How to use Scrapy and proxy IP to crawl data efficiently
In today's data-driven world, web scraping is crucial for businesses and individuals seeking online information. Scrapy, a powerful open-source framework, excels at efficient and scalable web crawling. However, frequent requests often trigger target websites' anti-scraping measures, leading to IP blocks. This article details how to leverage Scrapy with proxy IPs for effective data acquisition, including practical code examples and a brief mention of 98IP proxy as a potential service.
I. Understanding the Scrapy Framework
1.1 Scrapy's Core Components
The Scrapy architecture comprises key elements: Spiders (defining crawling logic and generating requests), Items (structuring scraped data), Item Loaders (efficiently populating Items), Pipelines (processing and storing scraped Items), Downloader Middlewares (modifying requests and responses), and Extensions (providing additional functionality like statistics and debugging).
1.2 Setting Up a Scrapy Project
Begin by creating a Scrapy project using scrapy startproject myproject
. Next, within the spiders
directory, create a Python file defining your Spider class and crawling logic. Define your data structure in items.py
and data processing flow in pipelines.py
. Finally, run your Spider with scrapy crawl spidername
.
II. Integrating Proxy IPs with Scrapy
2.1 The Need for Proxy IPs
Websites employ anti-scraping techniques like IP blocking and CAPTCHAs to protect their data. Proxy IPs mask your real IP address, allowing you to circumvent these defenses by dynamically changing your IP, thereby increasing scraping success rates and efficiency.
2.2 Configuring Proxy IPs in Scrapy
To use proxy IPs, create a custom Downloader Middleware. Here's a basic example:
# middlewares.py import random class RandomProxyMiddleware: PROXY_LIST = [ 'http://proxy1.example.com:8080', 'http://proxy2.example.com:8080', # ... Add more proxies ] def process_request(self, request, spider): proxy = random.choice(self.PROXY_LIST) request.meta['proxy'] = proxy
Enable this middleware in settings.py
:
# settings.py DOWNLOADER_MIDDLEWARES = { 'myproject.middlewares.RandomProxyMiddleware': 543, }
Note: The PROXY_LIST
is a placeholder. In practice, use a third-party service like 98IP Proxy for dynamic proxy IP acquisition. 98IP Proxy offers a robust API and high-quality proxy pool.
2.3 Proxy IP Rotation and Error Handling
To prevent single proxy IP blocks, implement proxy rotation. Handle request failures (e.g., invalid proxies, timeouts) with error handling. Here's an improved Middleware:
# middlewares.py (Improved) import random import time from scrapy.downloadermiddlewares.retry import RetryMiddleware from scrapy.exceptions import NotConfigured, IgnoreRequest from scrapy.utils.response import get_response_for_exception class ProxyRotatorMiddleware: PROXY_LIST = [] # Dynamically populate from 98IP Proxy or similar PROXY_POOL = set() PROXY_ERROR_COUNT = {} # ... (Initialization and other methods, similar to the original example but with dynamic proxy fetching and error handling) ...
This enhanced middleware includes a PROXY_POOL
for available proxies, PROXY_ERROR_COUNT
for tracking errors, and a refresh_proxy_pool
method for dynamically updating proxies from a service like 98IP Proxy. It also incorporates error handling and retry logic.
III. Strategies for Efficient Crawling
3.1 Concurrency and Rate Limiting
Scrapy supports concurrent requests, but excessive concurrency can lead to blocks. Adjust CONCURRENT_REQUESTS
and DOWNLOAD_DELAY
in settings.py
to optimize concurrency and avoid overwhelming the target website.
3.2 Data Deduplication and Cleaning
Implement deduplication (e.g., using sets to store unique IDs) and data cleaning (e.g., using regular expressions to remove noise) in your Pipelines to enhance data quality.
3.3 Exception Handling and Logging
Robust exception handling and detailed logging (using Scrapy's built-in logging capabilities and configuring LOG_LEVEL
) are essential for identifying and addressing issues during the crawling process.
IV. Conclusion
Combining Scrapy with proxy IPs for efficient web scraping requires careful consideration. By properly configuring Downloader Middlewares, utilizing a reliable proxy service (such as 98IP Proxy), implementing proxy rotation and error handling, and employing efficient crawling strategies, you can significantly improve your data acquisition success rate and efficiency. Remember to adhere to legal regulations, website terms of service, and responsible proxy usage to avoid legal issues or service bans.
The above is the detailed content of How to use Scrapy and proxy IP to crawl data efficiently. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










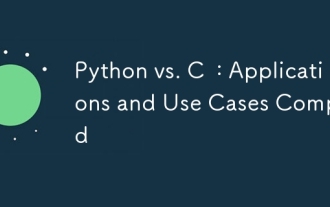
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
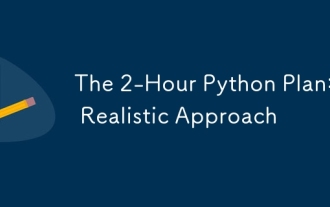
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
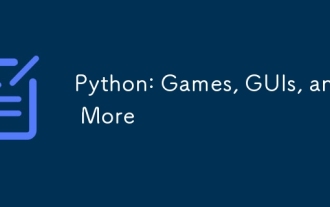
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
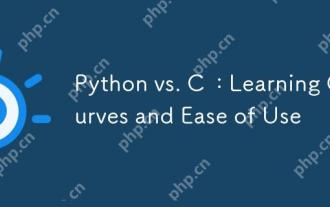
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
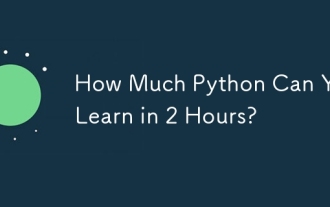
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
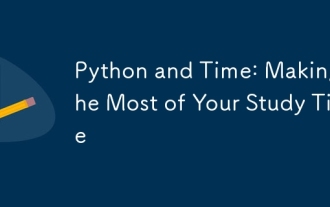
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
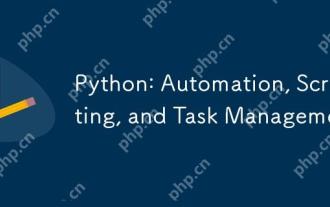
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
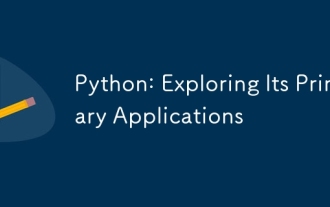
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
