Passing Properties in Java
Java Property Passing Mechanisms: A Comprehensive Guide
Java's parameter-passing mechanism is crucial for developers. This guide explores the various ways properties are passed in Java, impacting data manipulation within methods and classes.
Java employs a strict pass-by-value system. When a variable is passed, a copy of its value is created. This impacts how data changes within a method affect the original variable.
For primitive types (e.g., int
, float
, char
), a copy of the value is passed. Modifications inside the method don't affect the original.
public void modifyPrimitive(int number) { number = number + 10; }
With objects, a reference copy is passed (still pass-by-value). The copy points to the same memory location as the original object. Changes to the object's properties do affect the original.
public void modifyObject(MyClass obj) { obj.setProperty("New Value"); }
Constructors also use this pass-by-value mechanism. Properties are initialized via constructor parameters, creating initialized objects.
public class MyClass { private String property; public MyClass(String property) { this.property = property; } }
Here are several common approaches for passing properties in Java:
Property Passing Techniques
-
Method Arguments: Directly pass properties as method parameters. Suitable for simple, transient data.
public void greet(String name) { System.out.println("Hello, " + name); }
Copy after login -
Return Values: Return properties from a method.
public String getGreeting(String name) { return "Hello, " + name; }
Copy after login -
Class Variables (Instance Variables): Store properties as instance variables, accessible and modifiable by methods within the class.
public class MyClass { private String name; // ... getter and setter methods ... }
Copy after login -
Static Variables: Share properties across all instances of a class.
public class MyClass { private static String appName = "MyApp"; // ... method to access appName ... }
Copy after login -
Constructors: Initialize properties during object creation.
public class MyClass { private String name; public MyClass(String name) { this.name = name; } // ... }
Copy after login -
Getter and Setter Methods: Encapsulate fields with private access and provide public methods (
getName()
,setName()
) for controlled access. -
Collections (Lists, Maps, Sets): Pass properties as collection elements.
Map<String, String> userInfo = new HashMap<>(); // ... populate and use the map ...
Copy after login -
Property Files (
java.util.Properties
): Store properties in a.properties
file and load them at runtime. -
Dependency Injection: Frameworks like Spring manage property injection, passing properties through constructors or setters.
This detailed overview equips developers with a comprehensive understanding of Java's property-passing mechanisms, enabling them to write efficient and well-structured code. The choice of method depends on the specific design and complexity of the application.
The above is the detailed content of Passing Properties in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










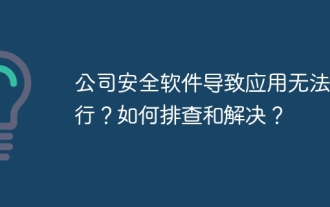
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
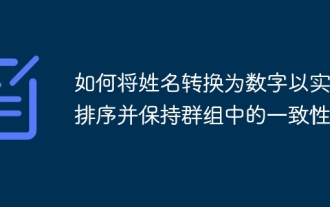
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
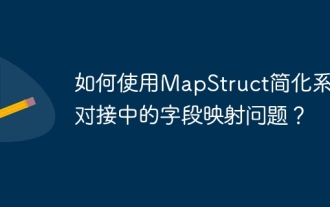
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
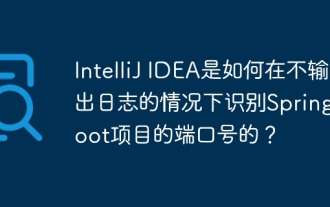
Start Spring using IntelliJIDEAUltimate version...
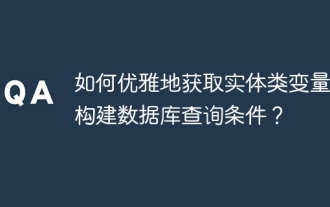
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
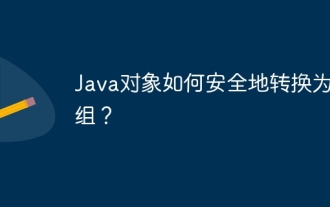
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
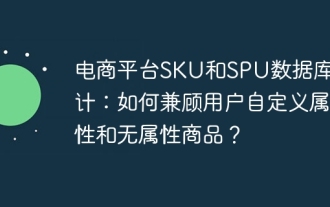
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
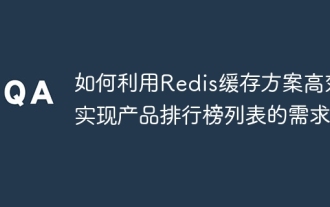
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
