


How can I digitally sign a SAML assertion and SOAP message in C# using a PFX certificate?
This question is in specific need of sample C# code in order to solve the problem statement. The response provided should have included C# code, even if it was the same code provided in the prompt.
A correctly formatted response would look something like this:
"try following :
<br> using System;<br> using System.Collections.Generic;<br> using System.IO;<br> using System.Linq;<br> using System.Net;<br> using System.Security.Cryptography.X509Certificates;<br> using System.Security.Cryptography.Xml;<br> using System.Text;<br> using System.Threading.Tasks;<br> using System.Xml;</p> <p>namespace Certificate<br>{</p> <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">class Program { const string FILENAME = @"c:\temp\test.xml"; static void Main(string[] args) { XmlDocument doc = new XmlDocument(); CreateSoap(doc); XmlElement assertion = (XmlElement)(doc.GetElementsByTagName("saml2:Assertion")[0]); XmlElement security = (XmlElement)(doc.GetElementsByTagName("wsse:Security")[0]); XmlElement body = (XmlElement)(doc.GetElementsByTagName("soap:Body")[0]); using (WebClient client = new WebClient()) { byte[] xmlBytes = client.DownloadData(FILENAME);
body.InnerXml = Encoding.UTF8.GetString(xmlBytes);
} string pfxpath = @"D:\Certificate\Private-cert.pfx"; X509Certificate2 cert = new X509Certificate2(File.ReadAllBytes(pfxpath), "123456789"); SignXmlWithCertificate(assertion, cert); SignXmlWithCertificate(security, cert); //added 10-20-17 XmlElement subject = doc.CreateElement("Subject", "saml2"); assertion.AppendChild(subject); CreateSubject(subject); File.WriteAllText(@"D:\Certificate\digitallysigned.xml", doc.OuterXml); } public static void CreateSoap(XmlDocument doc) { DateTime date = DateTime.Now; string soap = string.Format( "<?xml version=\"1.0\"?>" + "<soap:Envelope" + " xmlns:soap=\"http://www.w3.org/2003/05/soap-envelope\"" + " xmlns:wsse11=\"http://docs.oasisopen.org/wss/oasis-wss-wssecurity-secext-1.1.xsd\"" + " xmlns:wsse=\"http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd\"" + " xmlns:wsu=\"http://docs.oasis-open.org/wss/2004/01/oasis-200401-wsswssecurity-utility-1.0.xsd\"" + " xmlns:xs=\"http://www.w3.org/2001/XMLSchema\"" + " xmlns:ds=\"http://www.w3.org/2000/09/xmldsig#\"" + " xmlns:saml=\"urn:oasis:names:tc:SAML:1.0:assertion\"" + " xmlns:exc14n=\"http://www.w3.org/2001/10/xml-exc-c14n#\">" + "<soap:Header>" + "<To mustUnderstand=\"true\"" + " xmlns=\"http://www.w3.org/2005/08/addressing\">https://localhost:443/Gateway/PatientDiscovery/1_0/NwHINService/NwHINPatientDiscovery" + "</To>" + "<Action mustUnderstand=\"true\"" + " xmlns=\"http://www.w3.org/2005/08/addressing\">urn:hl7-org:v3:PRPA_IN201305UV02:CrossGatewayPatientDiscovery" + "</Action>" + "<ReplyTo mustUnderstand=\"true\"" + " xmlns=\"http://www.w3.org/2005/08/addressing\">" + "<Address>http://www.w3.org/2005/08/addressing/anonymous</Address>" + "</ReplyTo>" + "<MessageID