


Why You Should Use a Single FastAPI App and TestClient Instance
In FastAPI development, particularly for larger projects, employing a single FastAPI application instance and a single TestClient instance throughout your project is crucial for maintaining consistency, optimizing performance, and ensuring reliability. Let's examine the reasons behind this best practice and explore practical examples.
1. Application-Wide Consistency
Creating multiple FastAPI app instances can introduce inconsistencies. Each instance possesses its own internal state, middleware configuration, and dependency management. Sharing stateful data, such as in-memory storage or database connections, across multiple instances can lead to unpredictable behavior and errors.
2. Enhanced Performance
Each TestClient instance establishes its own HTTP connection and initializes dependencies. Utilizing a single TestClient minimizes overhead, resulting in faster test execution.
3. Preventing Initialization Problems
FastAPI applications often initialize resources, including database connections or background tasks, during startup. Multiple instances can cause redundant initializations or resource conflicts.
Hands-On Code Example
Correct Approach: Single App and TestClient
from fastapi import FastAPI, Depends from fastapi.testclient import TestClient # Single FastAPI app instance app = FastAPI() # Simple in-memory database database = {"items": []} # Dependency function def get_database(): return database @app.post("/items/") def create_item(item: str, db: dict = Depends(get_database)): db["items"].append(item) return {"message": f"Item '{item}' added."} @app.get("/items/") def list_items(db: dict = Depends(get_database)): return {"items": db["items"]} # Single TestClient instance client = TestClient(app) # Test functions def test_create_item(): response = client.post("/items/", json={"item": "foo"}) assert response.status_code == 200 assert response.json() == {"message": "Item 'foo' added."} def test_list_items(): response = client.get("/items/") assert response.status_code == 200 assert response.json() == {"items": ["foo"]}
Incorrect Approach: Multiple Instances
# Incorrect: Multiple app instances app1 = FastAPI() app2 = FastAPI() # Incorrect: Multiple TestClient instances client1 = TestClient(app1) client2 = TestClient(app2) # Problem: State changes in client1 won't affect client2
Common Problems with Multiple Instances
- Inconsistent State: Shared state (like a database) behaves independently across different app instances.
- Redundant Dependency Initialization: Dependencies such as database connections might be initialized multiple times, potentially leading to resource depletion.
- Overlapping Startup/Shutdown Events: Multiple app instances trigger startup and shutdown events independently, causing unnecessary or conflicting behavior.
Best Practices
Project Structure for Reusability
Create your FastAPI app in a separate file (e.g., app.py
) and import it where needed.
# app.py from fastapi import FastAPI app = FastAPI() # Add your routes here
# main.py from fastapi.testclient import TestClient from app import app client = TestClient(app)
Leveraging pytest Fixtures for Shared Instances
pytest fixtures effectively manage shared resources, such as the TestClient:
import pytest from fastapi.testclient import TestClient from app import app @pytest.fixture(scope="module") def test_client(): client = TestClient(app) yield client # Ensures proper cleanup
def test_example(test_client): response = test_client.get("/items/") assert response.status_code == 200
Relevant Documentation
- Starlette TestClient
- Testing with FastAPI
- pytest Fixtures
By adhering to these guidelines, your FastAPI project will be more consistent, efficient, and easier to maintain.
Photo by Shawon Dutta: https://www.php.cn/link/e2d083a5fd066b082d93042169313e21
The above is the detailed content of Why You Should Use a Single FastAPI App and TestClient Instance. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










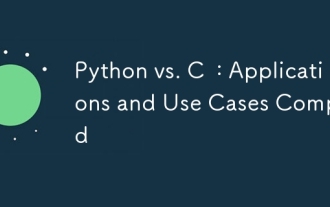
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
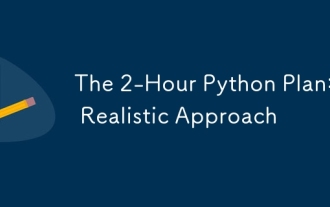
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
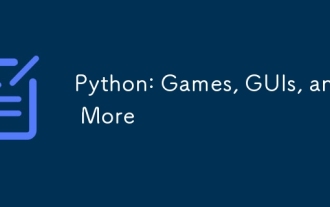
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
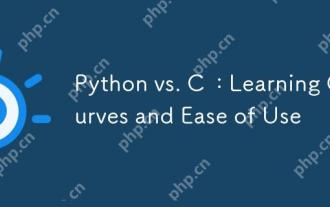
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
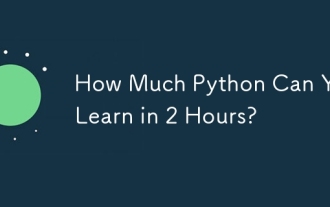
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
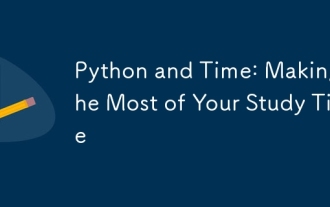
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
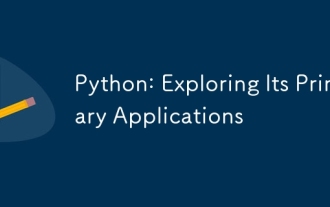
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
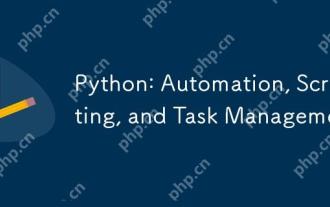
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
