


How Can I Prevent and Handle StackOverflowExceptions in XslCompiledTransform?
Addressing StackOverflowExceptions in XslCompiledTransform
During Xsl Editor development, encountering a StackOverflowException
when calling XslCompiledTransform.Transform
can be problematic. This exception typically stems from an infinitely recursive Xsl script, overwhelming the stack during transformation.
Microsoft recommends proactive prevention instead of relying on try-catch
blocks, which are ineffective against this specific exception. A counter or state mechanism within the Xsl script itself can interrupt recursive loops, preventing stack overflow.
However, if the exception originates from a .NET internal method, alternative strategies are required:
- Proactive Recursion Detection: Implement code to analyze the Xsl script for potential infinite recursion, warning users before execution.
-
Isolate XslTransform in a Separate Process: Run the
XslTransform
in a separate process. This isolates the potential crash, allowing the main application to continue and inform the user of the failure.
To isolate the transform into a separate process, use this code in your main application:
// Example demonstrating argument passing in StartInfo Process p1 = new Process(); p1.StartInfo.FileName = "ApplyTransform.exe"; p1.StartInfo.UseShellExecute = false; p1.StartInfo.WindowStyle = ProcessWindowStyle.Hidden; p1.Start(); p1.WaitForExit(); if (p1.ExitCode == 1) Console.WriteLine("A StackOverflowException occurred.");
In the separate process (ApplyTransform.exe
), handle the exception like this:
class Program { static void Main(string[] args) { AppDomain.CurrentDomain.UnhandledException += CurrentDomain_UnhandledException; try { //Your XslTransform code here throw new StackOverflowException(); //Example - Replace with your actual transform code } catch (StackOverflowException) { Environment.Exit(1); } } static void CurrentDomain_UnhandledException(object sender, UnhandledExceptionEventArgs e) { if (e.IsTerminating) { Environment.Exit(1); } } }
This revised example provides a more robust and clear solution for handling the StackOverflowException
. The try-catch
block in Main
now specifically catches the StackOverflowException
and the UnhandledException
event handler ensures a clean exit, preventing the "Illegal Operation" dialog. Remember to replace the example throw new StackOverflowException();
with your actual Xsl transformation code.
The above is the detailed content of How Can I Prevent and Handle StackOverflowExceptions in XslCompiledTransform?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


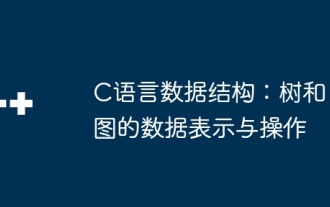
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
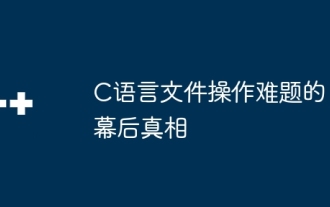
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
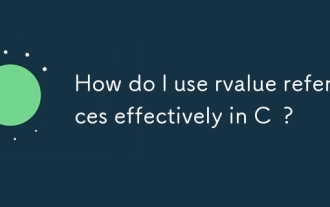
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
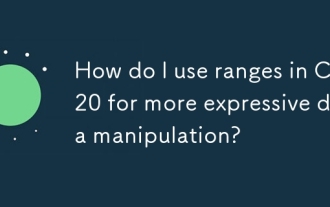
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
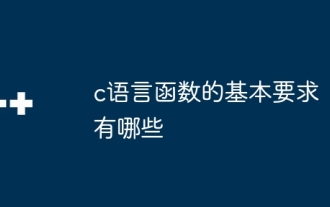
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
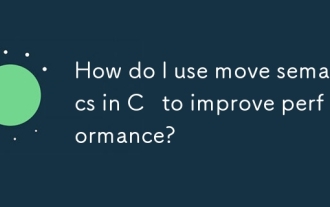
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
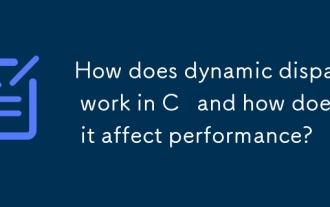
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
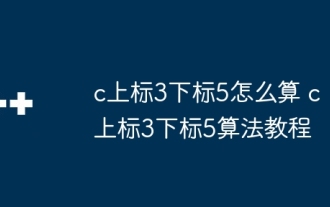
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
