How to Deserialize Nested JSON into C# Classes?
Deserialize JSON into nested C# classes
The JSON response retrieved from the REST API contains multiple job code entries nested in a complex structure. Deserializing this data requires a class structure that matches the JSON layout and an appropriate data type to handle the different keys.
First, create a root-level class RootObject
that contains the properties Results
of another class Results
. Results
will contain a JobCodes
named Dictionary<string, JobCode>
, where the string keys are job code identifiers ("1", "2", etc.) and the values are JobCode
objects.
Next, define the JobCode
class whose properties map to JSON values: StatusCode
, StatusMessage
, Id
, and Name
.
To deserialize JSON, use:
RootObject obj = JsonConvert.DeserializeObject<RootObject>(json);
This will create an instance of RootObject
which you can then access to retrieve a list of job codes like this:
List<JobCode> jobCodes = obj.Results.JobCodes.Values.ToList();
The above is the detailed content of How to Deserialize Nested JSON into C# Classes?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
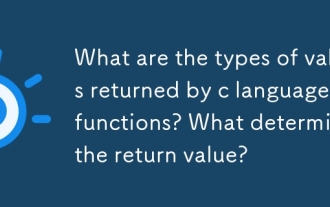
What are the types of values returned by c language functions? What determines the return value?
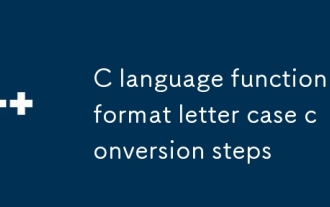
C language function format letter case conversion steps

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
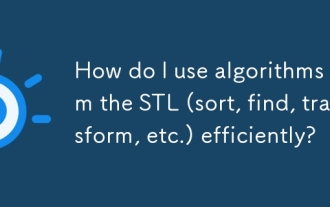
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
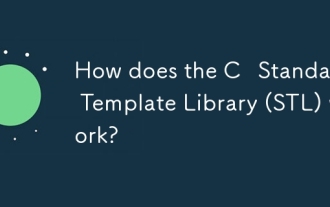
How does the C Standard Template Library (STL) work?
