Arrays in JavaScript
Detailed explanation of core JavaScript array methods
The following are some commonly used JavaScript array methods:
-
push()
: Adds one or more elements to the end of the array and returns the length of the new array.let numbers = [1, 2, 3]; console.log(numbers.push(4)); // 输出:4 numbers数组变为 [1, 2, 3, 4]
Copy after login -
slice()
: Extract elements within the specified index range from the array and return a new array. The original array is not changed.let numbers = [1, 2, 3, 4, 5]; console.log(numbers.slice(1, 4)); // 输出:[2, 3, 4] (从索引1到3)
Copy after login -
splice()
: Add or remove elements from the array. You can specify the starting index, the number of elements to delete, and the new elements to insert.let fruits = ['apple', 'mango', 'orange', 'pear']; fruits.splice(2, 0, 'pawpaw', 'strawberries'); // 从索引2开始,不删除任何元素,插入'pawpaw'和'strawberries' console.log(fruits); // 输出:['apple', 'mango', 'pawpaw', 'strawberries', 'orange', 'pear'] fruits.splice(2, 1); // 从索引2开始,删除1个元素 console.log(fruits); // 输出:['apple', 'mango', 'strawberries', 'orange', 'pear']
Copy after login -
concat()
: Concatenates two or more arrays and returns a new array. The original array is not changed.let arr1 = [1, 2, 3, 4]; let arr2 = [5, 6, 7]; console.log(arr1.concat(arr2)); // 输出:[1, 2, 3, 4, 5, 6, 7] let arr3 = [8, 9]; console.log(arr1.concat(arr2, arr3)); // 输出:[1, 2, 3, 4, 5, 6, 7, 8, 9]
Copy after login -
fill()
: Replaces elements in the specified range in the array with the specified value.let arr4 = [1, 2, 3, 4]; arr4.fill('Anurag', 2, 4); // 从索引2到3,用'Anurag'填充 console.log(arr4); // 输出:[1, 2, 'Anurag', 'Anurag']
Copy after login -
shift()
: Delete the first element of the array and return that element.let arr4 = [1, 2, 3, 4]; arr4.shift(); console.log(arr4); // 输出:[2, 3, 4]
Copy after login -
indexOf()
: Returns the index of the first occurrence of the specified element in the array. If the element does not exist, returns -1.let arr4 = [1, 2, 3, 4]; console.log(arr4.indexOf(3)); // 输出:2
Copy after login -
lastIndexOf()
: Returns the index of the last occurrence of the specified element in the array. If the element does not exist, returns -1.let arr4 = [1, 2, 3, 4, 3]; console.log(arr4.lastIndexOf(3)); // 输出:4
Copy after login -
includes()
: Determines whether the array contains the specified element and returns a Boolean value.let arr4 = [1, 2, 3, 4]; console.log(arr4.includes(4)); // 输出:true console.log(arr4.includes(5)); // 输出:false
Copy after login -
pop()
: Delete the last element of the array and return that element.let arr4 = [1, 2, 3, 4]; arr4.pop(); console.log(arr4); // 输出:[1, 2, 3]
Copy after login -
join()
: Concatenates array elements into a string and returns the string. Delimiters can be specified.let arr4 = [1, 2, 3]; console.log(arr4.join('and')); // 输出:1and2and3
Copy after login -
unshift()
: Adds one or more elements to the beginning of the array and returns the length of the new array.let arr4 = [1, 2, 3]; arr4.unshift(0); console.log(arr4); // 输出:[0, 1, 2, 3]
Copy after login
My GitHub link
My LinkedIn link (please replace with your actual link)
The above is the detailed content of Arrays in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










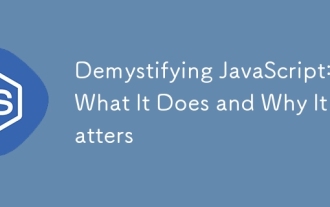
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
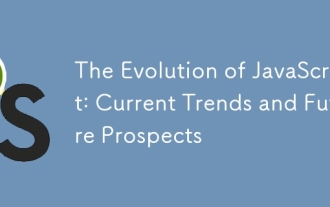
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
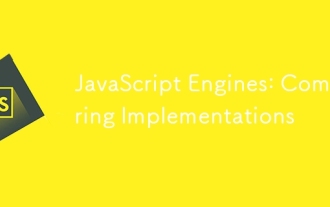
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
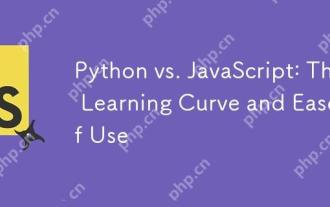
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
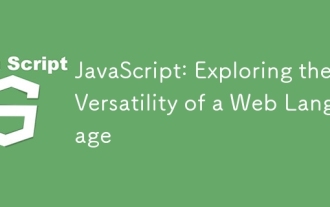
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
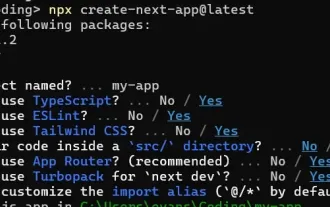
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
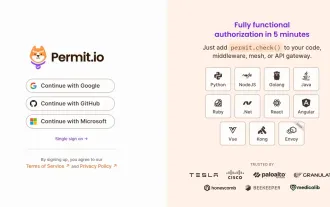
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
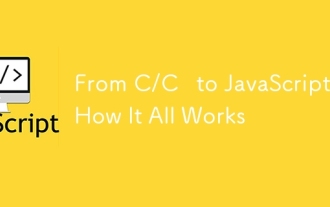
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
