


How Can I Retrieve Data with Dynamic Column Names Using Prepared Statements in MySQL and Java?
Dynamic Column Name Retrieval in MySQL and Java Using Prepared Statements: A Safer Approach
Many MySQL and Java developers have explored using prepared statements to retrieve data with dynamically generated column names. However, directly substituting column names into a prepared statement's parameters is not supported and introduces significant security risks. A database schema redesign is usually the best solution.
Attempting to use prepared statements in this way, as shown below, results in an error:
String columnNames = "d,e,f"; String query = "SELECT a,b,c,?" + " FROM " + name + " WHERE d=?"; // This results in "SELECT a,b,c,'d,e,f' FROM some_table WHERE d='x'", not the desired result.
The query treats the ?
placeholder as a string literal, not a column name.
To avoid SQL injection vulnerabilities, a more robust method involves storing the dynamic column names within the database itself. Consider a table like "user_data" with these columns:
id
(primary key)user_name
column_names
(a comma-separated string of column names)
The SQL query can then be constructed by concatenating the column_names
retrieved from this table:
String query = "SELECT a,b,c," + columnNames + " FROM " + name + " WHERE d=?";
This approach ensures that the column names are not treated as parameters susceptible to injection attacks. While it uses string concatenation, the vulnerable part (the column names) is already sanitized by being retrieved from the database. Remember, always sanitize any user-supplied data that's used in query construction, even if it's not directly part of the SQL statement. This revised approach offers a safer and more manageable solution for handling dynamic column names.
The above is the detailed content of How Can I Retrieve Data with Dynamic Column Names Using Prepared Statements in MySQL and Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










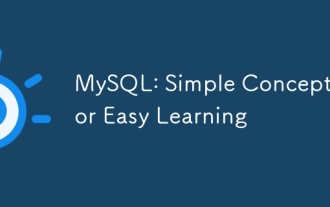
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
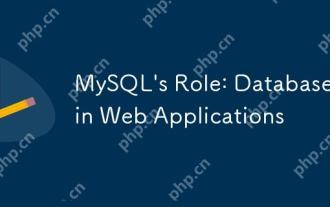
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
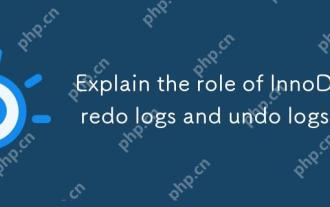
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
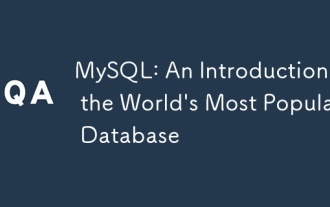
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
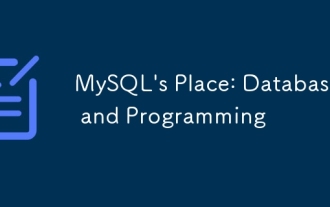
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
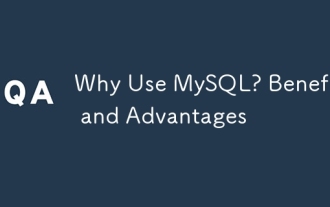
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
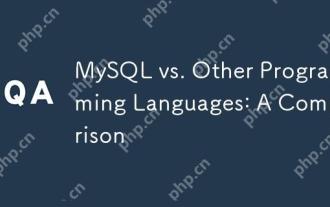
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
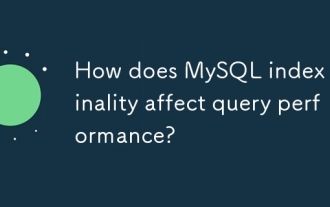
MySQL index cardinality has a significant impact on query performance: 1. High cardinality index can more effectively narrow the data range and improve query efficiency; 2. Low cardinality index may lead to full table scanning and reduce query performance; 3. In joint index, high cardinality sequences should be placed in front to optimize query.
