


How to Retrieve Multiple Keys Associated with a Specific Value in a Generic Dictionary?
Get multiple keys for a specific value in a generic dictionary
In .NET, retrieving the value associated with a key in a generic dictionary is very simple. However, determining the key of a given value is not easy, especially when multiple keys may correspond to the same value.
Question
Consider the following code snippet:
Dictionary<int, string> greek = new Dictionary<int, string>(); greek.Add(1, "Alpha"); greek.Add(2, "Beta"); int[] betaKeys = greek.WhatDoIPutHere("Beta"); // 预期结果为单个 2
The goal is to get an array containing keys that map to the value "Beta".
Solution
We can create a custom two-way dictionary that is able to retrieve keys and values:
using System; using System.Collections.Generic; using System.Linq; class BiDictionary<TFirst, TSecond> { private IDictionary<TFirst, IList<TSecond>> firstToSecond = new Dictionary<TFirst, IList<TSecond>>(); private IDictionary<TSecond, IList<TFirst>> secondToFirst = new Dictionary<TSecond, IList<TFirst>>(); public void Add(TFirst first, TSecond second) { IList<TSecond> seconds; IList<TFirst> firsts; if (!firstToSecond.TryGetValue(first, out seconds)) { seconds = new List<TSecond>(); firstToSecond[first] = seconds; } if (!secondToFirst.TryGetValue(second, out firsts)) { firsts = new List<TFirst>(); secondToFirst[second] = firsts; } seconds.Add(second); firsts.Add(first); } public IEnumerable<TSecond> GetByFirst(TFirst first) { IList<TSecond> list; return firstToSecond.TryGetValue(first, out list) ? list : Enumerable.Empty<TSecond>(); } public IEnumerable<TFirst> GetBySecond(TSecond second) { IList<TFirst> list; return secondToFirst.TryGetValue(second, out list) ? list : Enumerable.Empty<TFirst>(); } }
To use this two-way dictionary, we can replace the code in the previous example with:
BiDictionary<int, string> greek = new BiDictionary<int, string>(); greek.Add(1, "Alpha"); greek.Add(2, "Beta"); greek.Add(5, "Beta"); IEnumerable<int> betaKeys = greek.GetBySecond("Beta"); foreach (int key in betaKeys) { Console.WriteLine(key); // 2, 5 }
This solution effectively provides a way to retrieve all keys associated with a specified value in a multi-valued dictionary.
The above is the detailed content of How to Retrieve Multiple Keys Associated with a Specific Value in a Generic Dictionary?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
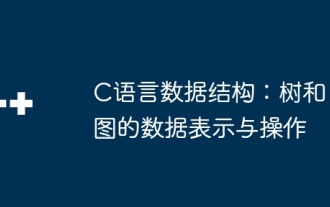
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
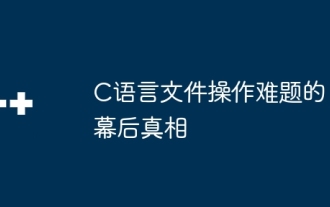
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
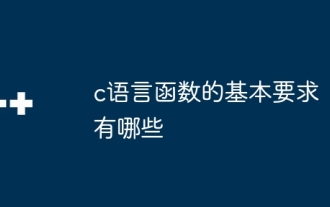
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
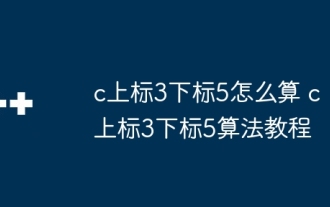
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
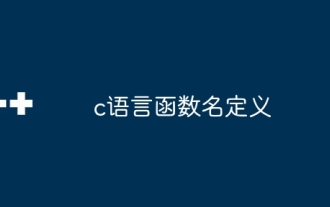
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
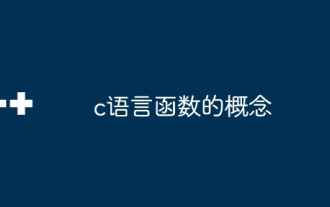
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
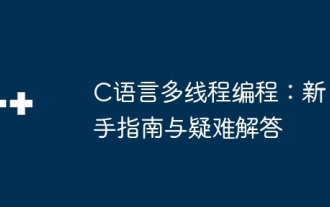
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
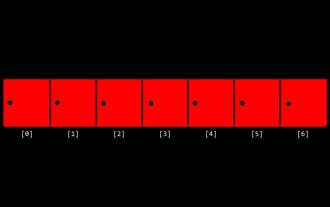
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
