


Mastering Custom Profiling in Go: Boost Performance with Advanced Techniques
Explore my Amazon books and follow my Medium for more insights. Your support is invaluable!
I've extensively researched and implemented custom profiling in Go, a powerful technique for significantly enhancing application performance and resource efficiency. Let's delve into my findings.
Profiling is crucial for understanding application behavior in real-world scenarios. While Go's built-in tools are excellent, custom profiling offers tailored analysis for deeper insights into performance characteristics.
To begin, define the metrics to track – function execution times, memory allocations, goroutine counts, or application-specific data.
Here's a basic custom function profiling example:
package main import ( "fmt" "sync" "time" ) type FunctionProfile struct { Name string CallCount int TotalTime time.Duration } var profiles = make(map[string]*FunctionProfile) var profileMutex sync.Mutex func profileFunction(name string) func() { start := time.Now() return func() { duration := time.Since(start) profileMutex.Lock() defer profileMutex.Unlock() if p, exists := profiles[name]; exists { p.CallCount++ p.TotalTime += duration } else { profiles[name] = &FunctionProfile{ Name: name, CallCount: 1, TotalTime: duration, } } } } func expensiveOperation() { defer profileFunction("expensiveOperation")() time.Sleep(100 * time.Millisecond) } func main() { for i := 0; i < 10; i++ { expensiveOperation() } profileMutex.Lock() defer profileMutex.Unlock() for name, p := range profiles { fmt.Printf("Function: %s, Call Count: %d, Total Time: %s\n", name, p.CallCount, p.TotalTime) } }
This example tracks function execution times and call counts. profileFunction
is a higher-order function returning a deferred function for accurate duration measurement.
Real-world applications often need more sophisticated techniques, tracking memory allocations, goroutine counts, or custom metrics. Let's expand the example:
package main import ( "fmt" "runtime" "sync" "time" ) // ... (rest of the code remains similar, with additions for memory and goroutine tracking)
This enhanced version adds memory usage and goroutine count tracking using a background goroutine for periodic updates.
Remember, custom profiling introduces overhead. Balance detail with performance impact. For production, consider dynamic enabling/disabling or sampling to reduce overhead. Here's a sampling example:
package main import ( "fmt" "math/rand" "runtime" "sync" "time" ) // ... (rest of the code includes sampling logic)
This advanced system allows dynamic control, sampling for reduced overhead, and improved concurrent safety.
Data analysis and visualization are crucial. Consider integrating with tools like Grafana or creating custom dashboards. Here's a basic HTTP endpoint example:
package main import ( "encoding/json" "fmt" "net/http" "runtime" "sync" "time" ) // ... (rest of the code, including HTTP handler for exposing profiling data)
This provides a JSON endpoint for accessing profiling data, easily integrated with visualization tools.
Custom profiling in Go provides powerful performance insights. Combine it with Go's built-in tools for comprehensive monitoring. Regularly review data, identify patterns, and use insights to optimize. Custom profiling is an invaluable asset in your Go development toolkit.
101 Books
101 Books, an AI-powered publisher co-founded by Aarav Joshi, offers affordable quality knowledge through low publishing costs (some books as low as $4). Explore our "Golang Clean Code" book on Amazon. Search for "Aarav Joshi" for more titles and special discounts!
Our Creations
Investor Central | Investor Central Spanish | Investor Central German | Smart Living | Epochs & Echoes | Puzzling Mysteries | Hindutva | Elite Dev | JS Schools
We are on Medium
Tech Koala Insights | Epochs & Echoes World | Investor Central Medium | Puzzling Mysteries Medium | Science & Epochs Medium | Modern Hindutva
The above is the detailed content of Mastering Custom Profiling in Go: Boost Performance with Advanced Techniques. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










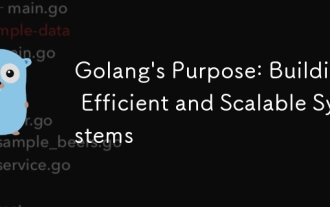
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
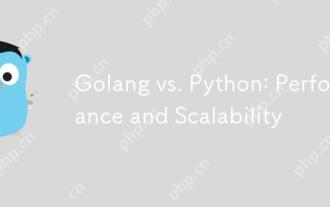
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
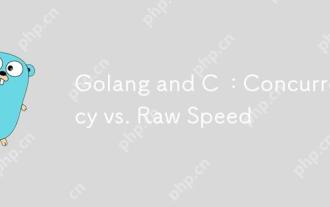
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
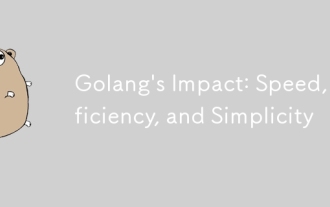
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
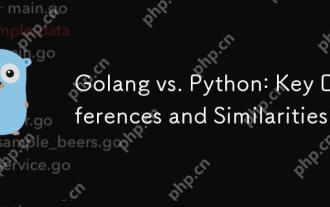
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
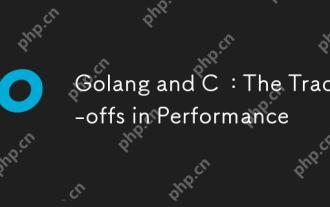
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
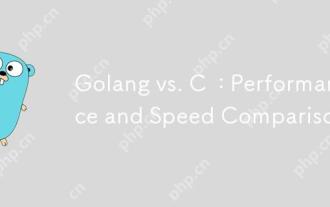
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
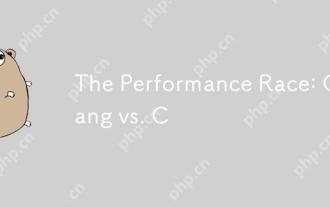
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
