Exploring Promises in JavaScript
Mastering asynchronous JavaScript often involves understanding Promises. While initially daunting, Promises become invaluable tools once grasped. This guide clarifies what Promises are, their functionality, and their significance.
Understanding JavaScript Promises
A Promise is a JavaScript object representing the eventual success or failure of an asynchronous operation. Essentially, it manages operations that don't return immediate results, such as API data retrieval or file reading.
Promises exist in three states:
- Pending: The operation is in progress.
- Fulfilled: The operation succeeded.
- Rejected: The operation failed.
Once fulfilled or rejected, a Promise's state is fixed.
The Necessity of Promises
JavaScript's single-threaded nature means it handles one operation at a time. Asynchronous operations prevent main thread blocking. Before Promises, callbacks were the standard, but nested callbacks resulted in complex, hard-to-maintain code. Promises offer a cleaner, more readable alternative for managing asynchronous tasks.
Promise Anatomy
Promise creation uses the Promise
constructor, accepting an executor function with resolve
and reject
arguments:
1 2 3 4 5 6 7 8 9 |
|
resolve
: Called on successful operation completion.reject
: Called on operation failure.
Utilizing a Promise
.then()
, .catch()
, and .finally()
handle Promise outcomes:
1 2 3 4 5 6 7 8 9 10 |
|
.then()
: Executes on fulfillment..catch()
: Executes on rejection..finally()
: Executes regardless of outcome.
Real-World Application: Data Fetching
Promises are frequently used with APIs. Here's a fetch
API example:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
This example shows:
fetch
returning a Promise.- The first
.then()
parsing the response. - The second
.then()
processing parsed data. .catch()
handling errors.
Advanced Techniques: Promise Chaining
Promise chaining is a key advantage. Each .then()
returns a new Promise, enabling sequential asynchronous operation execution:
1 2 3 4 |
|
This maintains code clarity and avoids deeply nested callbacks.
Async/Await: Simplified Syntax
ES2017's async/await
simplifies Promise handling, making asynchronous code appear synchronous:
1 2 3 4 5 6 7 8 9 |
|
async/await
builds upon Promises; understanding Promises is essential for effective async/await
use.
Key Advantages of Promises
- Readability: Improved readability and maintainability of asynchronous code.
-
Error Handling: Centralized error handling via
.catch()
. - Chaining: Enables sequential asynchronous operation execution.
Common Mistakes
- Missing Promise Returns: Always return a Promise during chaining.
-
Unhandled Rejections: Use
.catch()
ortry-catch
for error handling. - Mixing Callbacks and Promises: Maintain consistency in approach.
Conclusion
Promises are a powerful JavaScript feature for simplifying asynchronous operation handling. Understanding their structure and usage leads to cleaner, more maintainable code. Refer back to this guide for future Promise refreshers! Share your questions and examples in the comments below!
The above is the detailed content of Exploring Promises in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
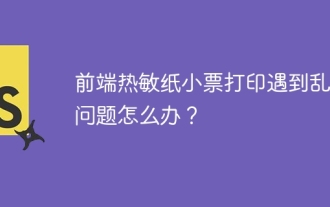
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
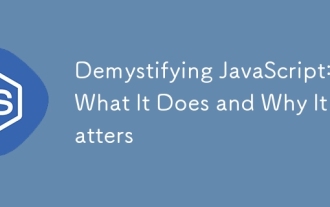
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
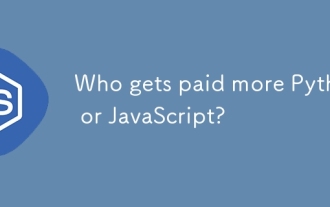
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
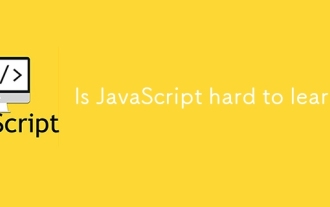
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
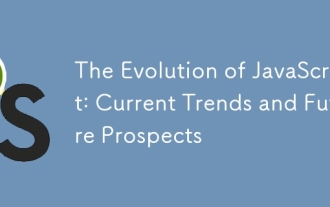
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
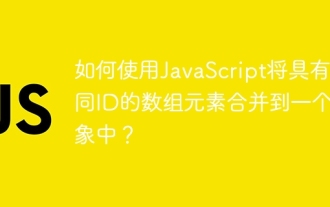
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
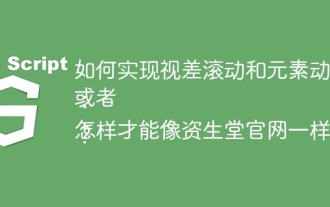
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
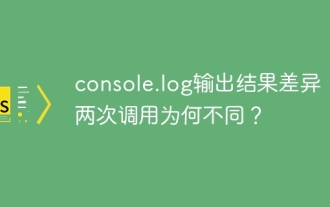
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
