Some Tips Typescript Design Pattern
Referencing Vilic Vane's "TypeScript Design Patterns," available on Amazon: https://www.php.cn/link/2e51055e7d09f972c49336144993e082
Chapter 2 Analysis: Navigating the Challenges of Expanding Complexity
Chapter 2 tackles the inherent complexities that arise as software projects scale. It highlights how disorganized codebases rapidly become unwieldy and offers solutions by emphasizing pattern identification and abstraction for enhanced maintainability.
Core Concepts
- The Complexity Conundrum: Addressing the escalating challenges of growing systems.
- Establishing Foundational Elements: Building the basic framework for manageable complexity.
- Common Development Pitfalls: Recognizing and avoiding typical coding errors.
- Strategies for Improvement: Implementing techniques for better code structure and scalability.
1. The Complexity Conundrum
The chapter uses a client-server synchronization system as a case study. Initially simple, handling a single data type, the system's complexity explodes as features are added (multiple data types, clients, conflict resolution). This illustrates how unstructured code quickly becomes difficult to manage.
Real-World Example:
Consider an HR system synchronizing employee data. Starting with just names, adding roles, salaries, and vacation time without a structured approach leads to a brittle and error-prone system.
2. Establishing Foundational Elements
Basic synchronization is achieved by comparing timestamps. The server sends data with the latest timestamp; the client sends back updated data with newer timestamps.
Basic TypeScript Code Example:
type DataItem = { id: number; value: string; timestamp: number }; function syncToClient(serverData: DataItem[], clientData: DataItem[]): DataItem[] { return serverData.filter(serverItem => { const clientItem = clientData.find(item => item.id === serverItem.id); return !clientItem || clientItem.timestamp < serverItem.timestamp; }); } function syncToServer(serverData: DataItem[], clientData: DataItem[]): DataItem[] { return clientData.filter(clientItem => { const serverItem = serverData.find(item => item.id === clientItem.id); return !serverItem || serverItem.timestamp < clientItem.timestamp; }); }
This basic approach, however, lacks scalability as the system grows.
3. Common Development Pitfalls
The author highlights common issues in poorly structured systems:
- Obscured Relationships: Data dependencies and relationships are ignored, leading to inconsistencies.
- Redundant Code: Repeated code increases maintenance burden.
- Lack of Abstraction: Complex logic is handled directly, resulting in tangled code.
Real-World Example:
In the HR system, neglecting the relationships between employees, departments, and organizations leads to data inconsistencies (e.g., assigning employees to non-existent departments).
4. Strategies for Improvement
The chapter advocates for:
- Identifying Abstractions: Refactoring repeated logic into reusable functions or classes.
- Decomposing Complex Processes: Breaking down complex tasks into smaller, manageable units.
- Applying Design Patterns: Utilizing patterns like the Strategy Pattern for modularity and reusability.
Improved Code Using the Strategy Pattern:
type DataItem = { id: number; value: string; timestamp: number }; function syncToClient(serverData: DataItem[], clientData: DataItem[]): DataItem[] { return serverData.filter(serverItem => { const clientItem = clientData.find(item => item.id === serverItem.id); return !clientItem || clientItem.timestamp < serverItem.timestamp; }); } function syncToServer(serverData: DataItem[], clientData: DataItem[]): DataItem[] { return clientData.filter(clientItem => { const serverItem = serverData.find(item => item.id === clientItem.id); return !serverItem || serverItem.timestamp < clientItem.timestamp; }); }
Key Takeaways
- Thorough Requirements Analysis: Understanding data relationships before implementation.
- Scalable Design: Employing design patterns for flexibility and maintainability.
- Simplified Logic: Avoiding overly complex functions and classes.
The above is the detailed content of Some Tips Typescript Design Pattern. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










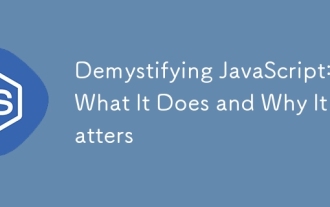
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
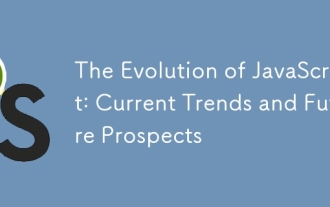
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
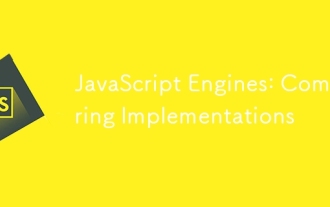
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
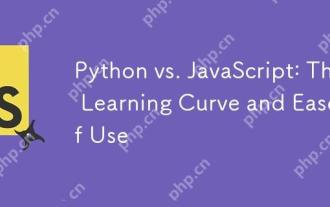
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
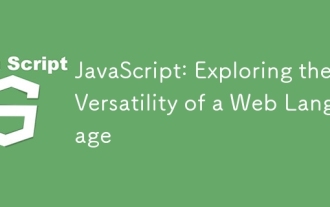
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
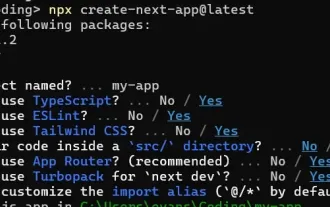
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
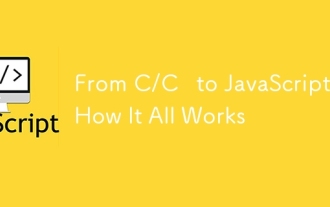
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
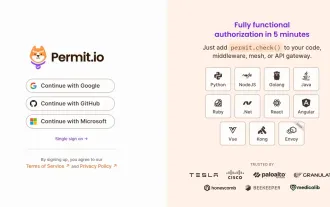
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
