How to Make HTTP GET and POST Requests in Unity using C#?
Using C# to make HTTP requests in Unity
When developing games or interactive applications in Unity, the ability to send HTTP requests is critical for tasks such as user authentication, getting data from web services, and submitting game events. This article demonstrates how to send HTTP GET and POST requests using C# in Unity to meet the requirements specified in the question.
UnityWebRequest: Request and response handling
UnityWebRequest provides a convenient and efficient way to make web requests in Unity. It handles coroutines and multi-threading internally, makes asynchronous requests and prevents UI freezing.
GET request
To send a GET request, just call UnityWebRequest.Get() and pass in the URI. The response text can be accessed via uwr.downloadHandler.text.
IEnumerator getRequest(string uri) { UnityWebRequest uwr = UnityWebRequest.Get(uri); yield return uwr.SendWebRequest(); if (uwr.isNetworkError) Debug.Log("发送错误: " + uwr.error); else Debug.Log("接收: " + uwr.downloadHandler.text); }
POST request containing form data
To send a POST request containing form data, build the form using WWWForm and pass it to UnityWebRequest.Post().
IEnumerator postRequest(string url) { WWWForm form = new WWWForm(); form.AddField("myField", "myData"); form.AddField("Game Name", "Mario Kart"); UnityWebRequest uwr = UnityWebRequest.Post(url, form); yield return uwr.SendWebRequest(); if (uwr.isNetworkError) Debug.Log("发送错误: " + uwr.error); else Debug.Log("接收: " + uwr.downloadHandler.text); }
JSON POST request
For JSON POST requests, create a raw upload handler and manually set the Content-Type header to application/json.
IEnumerator postRequest(string url, string json) { var uwr = new UnityWebRequest(url, "POST"); byte[] jsonToSend = new System.Text.UTF8Encoding().GetBytes(json); uwr.uploadHandler = (UploadHandler)new UploadHandlerRaw(jsonToSend); uwr.downloadHandler = (DownloadHandler)new DownloadHandlerBuffer(); uwr.SetRequestHeader("Content-Type", "application/json"); yield return uwr.SendWebRequest(); if (uwr.isNetworkError) Debug.Log("发送错误: " + uwr.error); else Debug.Log("接收: " + uwr.downloadHandler.text); }
PUT, DELETE and Multipart/Form-Data
UnityWebRequest also supports PUT, DELETE and multipart/form-data requests. See the provided code snippet for a detailed example.
By following these code examples, you can quickly send and handle HTTP requests in your Unity game or application, giving you powerful tools for seamless data exchange.
The above is the detailed content of How to Make HTTP GET and POST Requests in Unity using C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


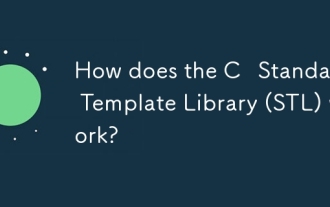
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
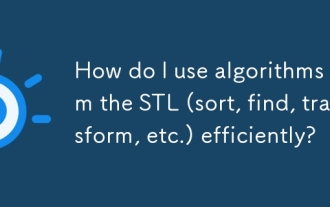
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
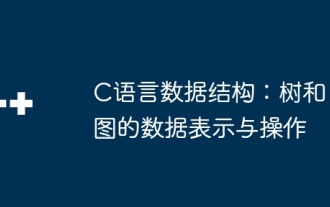
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
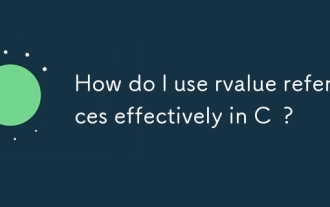
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
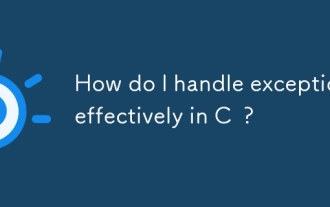
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
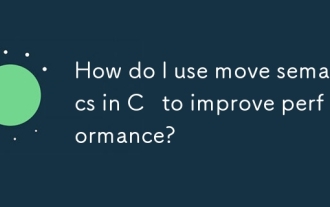
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
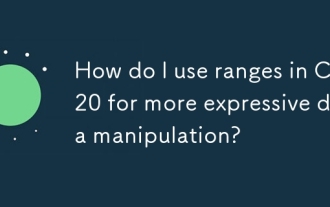
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
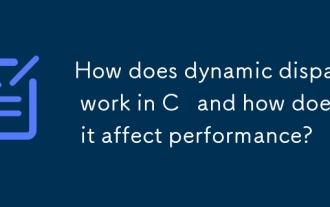
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
