The importance of backtracking for developpers
Backtracking: A Powerful Problem-Solving Technique
Backtracking is a versatile algorithmic approach used across various programming languages to systematically explore all potential solutions to a problem. It's particularly effective for tackling complex scenarios with numerous possible outcomes, such as navigating mazes, solving the N-Queens puzzle, or cracking Sudoku.
Why Use Backtracking?
When faced with a problem containing a vast number of potential solutions, manual verification becomes impractical. While iterative loops might seem like an alternative, they often strain computational resources. Backtracking provides an elegant solution. It efficiently explores each possibility; if a path proves unproductive, it retraces its steps ("backtracks") to explore alternative options until a valid solution is found.
Illustrative Example: Sudoku
Consider the classic Sudoku puzzle: Each row, column, and 3x3 subgrid must contain the digits 1 through 9 without repetition.
Solving a Sudoku puzzle using backtracking involves these steps:
- Validation Function: A function checks if placing a number in a specific cell adheres to all Sudoku rules.
- Recursive Exploration: Once a valid placement is confirmed, the algorithm recursively explores possibilities for the remaining empty cells.
- Backtracking Mechanism: If a placement leads to a conflict later, the algorithm backtracks, removes the incorrect number, and tries a different digit. This iterative process continues until all cells are filled correctly.
Core Principles of Backtracking
- Selection: Evaluate all feasible choices at each step.
- Constraint Check: Verify if a chosen option satisfies the problem's rules.
- Goal Test: Determine if the current solution fulfills all conditions.
- Retrace: If a choice leads to an invalid state, backtrack to explore other alternatives.
JavaScript Sudoku Solver (Illustrative Code)
// Partially filled Sudoku board (empty cells represented by ".") const board = [ ["5", "3", ".", "6", "7", "8", "9", "1", "2"], ["6", "7", "2", "1", "9", "5", "3", "4", "8"], ["1", "9", "8", "3", "4", "2", "5", "6", "7"], ["8", "5", "9", "7", "6", "1", "4", "2", "3"], ["4", "2", "6", "8", ".", "3", "7", "9", "1"], ["7", "1", "3", "9", "2", "4", "8", "5", "6"], ["9", "6", "1", "5", "3", "7", "2", "8", "4"], ["2", "8", "7", "4", "1", "9", "6", "3", "5"], ["3", "4", "5", "2", "8", "6", "1", ".", "9"] ]; // Valid Sudoku digits const possibleNumbers = ["1", "2", "3", "4", "5", "6", "7", "8", "9"]; // Function to check validity of a number placement function isValid(number, row, col, board) { // ... (Implementation to check row, column, and subgrid constraints) ... } // Recursive backtracking function to solve Sudoku function solveSudoku(board, emptySpaces, emptySpaceIndex) { // ... (Implementation of recursive backtracking logic) ... } // ... (Rest of the code to find empty spaces and initiate the solving process) ...
Key Takeaways
Backtracking offers a systematic and efficient way to explore solution spaces while adhering to constraints. Its recursive nature makes it particularly well-suited for constraint satisfaction problems. The provided code snippet demonstrates a basic framework for a Sudoku solver using this powerful technique.
Image Credit: Image by storyset on Freepik
The above is the detailed content of The importance of backtracking for developpers. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










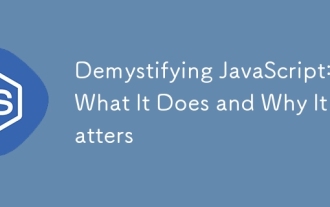
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
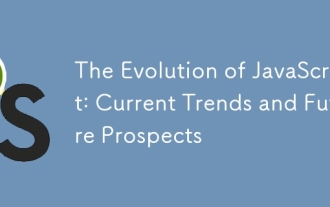
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
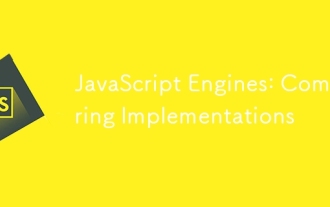
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
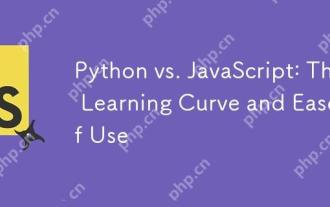
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
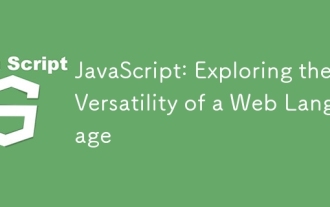
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
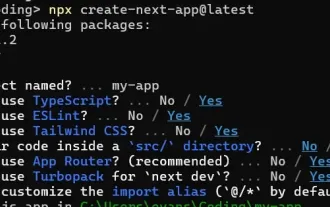
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
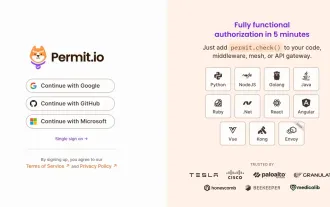
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
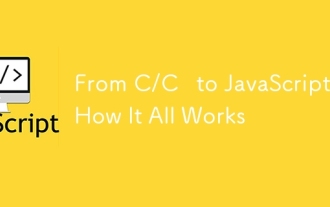
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
