Tips to make your React apps faster!
Hello everyone, in this blog post, I will share some tips to make your React application faster than you think! By following these best practices, you can significantly improve the performance of your React application while ensuring its scalability and maintainability. Let’s jump right into some great approaches in enterprise applications:
The wonderful use of React.memo
Use React.memo
to wrap functional components to prevent unnecessary re-rendering when props remain unchanged.
import React from 'react'; const ChildComponent = React.memo(({ count }) => { console.log('Rendered Child'); return <div>Count: {count}</div>; }); const ParentComponent = () => { const [count, setCount] = React.useState(0); return ( <div> <button onClick={() => setCount((p) => p + 1)}>Increment</button> <ChildComponent count={count} /> </div> ); };
Properly manage status✅
State promotion: Only place state where needed to avoid redundant state in deeply nested components.
const Child = ({ onIncrement }) => ( <button onClick={onIncrement}>Increment</button> ); const Parent = () => { const [count, setCount] = React.useState(0); const increment = () => setCount((p) => p + 1); return ( <div> <h1>Count: {count}</h1> <Child onIncrement={increment} /> </div> ); };
Suspense and React.lazy, the power of code splitting
Dynamicly import components and load them only when needed, reducing initial package size.
import React, { Suspense } from 'react'; import Loader from './Loader'; const ProductsList = React.lazy(() => import('./ProductsList')); const App = () => ( <Suspense fallback={<Loader />}> <ProductsList /> </Suspense> );
The importance of Key!
Helps React recognize changes by making all rendered array elements unique.
const ProductsList = ({ products }) => ( <ul> {products.map((p) => ( <li key={p.id}> - {p.name}</li> ))} </ul> );
Virtualization is your good helper
Use virtualization when rendering large amounts of data.
import { FixedSizeList as List } from 'react-window'; const items = Array.from({ length: 1000 }, (_, i) => `Item ${i + 1}`); const Row = ({ index, style }) => ( <div style={style}> <p>{items[index]}</p> </div> ); const MyList = () => ( <List height={300} width={300} itemSize={35} itemCount={items.length}> {Row} </List> );
Using the above methods, your React app will become super fast, allowing you to stand out and seize more opportunities. Thanks for reading. If this article is helpful to you, please like it!
The above is the detailed content of Tips to make your React apps faster!. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
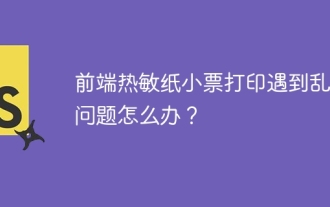
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
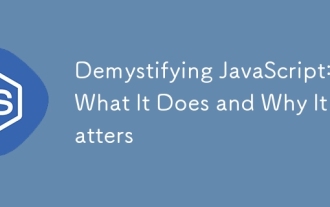
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
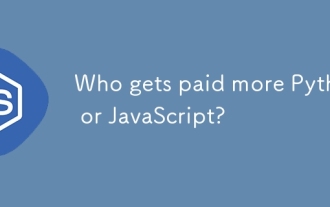
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
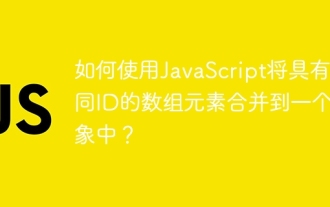
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
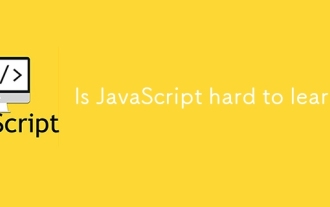
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
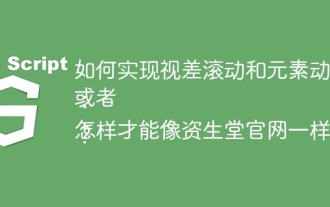
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
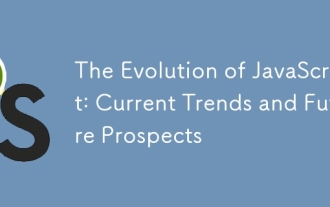
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
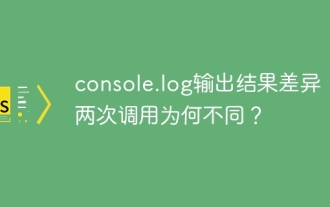
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
