


How to Effectively Remove Illegal Characters from File Paths and Filenames?
Clear illegal characters in paths and file names
The task of this programming example is to remove invalid path and file characters from a string without generating errors. Although the given code is intended to solve this problem, it doesn't seem to work. Let’s analyze the missing pieces:
The code attempts to remove illegal characters from a string using the Trim
method. However, Trim
can only remove whitespace characters such as spaces and tabs at the beginning and end of a string. It has no effect on non-whitespace characters.
In order to effectively remove illegal characters, you need to use the specific methods provided by the System.IO
namespace. Here is the modified code that handles this task efficiently:
using System; using System.IO; namespace ConsoleApplication1 { class Program { static void Main(string[] args) { string illegal = " \"M\"\"\a/ry/ h**ad:>> a\/:*?\"| li*tt|le|| la\"mb.?"; // 删除无效的文件字符 illegal = illegal.Replace(Path.GetInvalidFileNameChars(), ""); // 删除无效的路径字符 illegal = illegal.Replace(Path.GetInvalidPathChars(), ""); Console.WriteLine(illegal); Console.ReadLine(); } } }
In this modified code, we replaced the Replace
method with the Trim
method, which allows us to specify which characters to remove from the string. By calling Path.GetInvalidFileNameChars()
and Path.GetInvalidPathChars()
we get arrays of invalid file and path characters respectively. We then pass these arrays as the characters to replace, effectively removing them from the original string.
Here is another solution provided by Ceres in another discussion thread:
public string RemoveInvalidChars(string filename) { return string.Concat(filename.Split(Path.GetInvalidFileNameChars())); }
This code uses the Split
method to split a string at the location of invalid characters and then reassembles the resulting substrings to create a new string that does not contain invalid characters.
You may prefer to replace invalid characters with specific characters, such as underscore:
public string ReplaceInvalidChars(string filename) { return string.Join("_", filename.Split(Path.GetInvalidFileNameChars())); }
This code replaces all invalid characters with underscores, making it suitable for scenarios where you want to preserve the original structure of a path or filename.
These solutions provide powerful and effective ways to eliminate illegal characters in file and path strings, ensuring compatibility and preventing potential file system errors.
The above is the detailed content of How to Effectively Remove Illegal Characters from File Paths and Filenames?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










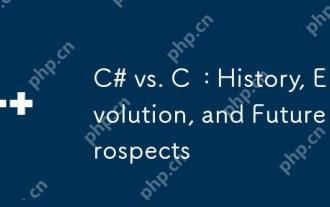
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
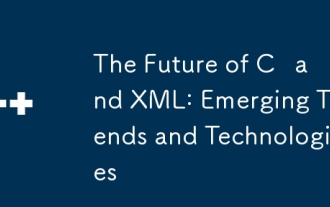
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
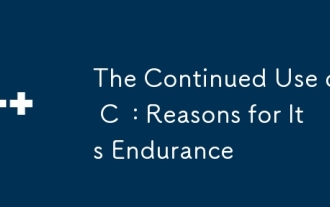
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
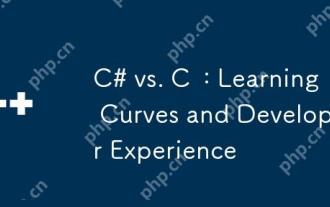
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
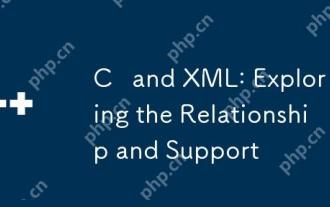
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
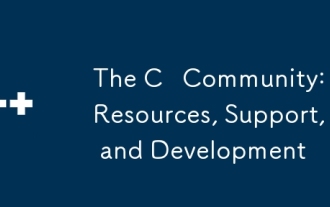
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
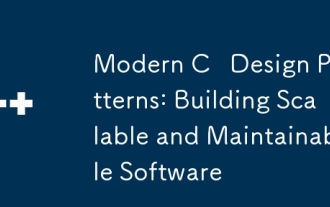
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
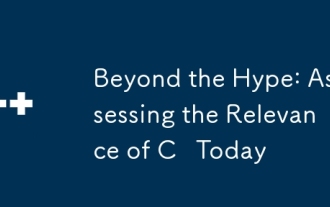
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
