


Why Does C# Throw a 'Cannot Modify Return Value' Error When Modifying Auto-Implemented Properties?
Jan 19, 2025 am 09:47 AMDetailed explanation of "Cannot modify return value" error in C#
Auto-implemented properties in C# provide a convenient way to define properties, but sometimes when trying to modify the property value, it may result in a "Cannot modify return value" error.
Please see the following code:
public Point Origin { get; set; } Origin.X = 10; // 产生 CS1612 错误
This code attempts to set the X coordinate of the Origin property. However, it will report an error saying that the return value of the property cannot be modified because it is not a variable.
Root cause: value types and reference types
This error occurs because Point is a value type (structure). Value types are copied by value, which means that when you assign a value type to a variable, a new copy is created. In this case, when you access the Origin property, you are actually accessing a copy of the Point value, not the original value stored in the class.
Solution
To resolve this issue, you have two options:
- Use reference types: Change Point to a reference type (class) instead of a value type. Reference types are copied by reference, which means that when you assign a reference type to a variable, the pointer to the original object is copied. This allows the original object to be modified directly via its properties.
- Stored in a temporary variable: If you want to keep Point as a value type, you need to store the result of the property access in a temporary variable before modifying it. For example:
Point temp = Origin; temp.X = 10; Origin = temp;
By storing the property value in the temp variable, you create a modifiable copy of the value type. You can then set properties to the modified copy.
The above is the detailed content of Why Does C# Throw a 'Cannot Modify Return Value' Error When Modifying Auto-Implemented Properties?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
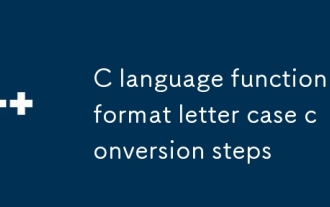
C language function format letter case conversion steps
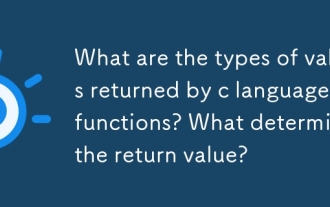
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
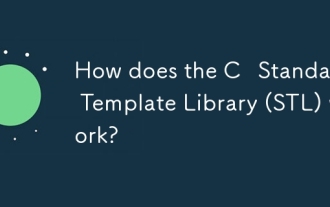
How does the C Standard Template Library (STL) work?

Where is the return value of the c language function stored in memory?
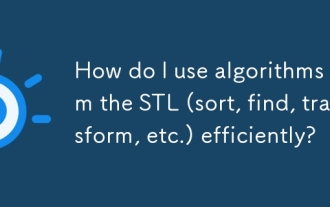
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
