Utilizing Promise.all()
Use once
await
to wait for multiple asynchronous processes.
One of the strengths of JavaScript is its ability to perform asynchronous operations to avoid processes waiting for each other. In practical applications, asynchronous operations are often used for processes whose waiting time depends on external factors, such as network connection, storage speed, etc. Here are some examples of asynchronous operations:
- Database query
- Get data from other websites (HTTP request)
- Read and write files
- Send email
Execution of asynchronous functions
In JavaScript, asynchronous operations are usually implemented using functions. A function is a set of blocks of code that perform a specific task, such as an addition function to calculate a sum and a shutdown function to shut down the computer (maybe there is such a function?).
Define function
To define an asynchronous function, just add the async
keyword when the function is declared, and the rest is the same as an ordinary function.
For example, we define a function to send emails asynchronously:
async function kirimEmail(tujuan, judul, isi) { // 发送邮件 // ... }
Or use arrow functions:
const kirimEmail = async (tujuan, judul, isi) => { // 发送邮件 // ... }
Call function
When the above function is called, it will automatically execute asynchronously, which means there will be no waiting for each other.
For example:
kirimEmail('contoh1@email.com', 'Tes 1 Email', 'Halo. Ini saya lagi ngetes.'); kirimEmail('contoh2@email.com', 'Tes 2 Email', 'Halo. Ini saya lagi ngetes.'); kirimEmail('contoh3@email.com', 'Tes 3 Email', 'Halo. Ini saya lagi ngetes.');
In the above example, all processes that send emails are executed in sequence, but no one process is waited for to complete. Therefore, the next process will be started while the previous process is not completed and will not block each other.
If you need to get data or wait for an asynchronous process to complete, you can use the await
keyword when calling a function.
For example:
await kirimEmail('contoh1@email.com', 'Tes 1 Email', 'Halo. Ini saya lagi ngetes.'); await kirimEmail('contoh2@email.com', 'Tes 2 Email', 'Halo. Ini saya lagi ngetes.'); await kirimEmail('contoh3@email.com', 'Tes 3 Email', 'Halo. Ini saya lagi ngetes.');
In this example, each process will wait for the previous process to complete. This usually happens when data needs to be fetched from one process for the next process, so one has to wait for the previous process to complete before fetching the required data.
Promise.all()
Using Promise.all
, we can call and wait for multiple asynchronous functions at the same time.
Call function
An example of using Promise.all
to call multiple asynchronous functions is as follows:
await Promise.all([ kirimEmail('contoh1@email.com', 'Tes 1 Email', 'Halo. Ini saya lagi ngetes.'), kirimEmail('contoh2@email.com', 'Tes 2 Email', 'Halo. Ini saya lagi ngetes.'), kirimEmail('contoh3@email.com', 'Tes 3 Email', 'Halo. Ini saya lagi ngetes.'), ]);
In the above example, we wait for the completion of three sending mail processes, and the results will be returned in the form of an array regardless of success or failure.
Features
Promise.all
has the following properties:
- The asynchronous function to be executed is placed in an array;
- All functions are executed as a whole, from startup to completion;
- Since they work as a whole, all processes will wait until completion, especially if
await
is used; - If an error occurs in one of the processes, all processes will report an error.
Please refer to MDN's Promise.all
documentation for more details.
Conclusion
Using Promise.all
is very convenient, we only need to write await
? once, and it is also useful if we want to stop all processes if one of them errors. However, if we want to continue executing other processes even if one process fails, we will discuss this in the next article.
Thank you for reading. If you want to discuss, please leave a message. If you want to make friends, please let me know ?
The above is the detailed content of Utilizing Promise.all(). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










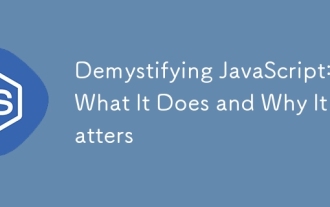
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
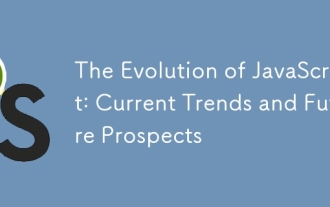
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
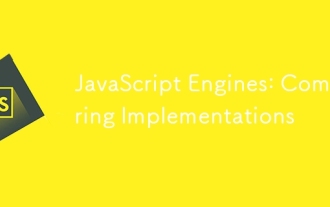
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
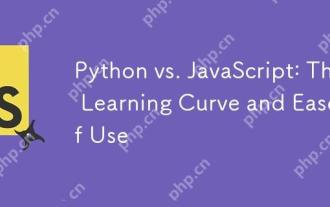
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
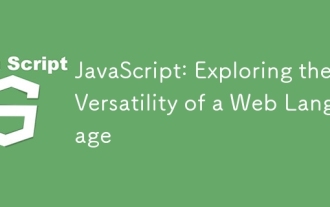
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
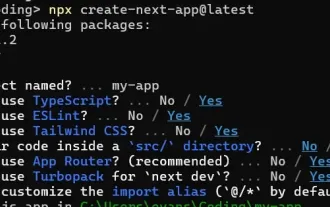
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
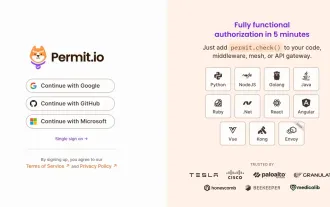
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
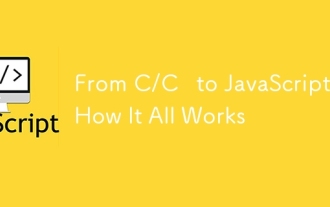
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
