and password to access the database. Permissions are granted through the GRANT command, while the table is created by the CREATE TABLE command. To establish a relationship between a user and a database, you need to create a database, create a user, and then grant permissions.
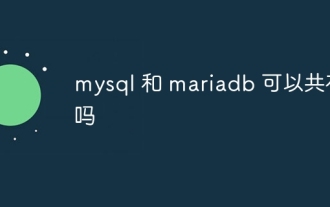
MySQL and MariaDB can coexist, but need to be configured with caution. The key is to allocate different port numbers and data directories to each database, and adjust parameters such as memory allocation and cache size. Connection pooling, application configuration, and version differences also need to be considered and need to be carefully tested and planned to avoid pitfalls. Running two databases simultaneously can cause performance problems in situations where resources are limited.
