C language from 0
Embark on your C programming journey! While initially daunting, mastering C's fundamentals is achievable with the right approach. This guide provides a structured introduction, progressing from basic concepts to more advanced topics.
Table of Contents
- C Basics and Data Types
- User Input and Output
- Conditional Statements (including shortcuts)
- Switch Statements
- Arrays: One and Two-Dimensional
- Nested Loops
- Functions: Structure and Usage
- Structures (
structs
) - Pointers
C Basics and Data Types
C programs adhere to a standard structure and utilize various data types for variables. A simple example:
#include <stdio.h> int main() { printf("Hello, World!\n"); return 0; }
Key Concepts:
- Data Types:
int
: Integers (e.g.,int x = 10;
)float
anddouble
: Floating-point numbers (decimals) (e.g.,float pi = 3.14;
)char
: Single characters or ASCII codes (e.g.,char letter = 'A';
)bool
: Boolean values (true/false) (requires<stdbool.h>
)
// Data Type Examples: int a = 40; // Integer (4 bytes) short int b = 32767; // Short Integer (2 bytes) unsigned int c = 4294967295; // Unsigned Integer (4 bytes) float d = 9.81; // Float (4 bytes) double e = 3.14159; // Double (8 bytes) bool f = true; // Boolean (1 byte) char g = 'e'; // Character (1 byte) char h = 100; // Character (1 byte) char name[] = "Example"; // String (array of characters) // Variable declaration and initialization int age; // Declaration age = 5; // Initialization char letter = 'C'; // Declaration and initialization // Displaying variables printf("You are %d years old\n", age); // Integer printf("Hello %s\n", name); // String printf("Learning %c\n", letter); // Character // Format specifiers: %d (int), %s (string), %c (char), %f (float), %.2f (float to 2 decimal places)
- Operators:
-
,*
,/
,%
(modulo),--
(decrement). Remember type casting for accurate results (e.g.,float z = 5 / (float)2;
).
User Input and Output
For user input in VS Code, use the Terminal tab.
int age; char name[25]; // Integer Input printf("Enter your age: "); scanf("%d", &age); printf("You are %d years old\n", age); // String Input (using `fgets` for safer input) printf("Enter your name: "); fgets(name, sizeof(name), stdin); // fgets handles spaces name[strcspn(name, "\n")] = 0; // Remove trailing newline from fgets printf("Hello, %s!\n", name);
Case sensitivity matters in C. Use functions like toupper()
from <ctype.h>
for case-insensitive comparisons.
Conditional Shortcuts (Ternary Operator)
The ternary operator provides a concise way to write if-else
statements:
int max = (a > b) ? a : b; // Equivalent to an if-else statement
Switch Statements
Handle multiple conditions efficiently:
char grade = 'A'; switch (grade) { case 'A': printf("Excellent!\n"); break; case 'B': printf("Good!\n"); break; default: printf("Try again!\n"); }
Always include a default
case.
Arrays
Arrays store collections of same-type variables:
int numbers[5] = {10, 20, 30, 40, 50}; printf("%d\n", numbers[0]); // Accesses the first element (10) // 2D Array int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}}; // Array of Strings char cars[][10] = {"BMW", "Tesla", "Toyota"};
Nested Loops
Loops within loops, useful for processing multi-dimensional data: (Example omitted for brevity, but easily constructed using nested for
loops).
Functions
Functions promote code reusability:
void greet(char name[]) { printf("Hello, %s!\n", name); } int main() { greet("Alice"); return 0; }
Structures (structs
)
Group related variables:
struct Player { char name[50]; int score; }; struct Player player1 = {"Bob", 150}; printf("Name: %s, Score: %d\n", player1.name, player1.score);
Pointers
Variables storing memory addresses:
#include <stdio.h> int main() { printf("Hello, World!\n"); return 0; }
Pointers are crucial for dynamic memory allocation. This guide provides a solid foundation. Consistent practice is key to mastering C programming.
The above is the detailed content of C language from 0. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










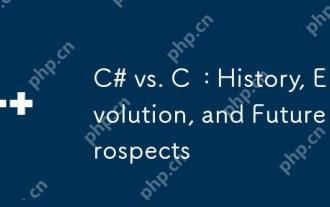
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
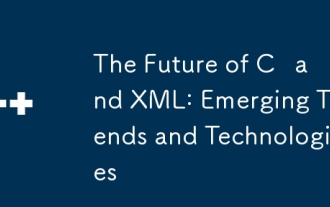
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
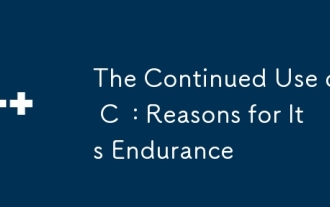
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
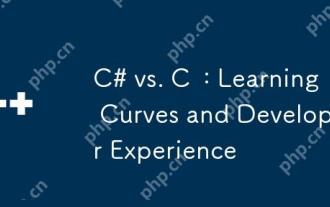
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
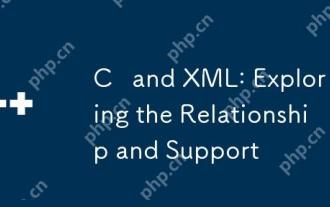
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
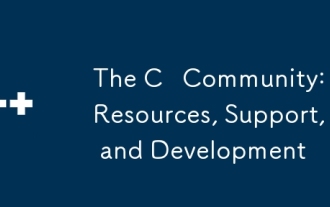
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
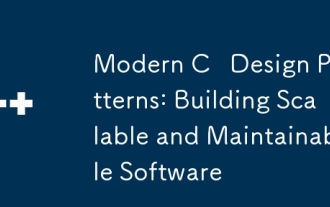
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
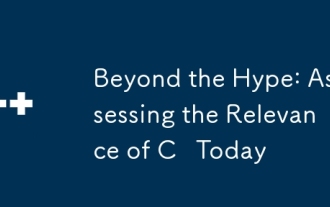
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
