Unit Testing in Python
Python unit testing is a software testing method that tests individual units or components of code individually to ensure that they work as expected. These building blocks can be functions, classes, or methods.
Importance of unit testing
Unit testing is crucial when:
Catch bugs early: Unit testing allows you to catch bugs early in development, making them easier and more cost-effective to fix.
Improving code quality: Writing tests encourages you to consider edge cases and potential problems, resulting in well-structured code.
Facilitate refactoring: Unit tests allow you to refactor at scale without worrying about breaking functionality.
Documentation: Unit tests act as dynamic documents, demonstrating how the code is used.
How to do unit testing in Python?
Here’s how to do unit testing in Python:
Using the unittest module: Python provides a built-in module called unittest for writing unit tests.
Create a test case: A test case is a class that is a subclass of unittest.TestCase. In this class, you can define methods to test specific functionality of your code.
Using assertions: The UnitTest module contains built-in assertions for verifying that the actual output matches the expected output.
Running tests: Tests can be executed using the UnitTest command line interface or by running the test file directly.
Example
The following example illustrates how to use unit tests in your code:
import unittest def add(x, y): return x + y class TestAddFunction(unittest.TestCase): def test_add_positive_numbers(self): result = add(2, 3) self.assertEqual(result, 5) def test_add_negative_numbers(self): result = add(-2, -3) self.assertEqual(result, -5) if __name__ == '__main__': unittest.main()
Results
<code>---------------------------------------------------------------------- Ran 0 tests in 0.000s OK</code>
Unit testing framework in Python
The PyUnit framework (sometimes called the unit testing framework) is Python’s standard library module for unit testing. It provides a wide range of tools to create and execute tests, automate the testing process, and detect software issues early in the development cycle. Unit testing supports test automation, shared test setup and shutdown code, grouping of tests into collections, and independence of testing from reporting frameworks.
Click here to read the full tutorial
The above is the detailed content of Unit Testing in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










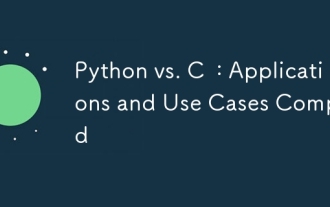
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
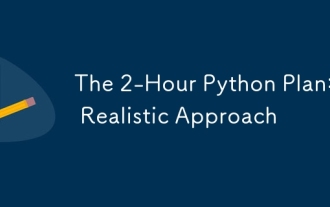
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
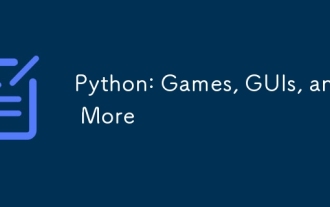
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
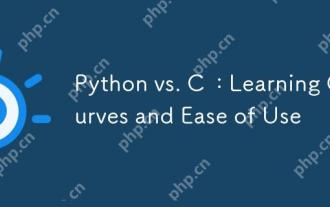
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
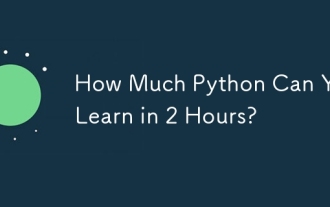
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
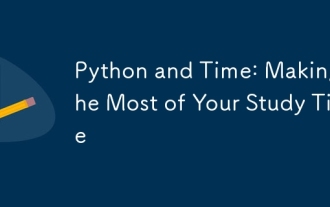
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
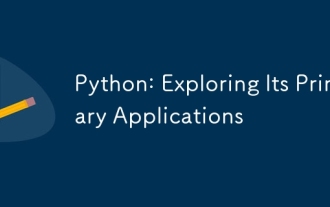
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
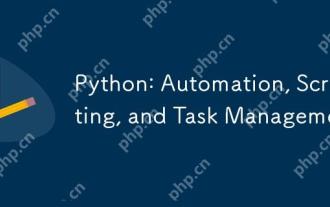
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
