Safe Ways to Access Data on Objects
Cleverly avoid errors caused by null values of JavaScript objects
In JavaScript, secure access to object data is crucial to ensure that applications can function properly even if the data is incomplete. The core is to avoid errors caused by null values.
Attention!
To better understand this article, you need to understand the concepts of truthy and falsy values in JavaScript.
Case
When writing code, we often need to access data in objects. Suppose we have an employee object and need to get its status information.
If the status information exists, the status will be displayed; if it does not exist, "Internship" will be displayed.
The sample code is as follows:
const pegawai = { nama: 'Alex Under', status: 'tetap', }; console.log(pegawai.nama, 'adalah pegawai', pegawai.status);
Output result:
<code>Alex Under adalah pegawai tetap</code>
Problem and Solution 1
Now, we reduce the properties of the object:
const pegawai = { }; console.log(pegawai.nama, 'adalah pegawai', pegawai.status);
Output result:
<code>undefined adalah pegawai undefined</code>
Although there is no error reported here, the data is displayed as undefined
, which does not look pretty.
To solve this problem, we can add the if-else
statement:
const pegawai = { }; if (!pegawai.nama) { pegawai.nama = 'Seorang Pegawai'; } if (!pegawai.status) { pegawai.status = 'magang'; } console.log(pegawai.nama, 'adalah pegawai', pegawai.status);
Output result:
<code>Seorang Pegawai adalah pegawai magang</code>
We provide a default value for the data, if the data does not exist, the default value is used.
Additional Tips
To simplify the code, you can use the following method:
const pegawai = { }; pegawai.nama = pegawai.nama || 'Seorang Pegawai'; pegawai.status = pegawai.status || 'magang'; console.log(pegawai.nama, 'adalah pegawai', pegawai.status);
Problem and Solution 2
What happens if the object itself does not exist (as null
)?
const pegawai = null; console.log(pegawai.nama, 'adalah pegawai', pegawai.status);
This will result in an error like this:
<code>Uncaught TypeError: Cannot read properties of null (reading 'nama')</code>
App crashed with error.
To solve this problem, we can use the following method:
const pegawai = null; const pegawaiSafe = pegawai || {}; if (!pegawaiSafe.nama) { pegawaiSafe.nama = 'Seorang Pegawai'; } if (!pegawaiSafe.status) { pegawaiSafe.status = 'magang'; } console.log(pegawaiSafe.nama, 'adalah pegawai', pegawaiSafe.status);
In this way, no error will be reported, and the output result is:
<code>Seorang Pegawai adalah pegawai magang</code>
Additional Tips
Similarly, to simplify the code, you can use the following:
const pegawai = null; const pegawaiSafe = {}; pegawaiSafe.nama = (pegawai || {}).nama || 'Seorang Pegawai'; pegawaiSafe.status = (pegawai || {}).status || 'magang'; console.log(pegawaiSafe.nama, 'adalah pegawai', pegawaiSafe.status);
or:
const pegawai = null; const pegawaiSafe = pegawai || {}; pegawaiSafe.nama = pegawaiSafe.nama || 'Seorang Pegawai'; pegawaiSafe.status = pegawaiSafe.status || 'magang'; console.log(pegawaiSafe.nama, 'adalah pegawai', pegawaiSafe.status);
Summary
Through the above method, we can effectively avoid the object null value problem, especially when dealing with objects from external input (such as user input, database, third-party services, etc.).
Thank you for reading!
Welcome to discuss and communicate, and also welcome to make friends?
The above is the detailed content of Safe Ways to Access Data on Objects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
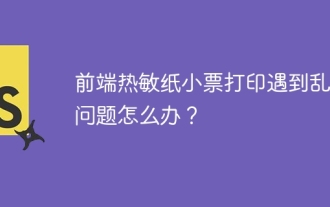
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
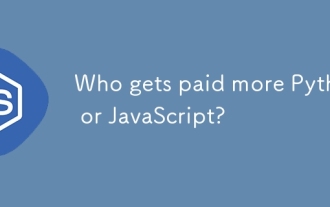
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
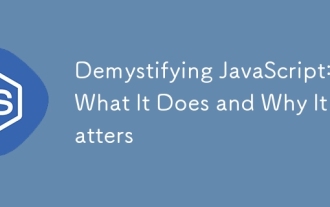
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
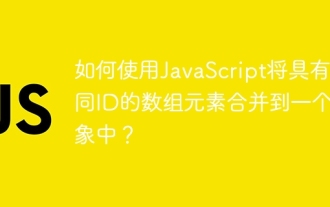
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
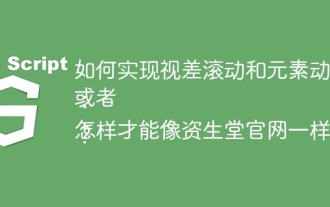
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
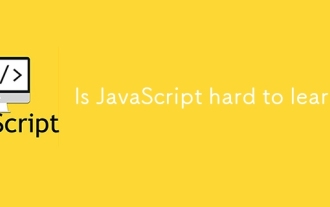
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
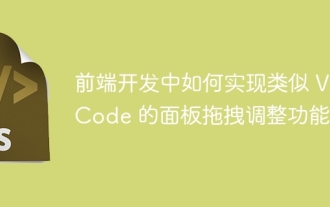
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
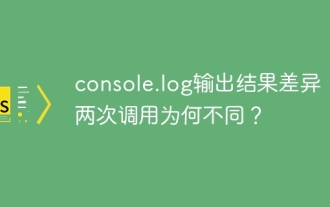
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
