How to Open Links in the Default Browser from a C# Application?
Opening URLs in Your Default Browser from C# Applications
When embedding a web browser control in a C# application, links may unexpectedly open in Internet Explorer, even if Google Chrome (or another browser) is your default. This is because the built-in WebBrowser
control essentially mimics Internet Explorer's behavior.
Here's how to ensure links open in your system's default browser:
Method 1: Handling the Navigating
Event
Intercept the Navigating
event of your web browser control:
private void webBrowser1_Navigating(object sender, WebBrowserNavigatingEventArgs e) { System.Diagnostics.Process.Start(e.Url.ToString()); e.Cancel = true; }
This code uses System.Diagnostics.Process.Start()
to launch the URL in the default browser when a link is clicked. e.Cancel = true;
prevents the link from opening within the WebBrowser
control itself.
Method 2: Directly Opening the URL
Alternatively, you can directly open a URL in the default browser:
System.Diagnostics.Process.Start("http://google.com");
This approach uses System.Diagnostics.Process.Start()
to launch the specified URL without needing event handling.
Summary:
By using either the Navigating
event handler or the direct System.Diagnostics.Process.Start()
method, you can reliably open links in your default browser from within your C# application, bypassing the limitations of the embedded WebBrowser
control.
The above is the detailed content of How to Open Links in the Default Browser from a C# Application?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


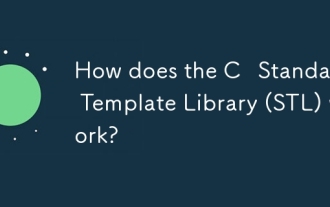
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
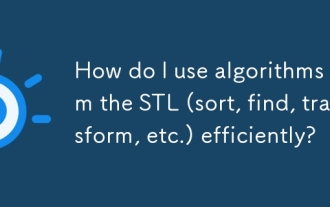
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
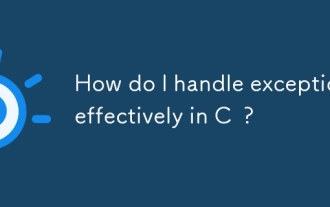
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
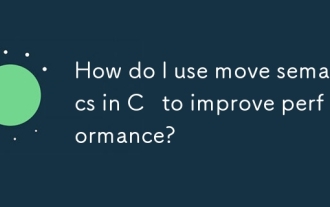
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
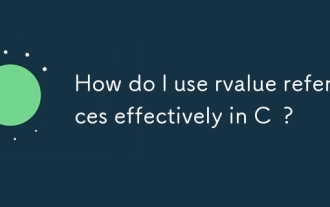
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
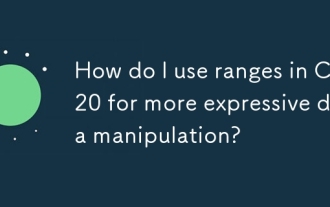
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
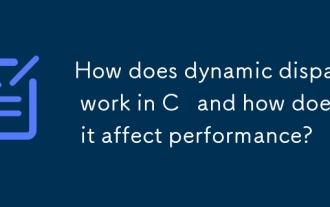
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
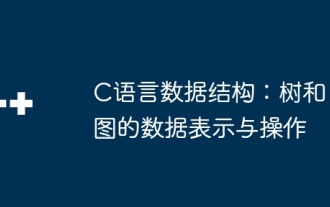
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
