


How to Efficiently Find an Element in a Large JSON Array in PostgreSQL?
Optimizing JSON Array Element Searches in PostgreSQL
Efficiently locating specific elements within large JSON arrays stored in PostgreSQL is crucial for performance. While PostgreSQL's json_array_elements
function is readily available, it can significantly impact query speed when dealing with extensive arrays.
A common approach involves utilizing a GIN index on the JSON array. However, this method is limited to arrays containing primitive data types (numbers, strings). Arrays of JSON objects won't benefit from this indexing strategy.
A more robust solution involves creating a custom function to extract the desired element and then indexing the extracted value. This allows for efficient lookups even with complex JSON array structures.
Example Implementation:
Here's how to create a function to extract an element based on a key and subsequently index it using GIN:
CREATE OR REPLACE FUNCTION extract_element(j JSONB, key TEXT) RETURNS TEXT AS $$ SELECT value ->> key FROM jsonb_each(j) WHERE key = key $$ LANGUAGE SQL IMMUTABLE; CREATE INDEX tracks_artists_gin_idx ON tracks USING GIN (extract_element(artists, 'name'));
This function, extract_element
, takes a JSONB object (j
) and a key (key
) as input. It uses jsonb_each
to iterate through the JSONB object and extracts the value associated with the specified key using ->>
. The WHERE
clause ensures only the matching key's value is returned. The index is then created on the result of this function applied to the 'artists' column (assuming 'artists' is a JSONB column containing an array of JSON objects, each with a 'name' key).
Improved Query Performance:
With this index in place, queries like the following will leverage the index for significantly faster execution:
SELECT * FROM tracks WHERE artists @> '[{"name": "The Dirty Heads"}]';
This query, previously resulting in a full table scan, now efficiently uses the GIN index, drastically improving performance for large datasets.
The above is the detailed content of How to Efficiently Find an Element in a Large JSON Array in PostgreSQL?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










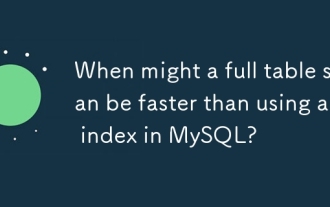
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
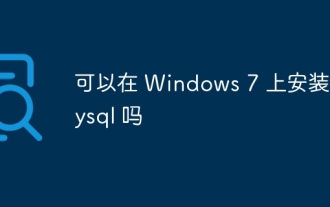
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
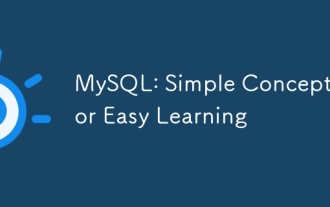
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
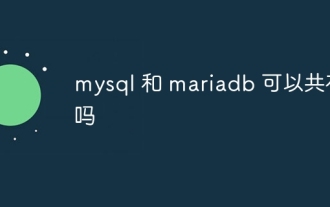
MySQL and MariaDB can coexist, but need to be configured with caution. The key is to allocate different port numbers and data directories to each database, and adjust parameters such as memory allocation and cache size. Connection pooling, application configuration, and version differences also need to be considered and need to be carefully tested and planned to avoid pitfalls. Running two databases simultaneously can cause performance problems in situations where resources are limited.
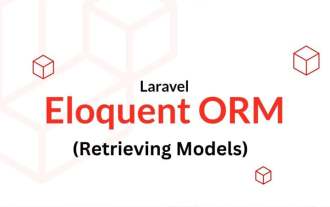
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
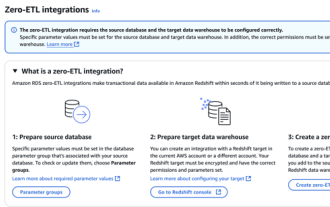
Data Integration Simplification: AmazonRDSMySQL and Redshift's zero ETL integration Efficient data integration is at the heart of a data-driven organization. Traditional ETL (extract, convert, load) processes are complex and time-consuming, especially when integrating databases (such as AmazonRDSMySQL) with data warehouses (such as Redshift). However, AWS provides zero ETL integration solutions that have completely changed this situation, providing a simplified, near-real-time solution for data migration from RDSMySQL to Redshift. This article will dive into RDSMySQL zero ETL integration with Redshift, explaining how it works and the advantages it brings to data engineers and developers.
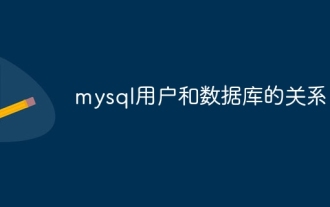
In MySQL database, the relationship between the user and the database is defined by permissions and tables. The user has a username and password to access the database. Permissions are granted through the GRANT command, while the table is created by the CREATE TABLE command. To establish a relationship between a user and a database, you need to create a database, create a user, and then grant permissions.
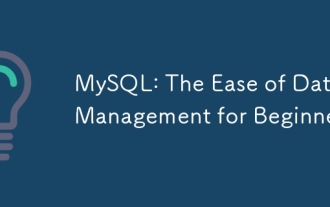
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
