How Hibernate ORM Works Under the Hood
Hibernate ORM: A Deep Dive into its Inner Workings
Hibernate ORM simplifies database interaction in Java applications by bridging the gap between Java objects and database tables. This abstraction streamlines CRUD (Create, Read, Update, Delete) operations.
1.1 Mapping Java Objects to Database Tables
Hibernate uses metadata annotations or XML configuration files to map Java classes to database tables. Each class represents a table, and each class field corresponds to a table column. For example:
@Entity @Table(name = "users") public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "username") private String username; @Column(name = "email") private String email; // Getters and setters }
Mapping options include:
-
Annotations: Annotations within Java classes define the mapping (e.g.,
@Entity
,@Table
,@Column
). - XML Configuration: XML files provide an alternative mapping definition.
Hibernate uses configuration files (like hibernate.cfg.xml
) to establish database connections, define dialects, and configure other settings. This file dictates database connectivity and specifics.
1.2 The Hibernate Session
The Session
object facilitates database interaction, providing CRUD methods and managing object persistence.
A SessionFactory
, a thread-safe object, creates Session
instances and manages caching and transactions.
The Session
lifecycle:
- Open Session: Initiates database interaction.
- Transaction Management: Ensures atomicity within transactions.
- Session Operations: Performs CRUD operations.
- Close Session: Terminates the database connection after operations.
-
Transaction Management in Hibernate
Hibernate's transaction management ensures that database operations are atomic—either all succeed or all fail.
The Transaction
interface (accessed via the Session
object) manages transactions:
-
Begin Transaction:
beginTransaction()
starts a transaction. -
Commit Transaction:
commit()
saves changes to the database. -
Rollback Transaction:
rollback()
undoes changes in case of errors.
The Session
maintains a persistence context, tracking entity changes and database synchronization.
- Auto-Flush: Hibernate automatically flushes changes upon transaction commit.
- Transaction Synchronization: Hibernate synchronizes transactions with the database using JTA or JDBC. JTA supports distributed transactions and Java EE integration.
-
Hibernate's Internal Mechanisms
3.1 Bytecode Enhancement
Bytecode enhancement optimizes entity operations by modifying Java class bytecode at runtime.
How it Works:
- Instrumentation: Hibernate instruments bytecode to inject logic without modifying source code, often using tools like Java agents or libraries like ASM or Javassist.
- Enhancement Capabilities: This includes lazy loading, dirty checking, and optimized field access.
Enhancement can occur during build time (Maven, Gradle plugins) or runtime (Java agents, Hibernate configurations).
Benefits: Improved performance, reduced memory usage, and fewer database queries.
3.2 Proxy Objects
Proxy objects, primarily used for lazy loading, enhance performance.
How they Work:
- Lazy Loading: For lazy-loaded associations, Hibernate returns a proxy object instead of immediately loading associated data.
- Proxy Characteristics: The proxy acts as a placeholder, loading data only when accessed. Access is transparent to the developer.
Hibernate creates dynamic proxies at runtime, extending the entity class and intercepting method calls. Static proxies are also possible, particularly with bytecode enhancement.
Benefits: Efficient data loading, reduced memory usage, and fewer database queries.
-
Conclusion
Hibernate ORM is a powerful tool for efficient database management in Java. Understanding its internal mechanisms—object mapping, transaction handling, and performance optimizations—allows developers to fully utilize its capabilities. For further details, see: How Hibernate ORM Works Under the Hood
The above is the detailed content of How Hibernate ORM Works Under the Hood. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


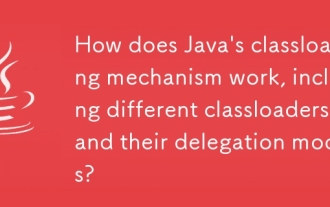
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
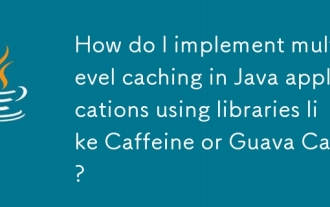
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
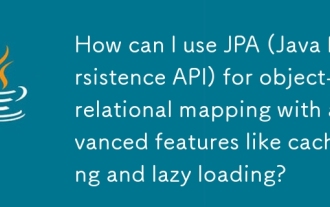
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
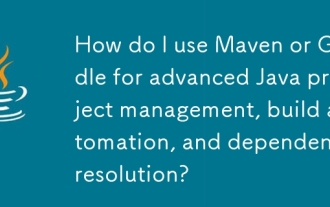
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
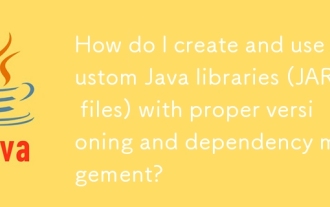
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
