A Quick Intro to React.js
React empowers the creation of dynamic websites rendered entirely within the browser. Its core element is JSX, a syntax resembling HTML and XML.
Language | Full Form | Use | Tags |
---|---|---|---|
HTML | Hyper Text Markup Language | Website Development | Predefined tags (e.g., H1, H2, P) |
XML | Extensible Markup Language | Data Structuring | Customizable Tags |
JSX | JavaScript XML | Combines HTML and JavaScript | HTML & Custom Tags |
Illustrative Examples
HTML
<div> <h1>This is heading 1</h1> <p>This is an example paragraph in HTML</p> </div>
XML
<example><title>XML Example <note>This is a note</note><data1>Example Data</data1><data2>Example Data</data2></title></example>
JSX
function ExampleReactComponent() { return ( <div> <h1>This is heading 1</h1> <p>This is an example paragraph in JSX</p> </div> ); }
The similarity between HTML, XML, and JSX is evident. JSX uniquely integrates HTML within JavaScript, hence its name: JavaScript XML.
This fusion of HTML and JavaScript enables dynamic HTML manipulation. For instance, a button click could toggle a class name.
React Components
React facilitates the definition of custom components that return JSX. Essentially, a React component is a JavaScript function generating JSX.
Upon rendering a root React component, JSX is automatically converted into HTML and integrated into the webpage. React allows creating custom components (akin to custom tags) for inclusion within other components.
Here's a component utilizing the ExampleReactComponent
:
function RootReactComponent() { return ( <div> <h1>This is the main Component</h1> <ExampleReactComponent /> </div> ); }
Props
Similar to HTML attributes, React components employ props.
function PropsExample(props) { console.log(props); // Prints { exampleValue: "example-value" } return ( <div> <h1>Props Example</h1> {props.exampleValue} </div> ); } function MainComponent() { return ( <div> <h1>This is the Main Component</h1> <PropsExample exampleValue={"example-value"} /> </div> ); }
Rendering MainComponent
invokes PropsExample
with { exampleValue: "example-value" }
as the props
argument. Note that JavaScript variables are embedded within JSX using {}
.
State
Data is typically stored in variables for display. React utilizes "state" for data management, acting as a getter/setter mechanism. When state is modified, React automatically updates the component's HTML.
This example demonstrates automatic HTML updates upon state changes:
import { useState } from 'react'; function IncrementDecrement() { const [value, setValue] = useState(0); // Initial value: 0 function increment(e) { setValue(value + 1); } function decrement(e) { setValue(value - 1); } return ( <div> <button onClick={increment}>Increment</button> <button onClick={decrement}>Decrement</button> {value} </div> ); }
Building React Apps with Vite
Tools like Vite streamline the bundling of React components and JSX into browser-executable JavaScript.
To initiate a React project:
npm create vite@latest my-react-app -- --template react cd my-react-app npm install npm run dev
Conclusion
This introduction to React.js provides a foundational understanding. After setting up a Vite project, experiment with creating React components to deepen your comprehension. Further learning resources are available at https://www.php.cn/link/27a5eaafdb88c45dd61732d6a6493421.
The above is the detailed content of A Quick Intro to React.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
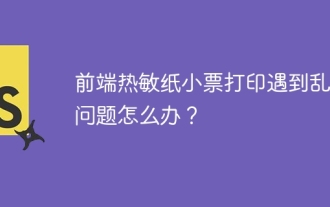
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
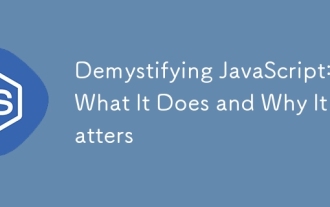
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
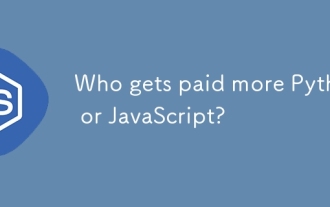
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
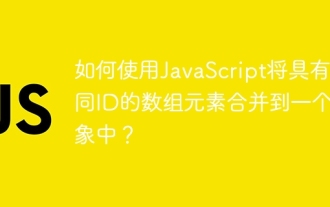
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
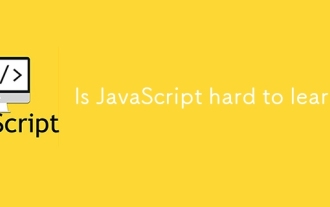
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
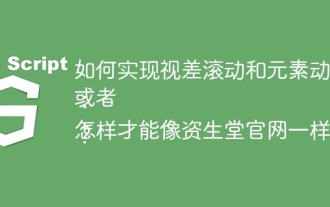
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
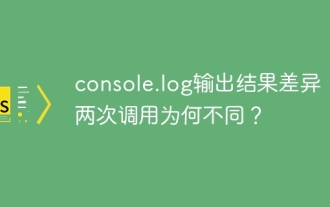
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
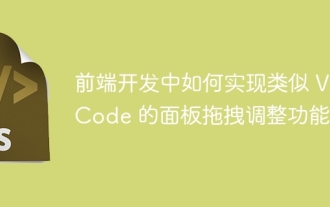
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
