This is How All Good React Developers Structure Their State.
Poorly structured React component state is a breeding ground for errors, bugs, and difficult debugging. Well-structured state, however, results in cleaner, more maintainable, and easier-to-debug components. Let's explore key principles for effective state management.
Fundamentals of Data Structuring
React components often rely on state. The number of state variables and the properties within object states are developer choices. Even with less-than-ideal structuring, a component might function, but we can strive for better. These guidelines will help you make informed decisions about your component's state structure:
1. Merge Interconnected States: Frequently updated states should be grouped. While separating states is acceptable, merging interconnected states significantly reduces the risk of synchronization errors. For example, consider aspect ratio:
// Less efficient: const [height, setHeight] = useState(500); const [width, setWidth] = useState(500); // More efficient: const [aspectRatio, setAspectRatio] = useState({ width: 500, height: 500 });
Remember, when merging into an object, update all properties using the spread operator to avoid losing data:
setAspectRatio({ ...aspectRatio, width: 750 }); // Correct // setAspectRatio({ width: 750 }); // Incorrect
2. Avoid State Conflicts: Consider a submit button. You might use state to indicate loading, error, and success. The following structure is problematic:
// Problematic structure: const [loading, setLoading] = useState(false); const [error, setError] = useState(false); const [resolved, setResolved] = useState(true);
This setup allows for contradictory states (e.g., loading and resolved simultaneously). A better approach uses constants to represent states:
// Improved structure: const STATE = { loading: 'loading', error: 'error', resolved: 'resolved', }; const [requestState, setRequestState] = useState(STATE.resolved);
This prevents conflicting states and makes error tracking easier.
3. Avoid Unnecessary State: Before creating state, check if the value can be derived from props, existing state, or state combinations during rendering. If derivable, avoid adding it as component state.
// Inefficient: const [x, setX] = useState(0); const [y, setY] = useState(0); const [position, setPosition] = useState({ x: 0, y: 0 });
Here, position
is redundant. Either remove position
or eliminate x
and y
.
4. Eliminate State Duplication: Duplicated state is difficult to keep synchronized. If you find yourself using multiple states to maintain the same data, consolidate them, potentially raising the state to a higher component level.
5. Minimize State Nesting: Deeply nested state is hard to update. "Flatten" or "normalize" it. Instead of nested objects, consider using arrays of IDs and a separate mapping from IDs to data. The depth of nesting is a trade-off, but flatter state simplifies updates and reduces duplication risks. Libraries like Immer can assist with managing immutable nested state.
If you have any questions, please leave a comment.
The above is the detailed content of This is How All Good React Developers Structure Their State.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










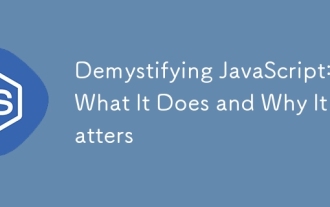
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
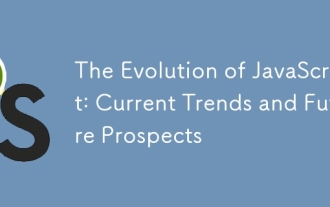
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
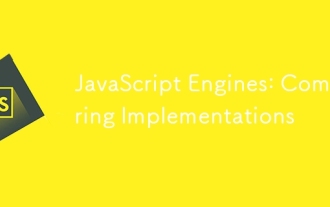
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
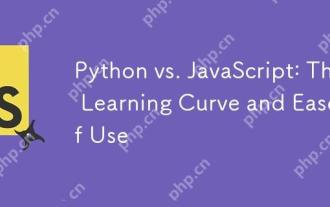
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
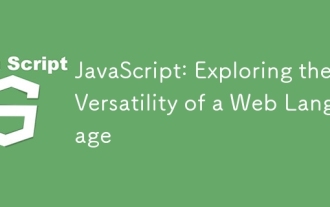
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
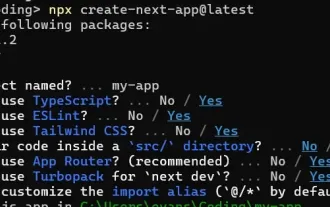
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
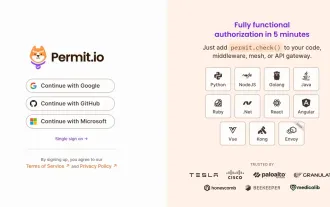
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
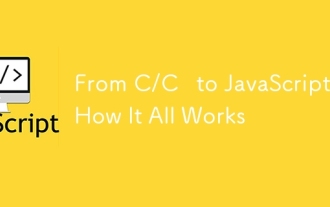
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
