How to Effectively Mock Extension Methods Using Moq?
Mocking extension methods with Moq: A complete guide
Testing code that relies on extension methods can pose unique challenges, especially if you want to ensure that future failures of these extension methods do not impact your tests. While Moq does not directly support overriding static methods (such as those used in extension methods), there is a clever workaround to achieve mocking in this situation.
Unlike instance methods, static methods cannot be overridden or hidden. Moq's primary functionality is to create mock instances of objects, which essentially excludes targeting static methods.
The trick to mocking extension methods is to realize that they are essentially just static methods disguised as instance methods. To do this we need:
- Extract extension methods into static utility classes: Move extension methods into dedicated classes that contain only static methods. This allows us to isolate extension methods for testing.
- Create a mock instance of a utility class: Using Moq, we can create a mock instance of a utility class. This will give us access to extension methods as mockable entities.
- Configure the mock to return the desired results: Once we have a mock instance of the utility class, we can configure it to return the specific results required for the test.
Here’s an example of how this works:
// 在实用程序类中定义扩展方法 public static class Utility { public static SomeType GetFirstWithId(this List<SomeType> list, int id) { return list.FirstOrDefault(st => st.Id == id); } } // 创建实用程序类的模拟实例 var mockUtility = new Mock<Utility>(); // 配置模拟以返回我们想要的结果 mockUtility.Setup(u => u.GetFirstWithId(It.IsAny<List<SomeType>>(), 5)).Returns(new SomeType { Id = 5 }); // 设置测试 var listMock = new Mock<List<SomeType>>(); listMock.Setup(l => l.Count).Returns(0); // 此示例中列表中没有实际项目 // 测试的断言 Assert.That(listMock.Object.GetFirstWithId(5), Is.Not.Null);
By moving the extension methods into a static utility class and mocking the class itself, we effectively overcome the limitation of not being able to directly override static extension methods. This workaround ensures that your tests remain robust even if future extension methods fail.
The above is the detailed content of How to Effectively Mock Extension Methods Using Moq?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
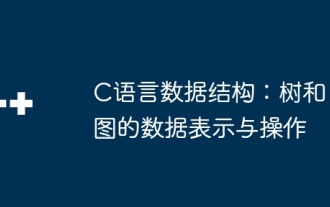
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
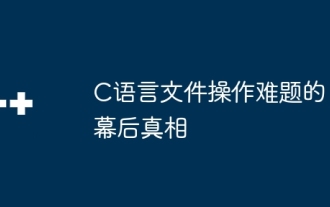
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
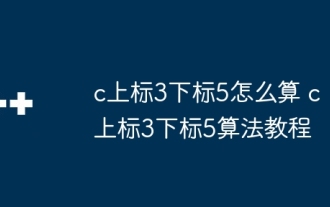
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
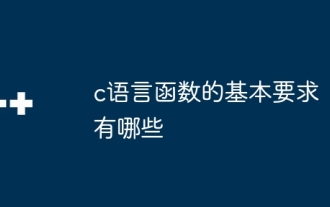
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
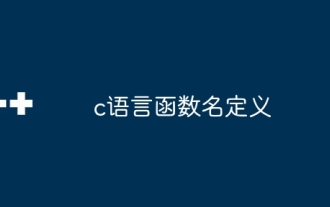
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
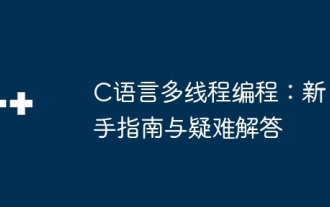
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
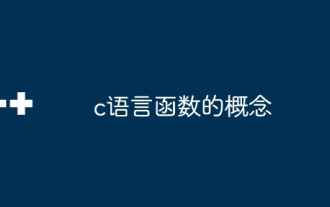
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
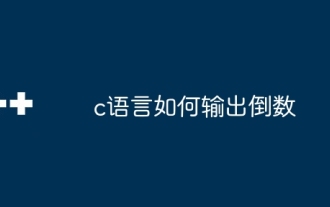
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
