How to Efficiently Select Random Rows from a Large PostgreSQL Table?
Select random rows from large PostgreSQL table
When working with large data sets, selecting random rows can be a computationally intensive task. This article explores various methods for retrieving random rows from a table containing approximately 500 million rows, and discusses their performance and accuracy.
Method 1: Use RANDOM() and LIMIT
The first method involves using the RANDOM() function to generate random numbers and then using the LIMIT clause to filter the results to get the required number of rows.
SELECT * FROM table WHERE RANDOM() < 0.000002 LIMIT 1000;
This approach has the advantage of being easy to implement, but may be inefficient for large tables. Because of the LIMIT clause, the database must scan all rows of the table to pick random rows and discard the rest.
Method 2: Use ORDER BY RANDOM() and LIMIT
Another approach is to first sort the rows by the RANDOM() function and then use the LIMIT clause to get random rows.
SELECT * FROM table ORDER BY RANDOM() LIMIT 1000;
This method is similar to the first method, but the sorting guarantees more efficient selection of random rows. It reduces the number of scans required, making it a better choice for large tables. However, it is still not the best choice for tables with extremely large number of rows.
Efficient approach: use numeric ID columns and indexes
For tables with numeric ID columns and fewer gaps, a more efficient approach can be used. This involves generating random numbers within a range of IDs and using them to join with the table.
WITH params AS ( SELECT 1 AS min_id, -- 最小 ID <= 当前最小 ID 5100000 AS id_span -- 四舍五入。(max_id - min_id + buffer) ) SELECT * FROM ( SELECT p.min_id + trunc(random() * p.id_span)::integer AS id FROM params p, generate_series(1, 1100) g -- 1000 + buffer GROUP BY 1 -- 去除重复项 ) r JOIN table USING (id) LIMIT 1000;
This approach leverages index access to significantly reduce the number of scans required. It is ideal for tables with a large number of rows and few gaps in the ID column.
Considerations and Recommendations
The best way to select random rows depends on specific table characteristics and performance requirements. For small tables, the RANDOM() or ORDER BY RANDOM() methods may be sufficient. However, for large tables with numeric ID columns and few gaps, it is recommended to use the above optimization method for best performance.
It should be noted that due to the nature of pseudo-random number generation in computers, none of these methods can guarantee true randomness. However, they provide a practical way to obtain a random sample of rows from a large table with reasonable efficiency and accuracy.
The above is the detailed content of How to Efficiently Select Random Rows from a Large PostgreSQL Table?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
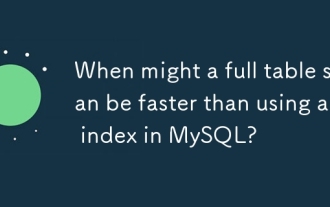
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
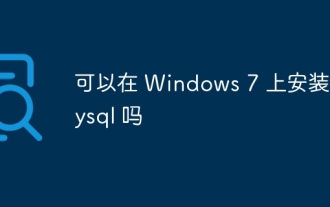
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
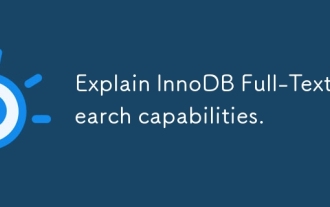
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
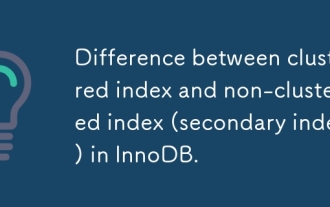
The difference between clustered index and non-clustered index is: 1. Clustered index stores data rows in the index structure, which is suitable for querying by primary key and range. 2. The non-clustered index stores index key values and pointers to data rows, and is suitable for non-primary key column queries.
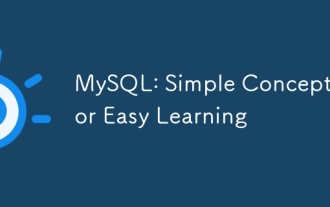
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
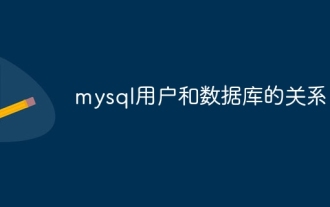
In MySQL database, the relationship between the user and the database is defined by permissions and tables. The user has a username and password to access the database. Permissions are granted through the GRANT command, while the table is created by the CREATE TABLE command. To establish a relationship between a user and a database, you need to create a database, create a user, and then grant permissions.
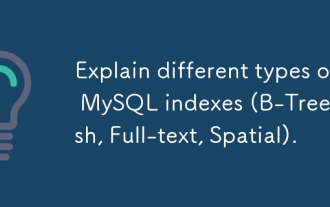
MySQL supports four index types: B-Tree, Hash, Full-text, and Spatial. 1.B-Tree index is suitable for equal value search, range query and sorting. 2. Hash index is suitable for equal value searches, but does not support range query and sorting. 3. Full-text index is used for full-text search and is suitable for processing large amounts of text data. 4. Spatial index is used for geospatial data query and is suitable for GIS applications.
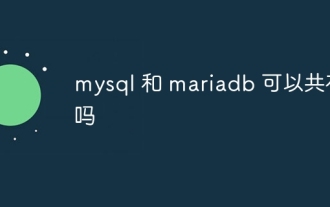
MySQL and MariaDB can coexist, but need to be configured with caution. The key is to allocate different port numbers and data directories to each database, and adjust parameters such as memory allocation and cache size. Connection pooling, application configuration, and version differences also need to be considered and need to be carefully tested and planned to avoid pitfalls. Running two databases simultaneously can cause performance problems in situations where resources are limited.
