How Do Dynamic Variables Impact C# Performance?
C# Dynamic Variables: Performance Implications
Dynamic Variable Behavior and Performance
Using dynamic variables in C# introduces specific performance challenges. During compilation, the compiler must perform extra work to determine the variable's type and appropriate actions. This contrasts with statically-typed variables where type information is known at compile time.
The Dynamic Invocation Process
Each dynamic expression generates a dynamic call site object. While runtime caching of these call sites optimizes repeated invocations, the initial overhead remains.
Type Determination and Optimization
The Dynamic Language Runtime (DLR) identifies the type of dynamic objects. For specialized types (like COM objects or IronPython objects), optimizations are possible. However, with standard C# objects, the DLR necessitates a re-compilation step.
Re-compilation Overhead
This re-compilation uses a lightweight C# compiler to generate an Expression Tree representing the dynamic call. This tree is then compiled into an executable delegate and cached. The significant performance cost arises from this re-compilation process.
Caching Effects on Performance
The impact of dynamic variables depends heavily on caching. Repeated use of the same dynamic expression benefits from the cached delegate, minimizing the overhead. Conversely, multiple dynamic operations within a single expression create multiple call sites and caches, increasing the performance penalty.
Illustrative Example: A Timed Loop
The accompanying code example (not shown here, but referenced in the original text) demonstrates this performance difference. A Sum()
method (baseline) iterates without dynamic operations. SumInt()
uses a class with integer properties. DynamicSum()
introduces a dynamic property, showcasing a substantial performance drop (approximately two orders of magnitude). This clearly illustrates the re-compilation overhead for dynamic operations within loops.
The above is the detailed content of How Do Dynamic Variables Impact C# Performance?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










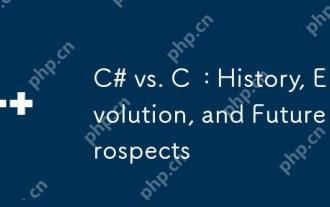
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
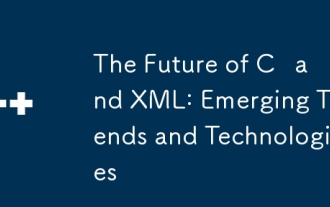
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
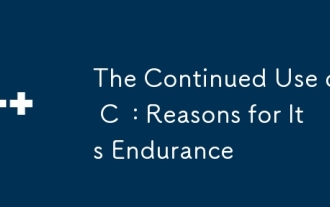
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
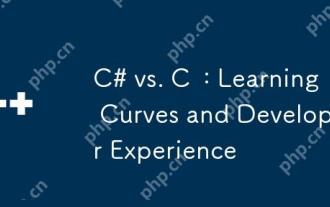
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
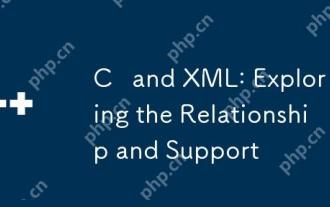
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
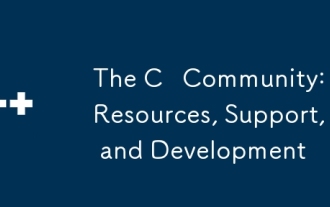
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
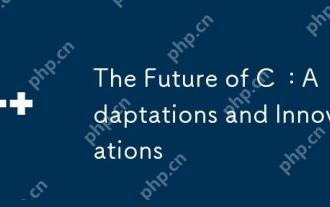
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
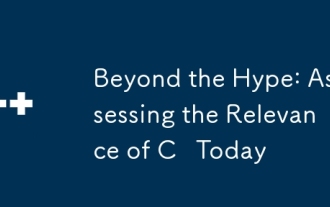
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