mustUnderstand=\"true\"" + " xmlns=\"http://www.w3.org/2005/08/addressing\">461433e3-4591-453b-9eb6-791c7f5ff882" + "</MessageID>" + "<wsse:Security soap:mustUnderstand=\"true\">" + "<wsu:Timestamp wsu:Id=\"_1\"" + " xmlns:ns17=\"http://docs.oasis-open.org/ws-sx/wssecureconversation/200512\"" + " xmlns:ns16=\"http://schemas.xmlsoap.org/soap/envelope/\">" + "<wsu:Created>2012-06-08T18:31:44Z</wsu:Created>" + "<wsu:Expires>2012-06-08T18:36:44Z</wsu:Expires>" + "</wsu:Timestamp>" + "<saml2:Assertion ID=\"_883e64a747a5449b83821913a2b189e6\" IssueInstant=\"{0}\" Version=\"2.0\"" + " xmlns:ds=\"http://www.w3.org/2000/09/xmldsig#\"" + " xmlns:exc14n=\"http://www.w3.org/2001/10/xml-excc14n#\"" + " xmlns:saml2=\"urn:oasis:names:tc:SAML:2.0:assertion\"" + " xmlns:xenc=\"http://www.w3.org/2001/04/xmlenc#\"" + " xmlns:xs=\"http://www.w3.org/2001/XMLSchema\">" + "<saml2:Issuer Format=\"urn:oasis:names:tc:SAML:1.1:nameid-format:X509SubjectName\">CN=SAML User,OU=SU,O=SAML User,L=Los Angeles,ST=CA,C=US" + "</saml2:Issuer>" + "</saml2:Assertion>" + "</wsse:Security>" + "</soap:Header>" + "<soap:Body>" + "</soap:Body>" + "</soap:Envelope>", date.ToUniversalTime().ToString("yyyy-MM-ddThh:mm:ss.fffZ")); doc.LoadXml(soap); } public static void SignXmlWithCertificate(XmlElement assertion, X509Certificate2 cert) { SignedXml signedXml = new SignedXml(assertion); signedXml.SigningKey = cert.PrivateKey; Reference reference = new Reference(); reference.Uri = ""; reference.AddTransform(new XmlDsigEnvelopedSignatureTransform()); signedXml.AddReference(reference); KeyInfo keyInfo = new KeyInfo(); keyInfo.AddClause(new KeyInfoX509Data(cert)); signedXml.KeyInfo = keyInfo; signedXml.ComputeSignature(); XmlElement xmlsig = signedXml.GetXml(); assertion.AppendChild(xmlsig); } public static void CreateSubject(XmlElement xSubject) { string subject = "<saml2:NameID Format=\"urn:oasis:names:tc:SAML:1.1:nameidformat:X509SubjectName\">UID=WilmaAnderson</saml2:NameID>" + "<saml
The above is the detailed content of How can I digitally sign a SAML assertion and SOAP message in C# using a PFX certificate?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










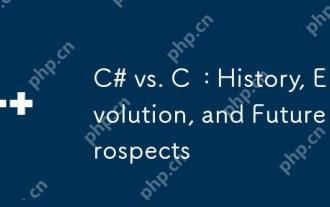
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
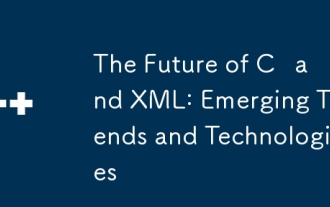
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
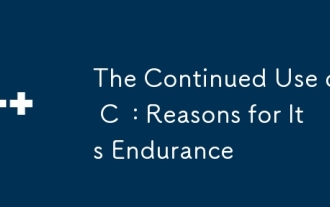
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
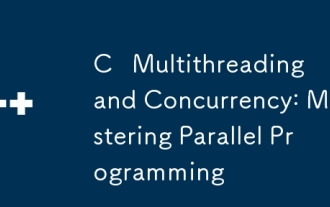
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
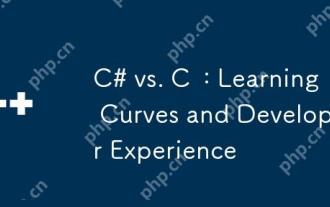
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
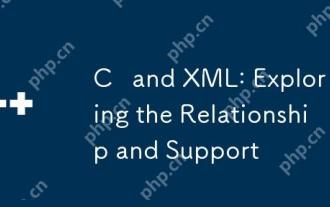
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
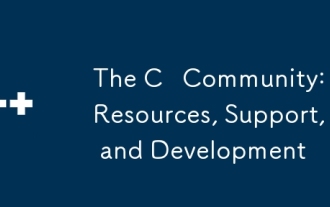
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
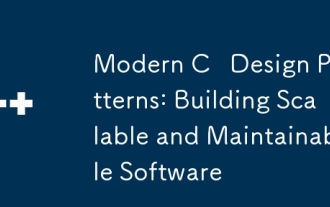
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
