Advanced Event Handling Patterns in JavaScript
JavaScript event handling is the core of building dynamic interactive web applications. While basic event handling (e.g., addEventListener
) is simple, advanced patterns allow developers to optimize performance, handle complex user interactions, and write easy-to-maintain code.
This article explores advanced event handling patterns in JavaScript and provides practical examples to improve your event handling skills.
-
Event delegation
What is event delegation?
Event delegation refers to attaching a single event listener to a parent element to manage events for its child elements. This mode is particularly useful for elements that are dynamically added to the DOM after the page loads.
Example:
document.getElementById("parent").addEventListener("click", function(event) { if (event.target && event.target.matches(".child")) { console.log("点击了子元素:", event.target.textContent); } });
Why use event delegation?
- Reduce the number of event listeners and improve performance.
- Simplify the management of dynamically added elements.
-
Throttle and anti-shake
What are they?
- ThrottlingEnsures that the function is executed at most once within the specified interval.
- AntishakeDelays the execution of a function until a certain amount of time has elapsed since the last event.
Example:
Throttling
function throttle(func, limit) { let lastCall = 0; return function(...args) { const now = Date.now(); if (now - lastCall >= limit) { lastCall = now; func.apply(this, args); } }; } window.addEventListener( "resize", throttle(() => { console.log("窗口大小已调整!"); }, 200) );
Anti-Shake
function debounce(func, delay) { let timer; return function(...args) { clearTimeout(timer); timer = setTimeout(() => func.apply(this, args), delay); }; } const searchInput = document.getElementById("search"); searchInput.addEventListener( "input", debounce(() => { console.log("输入事件触发!"); }, 300) );
Why use them?
- Improve performance by reducing redundant function calls, especially during high-frequency events such as resizing or scrolling.
-
Custom event emitter
What are they?
Custom event emitters allow developers to create, dispatch and listen to their own events for increased modularity.
const eventEmitter = { events: {}, on(event, listener) { if (!this.events[event]) this.events[event] = []; this.events[event].push(listener); }, emit(event, data) { if (this.events[event]) { this.events[event].forEach((listener) => listener(data)); } }, }; eventEmitter.on("dataReceived", (data) => { console.log("收到数据:", data); }); eventEmitter.emit("dataReceived", { id: 1, message: "Hello!" });
Why use them?
- Enhanced modularization and decoupling of components.
- Facilitates communication between different parts of the application.
-
One-time event handling
What is one-time event processing?
Sometimes you only need an event handler to execute once. Modern JavaScript provides an elegant way to handle this problem.
Example
const button = document.getElementById("myButton"); button.addEventListener( "click", () => { console.log("按钮被点击!"); }, { once: true } );
Why use it?
- Simplify the logic of one-time events.
- Avoid memory leaks by automatically removing listeners.
-
Event handler combination
What is event handler composition?
Event handler composition involves combining multiple handlers to handle events sequentially.
Example
function composeHandlers(...handlers) { return function(event) { handlers.forEach((handler) => handler(event)); }; } function logClick(event) { console.log("点击:", event.target); } function changeBackground(event) { event.target.style.backgroundColor = "yellow"; } document.getElementById("myElement").addEventListener( "click", composeHandlers(logClick, changeBackground) );
Why use it?
- Keep handlers small and reusable.
- Promote clean and maintainable code.
-
Capture and Bubbling
What are they?
JavaScript event flow is divided into two stages:
- Capture phase: Events flow from the root element to the target element.
- Bubbling phase: Events flow from the target element back to the root element.
Example
document.getElementById("parent").addEventListener("click", function(event) { if (event.target && event.target.matches(".child")) { console.log("点击了子元素:", event.target.textContent); } });
Why use it?
- Provides flexibility in managing event propagation.
-
Block default behavior and stop propagation
What are they?
preventDefault()
Prevent default browser actions (e.g. form submission).stopPropagation()
Prevents events from propagating to other listeners.
Example
function throttle(func, limit) { let lastCall = 0; return function(...args) { const now = Date.now(); if (now - lastCall >= limit) { lastCall = now; func.apply(this, args); } }; } window.addEventListener( "resize", throttle(() => { console.log("窗口大小已调整!"); }, 200) );
Why use it?
- Provides finer control over event behavior.
Conclusion
Advanced event handling patterns are essential for building efficient, interactive, and easy-to-maintain JavaScript applications. By mastering techniques like event delegation, throttling, custom emitters, and propagation controls, you can tackle complex use cases with ease.
The above is the detailed content of Advanced Event Handling Patterns in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










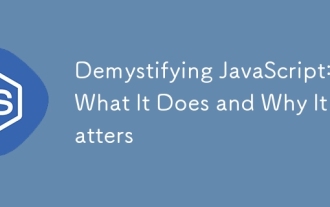
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
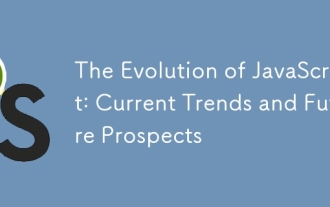
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
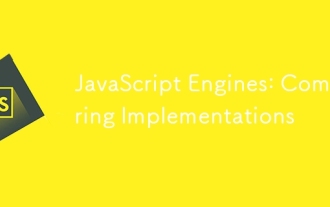
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
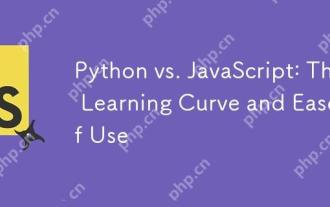
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
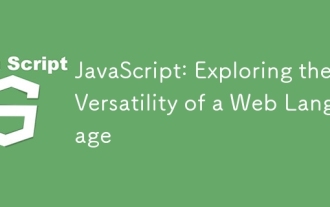
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
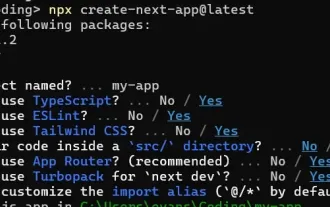
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
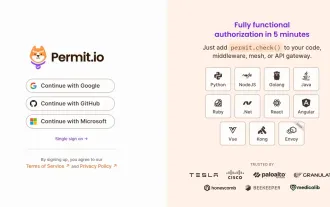
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
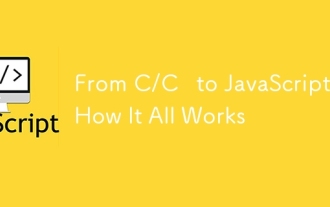
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
