React - New API: use
React 19’s use
API brings revolutionary improvements to easily handle data acquisition and asynchronous operations in components! ? This new approach, through direct integration with Suspense, lets you write cleaner, more readable code without cumbersome lifecycle methods and additional state management. ?
Purpose
The use
API in React 19 simplifies getting data and handling asynchronous resources directly in the component's render function. This eliminates the need for separate lifecycle methods or complex state management to handle loading and error states.
import { Suspense } from 'react' async function fetchData() { const response = await fetch('https://api.example.com/data') return await response.json() } function MyComponent() { const data = use(fetchData) return ( <Suspense fallback={<div>Loading Data...</div>}> <div> <h1>My Data Header</h1> <p>{data.message}</p> </div> </Suspense> ) }
How it works
- 1. Import Suspense: We import Suspense to handle the loading state.
-
2. Define an asynchronous function: We define an asynchronous function
fetchData
to get data from the API. -
3. Call
use
: In the component’s render function, we usefetchData
as a parameter to calluse
. - 4. Suspense wrapper: We use Suspense to wrap the content and provide a fallback message ("Loading Data...") when getting the data.
-
5. Rendering data: Once the data is available, the data provided by
use
will be used to render the content (message in the example above).
Advantages
1. More concise code
use
API makes your component logic concise and clear, focusing on UI rendering. It eliminates the boilerplate code typically needed to handle asynchronous operations.
2. Improve readability
By integrating with React’s Suspense mechanism, the use
API makes the data fetching and rendering process clearer, making it easier to understand the code.
3. Reduce errors
Auto-suspend during data acquisition helps prevent rendering issues that can occur when data is not yet available.
Practical Application
1. Obtain user data
use
API can be used to get user data from API and display on profile page. Components pause rendering until user data is available, ensuring a smooth user experience.
2. Load comments
Imagine a blog post component that gets comments from an API. use
The API can handle this by pausing the rendering of comments until they load, while showing a loading indicator.
3. Real-time data update
Theuse
API can also be used with libraries like WebSockets
to get real-time data updates. The component pauses until updates arrive and then re-renders with the latest information.
Conclusion
To summarize, the use
API in React 19 simplifies asynchronous operations and improves application performance by reducing boilerplate code and potential errors. Give it a try and experience a smoother, more efficient development experience! ?✨
The above is the detailed content of React - New API: use. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










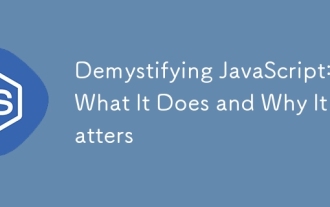
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
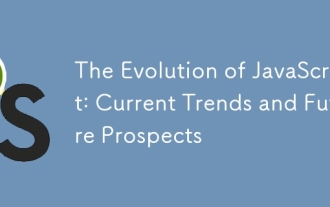
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
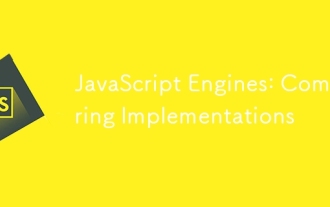
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
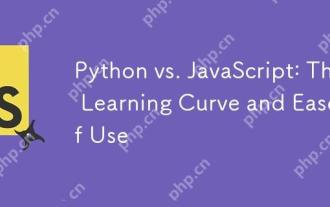
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
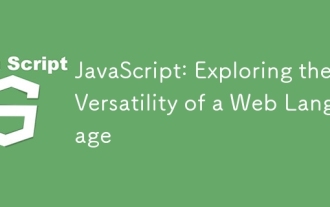
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
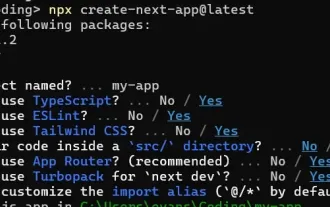
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
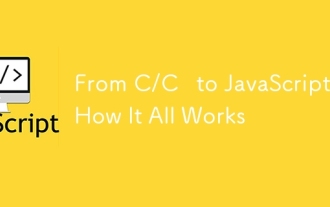
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
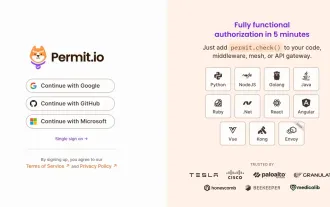
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
