AWS CDK Validation in Python
The AWS CDK application life cycle includes verification steps, which are explained in the CDK application synthesis chapter of the official documentation:
All constructs that implement the validate method can be self-validated to ensure they are in a state for correct deployment. Any validation failures that occur at this stage will be notified to you. In general, we recommend performing validation as quickly as possible (usually as soon as some input is obtained) and throwing exceptions as quickly as possible. Performing verification early improves reliability because stack traces will be more accurate and ensures your code can safely continue execution.
While there are some excellent articles on how to handle AWS CDK validation in TypeScript (linked below), I couldn't find a corresponding Python example. After creating some validations in Python, the following example complements the examples in these articles.
- Validation checks in AWS CDK constructs
- Use AWS CDK for validation (addValidation)
The following examples were developed using AWS CDK v2.176.0 and Python 3.13.1.
Example verification
The implementation of validation is done using the validate method which returns a list of error messages. If the list is empty, there are no errors; multiple entries correspond to different validation checks for which this validation failed. The validation logic is encapsulated in a class annotated with @jsii.implements(IValidation), indicating that it is the correct type for validation. In this example, there is a check that the Amazon S3 bucket passed to verify uses a custom AWS KMS key, which provides more control over the encryption process compared to the default encryption used by Amazon S3.
@jsii.implements(IValidation) class KodlotS3KmsValidator: """检查S3存储桶是否使用自定义KMS密钥加密。""" def __init__(self, s3_bucket: s3.Bucket): self.s3_bucket = s3_bucket def validate(self) -> List[str]: error_messages: List[str] = [] if self.s3_bucket.encryption_key is None: error_messages.append(f"存储桶必须使用自定义KMS密钥加密") return error_messages
AWS CDK Construction Example
This is a basic example of an AWS CDK construct that adds a single validation using the add_validation method of the constructor node in the scope tree. There was a bug during the implementation where a custom AWS KMS key was created but not passed to the encryption_key parameter of the Amazon S3 bucket construct.
class KodlotS3Bucket(Construct): """带有验证的S3存储桶。""" def __init__(self, scope: Construct, construct_id: str, bucket_name: str) -> None: super().__init__(scope, construct_id) s3_kms_key = kms.Key(self, f"KodlotS3KmsKey{bucket_name.title()}") self.s3_bucket = s3.Bucket( self, f"KodlotS3{bucket_name.title()}", bucket_name=bucket_name, encryption_key=None, ) self.node.add_validation(KodlotS3KmsValidator(self.s3_bucket))
This type of error is easily overlooked, which is where validation comes into play. Running a CDK composition or deployment using this example will result in the following error:
<code>RuntimeError: Error: 验证失败,错误如下: [KodlotS3Stack/KodlotBucket1] 存储桶必须使用自定义KMS密钥加密</code>
Multiple verifications
It is possible to have multiple validators testing different aspects of our configuration, and they can be reused for different constructs if we find common patterns like this. In this example, a validation check is added to ensure that the Amazon S3 bucket is not accidentally configured for static website hosting, in which case this would be an error.
@jsii.implements(IValidation) class KodlotS3NotWebsiteValidator: """检查S3存储桶未配置为静态网站托管。""" def __init__(self, s3_bucket: s3.Bucket): self.s3_bucket = s3_bucket def validate(self) -> List[str]: error_messages: List[str] = [] if self.s3_bucket.is_website: error_messages.append(f"存储桶不能用于静态网站托管") return error_messages
Updated AWS CDK construction example
To use an additional validator to check whether website hosting is configured for an Amazon S3 bucket, update the sample CDK construct as shown below. This time, the original error of not passing a custom AWS KMS key is retained. Now, in addition, website_index_document is incorrectly passed to the Amazon S3 bucket construct, indicating that it will be used for static website hosting.
@jsii.implements(IValidation) class KodlotS3KmsValidator: """检查S3存储桶是否使用自定义KMS密钥加密。""" def __init__(self, s3_bucket: s3.Bucket): self.s3_bucket = s3_bucket def validate(self) -> List[str]: error_messages: List[str] = [] if self.s3_bucket.encryption_key is None: error_messages.append(f"存储桶必须使用自定义KMS密钥加密") return error_messages
This time, we have two different issues with our Amazon S3 bucket, both of which are reported by the verification mechanism as shown below. Running a CDK composition or deployment using this example will result in the following error:
class KodlotS3Bucket(Construct): """带有验证的S3存储桶。""" def __init__(self, scope: Construct, construct_id: str, bucket_name: str) -> None: super().__init__(scope, construct_id) s3_kms_key = kms.Key(self, f"KodlotS3KmsKey{bucket_name.title()}") self.s3_bucket = s3.Bucket( self, f"KodlotS3{bucket_name.title()}", bucket_name=bucket_name, encryption_key=None, ) self.node.add_validation(KodlotS3KmsValidator(self.s3_bucket))
This is very powerful because we can collect different configuration issues in the cloud infrastructure and resolve them all without having to rerun and fix them again and again.
AWS CDK Stack Verification
It’s worth remembering that validation is not limited to AWS CDK constructs and can be added to the AWS CDK stack level as well. Here we can for example check if a given stack does not contain unwanted resources. If our requirement is that the stack should not contain any AWS Lambda resources, then we can simulate that requirement using the validation described below. Using the child of a node construct, you can use Python's built-in isinstance function to check whether any resource is an instance of an AWS CDK function.
