How to use an object with v-model in Vue
Everyone is very familiar with the v-model
directive in Vue.js, which implements two-way data binding between components. But when manually implementing v-model
for a custom component, you usually run into some issues.
The usual approach is as follows:
const props = defineProps(['modelValue']); const emit = defineEmits(['update:modelValue']); <template></template>
Please note that we will not modify the value of the modelValue
prop inside the component. Instead, we pass the updated value back to the parent component via the emit
method, and the parent component makes the actual modifications. This is because: Child components should not affect the state of the parent component, which complicates the data flow and makes debugging difficult.
As stated in the Vue documentation, props should not be modified inside child components. If you do this, Vue will issue a warning in the console.
How is the subject doing?
Objects and arrays in JavaScript are a special case because they are passed by reference. This means that components can directly modify nested properties of object props. However, Vue does not warn about modifications in nested object properties (tracking these modifications incurs a performance penalty). Therefore, such unexpected changes can cause problems in your application that are difficult to detect and debug.
Most of the time we use the base value as v-model
. However, in some cases, such as when building a form component, we may need a custom v-model
that can handle objects. This leads to an important question:
How to implement a custom
v-model
to handle objects while avoiding the above pitfalls?
Discussion Questions
One way is to use a writable computed property or defineModel
helper function. However, both solutions have a significant drawback: they directly modify the original object, which defeats the purpose of maintaining a clear data flow.
To illustrate this problem, let’s look at an example of a “form” component. This component is designed to emit an updated copy of the object back to the parent component when the value in the form changes. We will try to achieve this using writable computed properties.
In this example, the writable computed property still modifies the original object.
import { computed } from 'vue'; import { cloneDeep } from 'lodash-es'; type Props = { modelValue: { name: string; email: string; }; }; const props = withDefaults(defineProps<Props>(), { modelValue: () => ({ name: '', email: '' }), }); const emit = defineEmits<{ 'update:modelValue': [value: Props['modelValue']]; }>(); const formData = computed({ // 返回的getter对象仍然是可变的 get() { return props.modelValue; }, // 注释掉setter仍然会修改prop set(newValue) { emit('update:modelValue', cloneDeep(newValue)); }, });
This doesn't work because the object returned from the getter is still mutable, causing the original object to be modified unexpectedly.
defineModel
Same thing. Since update:modelValue
is not emitted from the component and the object properties are modified without any warning.
Solution
The "Vue way" of handling this situation is to use internal reactive values to represent objects, and implement two observers:
- An observer monitors the
modelValue
prop for changes and updates the internal value. This ensures that the internal state reflects the latest prop values passed by the parent component. - An observer observes changes to internal values. When the internal value is updated, it emits a fresh cloned version of the object to the parent component to avoid directly modifying the original object.
To prevent an endless feedback loop between these two observers, we need to ensure that updates to the modelValue
prop don't accidentally retrigger the observer for the inner value.
const props = defineProps(['modelValue']); const emit = defineEmits(['update:modelValue']); <template></template>
I know what you’re thinking: “This is too much!” Let’s see how we can simplify it even more.
Simplify your solution with VueUse
Extracting this logic into a reusable composed function is a great way to simplify the process. But the good news is: we don’t even need to do that! The useVModel
combined function in VueUse can help us deal with this problem!
VueUse is a powerful Vue utility library, often referred to as the "Swiss Army Knife" of composed utilities. It's fully tree-shakable, so we can use only the parts we need without worrying about increasing the size of the package.
Here is an example before refactoring using useVModel
:
import { computed } from 'vue'; import { cloneDeep } from 'lodash-es'; type Props = { modelValue: { name: string; email: string; }; }; const props = withDefaults(defineProps<Props>(), { modelValue: () => ({ name: '', email: '' }), }); const emit = defineEmits<{ 'update:modelValue': [value: Props['modelValue']]; }>(); const formData = computed({ // 返回的getter对象仍然是可变的 get() { return props.modelValue; }, // 注释掉setter仍然会修改prop set(newValue) { emit('update:modelValue', cloneDeep(newValue)); }, });
Much simpler!
That’s it! We've explored how to properly use objects with v-model
in Vue without modifying it directly from child components. By using observers or leveraging compositional functions like VueUse's useVModel
, we can maintain clear and predictable state management in our application.
Here is a Stackblitz link with all the examples in this article. Feel free to explore and experiment.
Thank you for reading and happy coding!
The above is the detailed content of How to use an object with v-model in Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










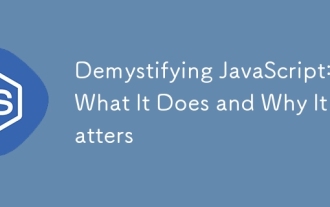
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
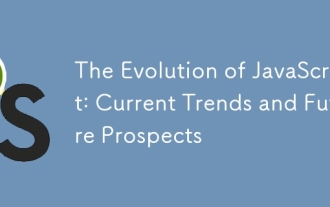
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
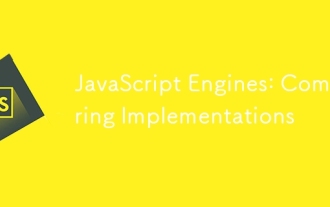
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
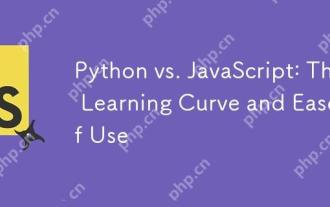
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
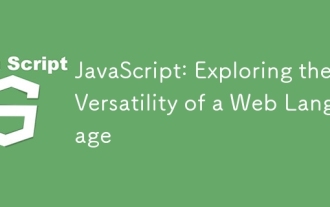
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
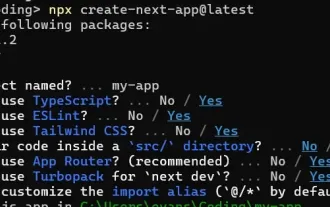
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
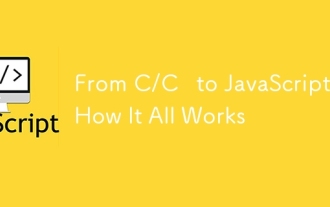
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
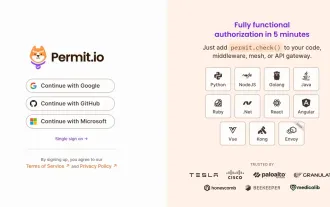
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
