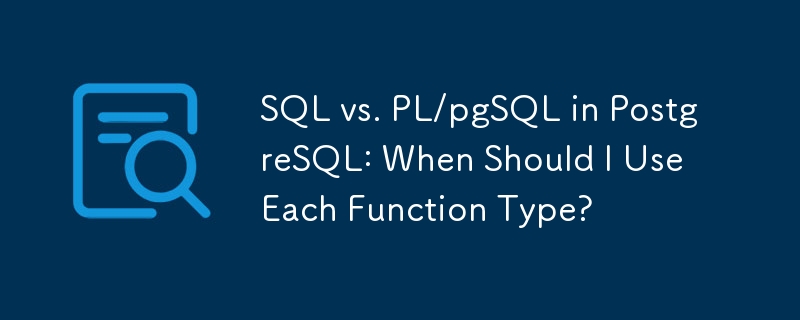
PostgreSQL Functions: Best Practices for SQL and PL/pgSQL
Functions in a PostgreSQL database can be implemented using SQL or PL/pgSQL (Procedural Language/PostgreSQL). Although both techniques can achieve similar results, which method to choose depends on the specific requirements of the problem.
SQL Function
Advantages:
- Simple and direct when used in scalar queries
- Minimum overhead when called occasionally
- Since there is no block structure, the code is concise
Usage scenarios:
- Simple query without procedural elements or variables
- Single or infrequent call
- Execute inline within SQL query
- Easy to use for users who are familiar with SQL but not familiar with PL/pgSQL
PL/pgSQL functions
Advantages:
- Supports procedural logic, variables and dynamic SQL
- Reuse calculation results in multiple executions
- Improving performance with query plan caching
- Catch errors and handle exceptions
- Trigger function
- Use DDL statements to modify database objects
Usage scenarios:
- Computational tasks that cannot be expressed in SQL alone
- Dynamic SQL generation and execution
- Complex processes or error handling
- Optimize repeated calls through query plan caching
- Synchronous execution of database modifications
Choice between SQL and PL/pgSQL
To make an informed decision, consider the following factors:
-
Simplicity: SQL functions are simpler to write and understand.
-
Performance: PL/pgSQL functions may perform better after the first call due to plan caching.
-
Flexibility: PL/pgSQL provides greater functionality through procedural elements and dynamic SQL.
-
Specific Requirements: Consider the specific needs of your application, such as variable manipulation, error handling, or database modifications.
Example of function implementation
Use the example provided in the query:
<code class="language-sql">CREATE OR REPLACE FUNCTION f1(istr varchar)
RETURNS text AS $$
SELECT 'hello! '::varchar || istr;
$$ LANGUAGE SQL;</code>
Copy after login
<code class="language-sql">CREATE OR REPLACE FUNCTION f2(istr varchar)
RETURNS text AS $$
BEGIN
RETURN 'hello! '; -- 类型默认为 text
END
$$ LANGUAGE plpgsql;</code>
Copy after login
In this example, the two functions return the same result for the input value "world", but the underlying implementation is different. f1 uses a simple SQL query, while f2 uses PL/pgSQL's BEGIN-END block and implicit returns.
The above is the detailed content of SQL vs. PL/pgSQL in PostgreSQL: When Should I Use Each Function Type?. For more information, please follow other related articles on the PHP Chinese website!