<code>RuntimeError: Error: 验证失败,错误如下: [KodlotS3Stack/KodlotBucket1] 存储桶必须使用自定义KMS密钥加密</code>
AWS CDK Stack Example
To add validation to the stack we use the same add_validation method as the constructor. Since KodlotS3Stack is identified as a stack that must not contain any AWS Lambda functions, it is a good place to test the validation of the example stack. If an error occurs during development, such as the one described below, where a developer defines an AWS Lambda function resource, the verification will take effect.
@jsii.implements(IValidation) class KodlotS3NotWebsiteValidator: """检查S3存储桶未配置为静态网站托管。""" def __init__(self, s3_bucket: s3.Bucket): self.s3_bucket = s3_bucket def validate(self) -> List[str]: error_messages: List[str] = [] if self.s3_bucket.is_website: error_messages.append(f"存储桶不能用于静态网站托管") return error_messages
Running the CDK composition or deployment of this example will result in the following error:
class KodlotS3Bucket(Construct): """带有验证的S3存储桶。""" def __init__(self, scope: Construct, construct_id: str, bucket_name: str) -> None: super().__init__(scope, construct_id) s3_kms_key = kms.Key(self, f"KodlotS3KmsKey{bucket_name.title()}") self.s3_bucket = s3.Bucket( self, f"KodlotS3{bucket_name.title()}", bucket_name=bucket_name, encryption_key=None, website_index_document="index.html", ) self.node.add_validation(KodlotS3KmsValidator(self.s3_bucket)) self.node.add_validation(KodlotS3NotWebsiteValidator(self.s3_bucket))
Built-in verification
It is also worth checking whether the AWS CDK already provides checks for certain aspects of our cloud infrastructure. In the context of Amazon S3, it turns out that if we configure the bucket name to k1, resulting in an illegal name length, we get the following built-in error:
<code>RuntimeError: Error: 验证失败,错误如下: [KodlotS3Stack/KodlotBucket1] 存储桶必须使用自定义KMS密钥加密 [KodlotS3Stack/KodlotBucket1] 存储桶不能用于静态网站托管</code>
Similarly, if we configure the bucket name as k_1, resulting in an illegal underscore character in it, the following built-in error will occur:
@jsii.implements(IValidation) class KodlotNoLambdaInStackValidator: """检查给定堆栈中未定义任何Lambda函数。""" def __init__(self, stack: cdk.Stack): self.stack = stack def validate(self) -> List[str]: error_messages: List[str] = [] for construct in self.stack.node.children: if isinstance(construct, _lambda.Function): error_messages.append(f"堆栈不能包含任何Lambda函数") return error_messages
Conclusion
AWS CDK validation is a useful mechanism that developers can use in conjunction with unit and integration tests to ensure the overall quality of the cloud computing infrastructure. Being able to catch common misconfigurations and define standards will lead to reusable validations that can be shared between different components. Validation can be added at different levels defined by AWS CDK, including AWS CDK constructs and AWS CDK stacks. It's good to see that the AWS CDK has captured some common validations, as they are fundamental to various cloud services and resources and should always be checked, reducing the likelihood of encountering deployment errors in a production environment.
The code blocks are preserved and the image is referenced correctly. The overall structure and meaning remain the same, while the wording is altered for a more natural flow and to avoid direct repetition.
The above is the detailed content of AWS CDK Validation in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










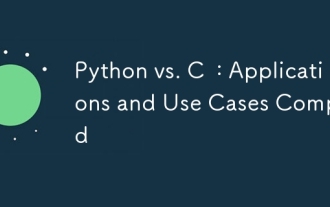
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
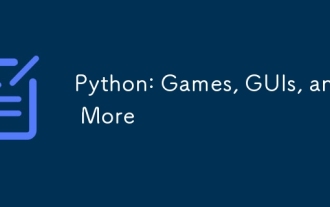
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
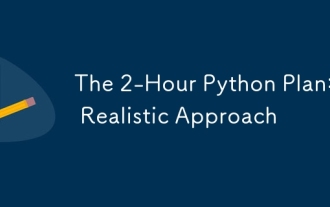
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
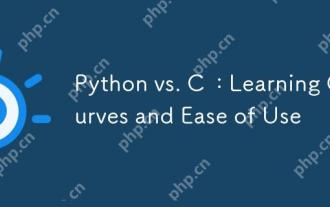
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
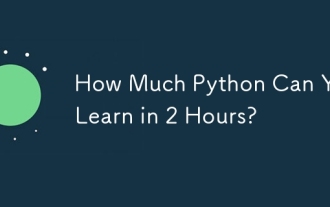
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
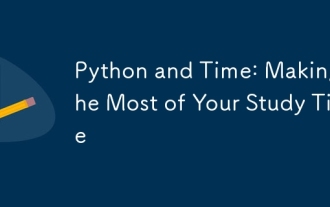
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
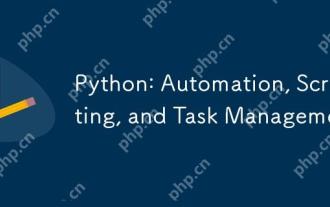
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
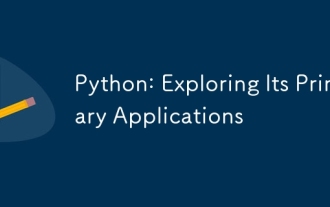
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
